


How to reasonably use the database connection pool to optimize the access performance of Java websites?
How to reasonably use the database connection pool to optimize the access performance of Java websites?
Nowadays, with the rapid development of the Internet, the number of website visits is also increasing. For a Java website, the database is an important part of supporting the normal operation of the website, and the database connection is the key to communication with the database. Therefore, how to reasonably use the database connection pool to optimize access performance has become a problem that every developer should pay attention to and solve. This article will introduce what a database connection pool is and how to rationally use database connection pools in Java websites to optimize access performance.
1. What is a database connection pool?
Database connection pool is a technology for maintaining database connections. It creates a certain number of database connections in advance and puts them into the pool. When the client needs to connect to the database, it obtains the connection from the connection pool and uses it again. Return the connection to the connection pool to reuse the connection. Compared with creating and closing connections each time, using a connection pool can reduce the cost of creating and destroying connections and improve the performance of database access.
2. Benefits of using database connection pool
- Improving response speed: By creating connections in advance, the overhead of establishing and releasing each connection is avoided and the response is reduced. time.
- Reduce resource consumption: Reasonably manage the number of connections in the connection pool to avoid resource waste and system crashes caused by frequent creation and closing of connections.
- Improve concurrency: The connection pool can manage connections and dynamically adjust the number of connections according to needs to meet the needs of multiple concurrent requests.
3. How to reasonably use the database connection pool to optimize access performance
- Import connection pool dependencies
Before using the database connection pool, you need to Add connection pool related dependencies in the project's pom.xml file. Taking the commonly used connection pool DBCP as an example, you can add the following dependencies in pom.xml:
<dependencies> <!-- 连接池依赖 --> <dependency> <groupId>commons-dbcp</groupId> <artifactId>commons-dbcp</artifactId> <version>1.4</version> </dependency> </dependencies>
- Initialization configuration of the connection pool
In the configuration file, you need to set Related parameters of the connection pool, such as the maximum number of connections, the minimum number of connections, the number of idle connections, etc. Adjustments can be made based on specific needs. The following is a simple connection pool configuration example:
import javax.sql.DataSource; import org.apache.commons.dbcp.BasicDataSource; public class ConnectionPool { private static final String DRIVER_CLASS = "com.mysql.jdbc.Driver"; private static final String URL = "jdbc:mysql://localhost:3306/db_name"; private static final String USER = "username"; private static final String PASSWORD = "password"; private static final int MAX_ACTIVE = 100; // 最大活动连接数 private static final int MAX_IDLE = 50; // 最大空闲连接数 private static final int MIN_IDLE = 10; // 最小空闲连接数 private static final int INITIAL_SIZE = 10; // 初始连接数 private static final long MAX_WAIT = 1000; // 最大等待时间 private static DataSource dataSource; static { BasicDataSource ds = new BasicDataSource(); ds.setDriverClassName(DRIVER_CLASS); ds.setUrl(URL); ds.setUsername(USER); ds.setPassword(PASSWORD); ds.setMaxActive(MAX_ACTIVE); ds.setMaxIdle(MAX_IDLE); ds.setMinIdle(MIN_IDLE); ds.setInitialSize(INITIAL_SIZE); ds.setMaxWait(MAX_WAIT); dataSource = ds; } public static DataSource getDataSource() { return dataSource; } }
- Use connection pool for database operations
When using the database connection pool, you need to obtain the connection and execute through the connection pool SQL operations, release connections. The following is a sample code:
import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import javax.sql.DataSource; public class DatabaseUtils { public static void main(String[] args) { DataSource dataSource = ConnectionPool.getDataSource(); Connection connection = null; PreparedStatement statement = null; ResultSet resultSet = null; try { connection = dataSource.getConnection(); String sql = "SELECT * FROM table_name WHERE column = ?"; statement = connection.prepareStatement(sql); statement.setString(1, "value"); resultSet = statement.executeQuery(); // 处理查询结果 while (resultSet.next()) { // ... } } catch (SQLException e) { e.printStackTrace(); } finally { // 释放连接 if (resultSet != null) { try { resultSet.close(); } catch (SQLException e) { e.printStackTrace(); } } if (statement != null) { try { statement.close(); } catch (SQLException e) { e.printStackTrace(); } } if (connection != null) { try { connection.close(); } catch (SQLException e) { e.printStackTrace(); } } } } }
Through the above steps, we can reasonably use the database connection pool in the Java website to optimize access performance.
Summary:
By properly configuring and using the database connection pool, we can improve the access performance of Java websites. The connection pool can reduce the cost of creating and destroying connections and improve response speed; reasonably manage the number of connections in the connection pool and reduce resource consumption; meet the needs of multiple concurrent requests and improve the concurrency capability of the website. Therefore, rational use of database connection pools is an effective way to improve Java website access performance.
The above is the detailed content of How to reasonably use the database connection pool to optimize the access performance of Java websites?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


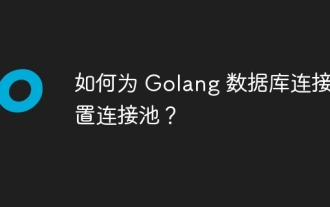
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
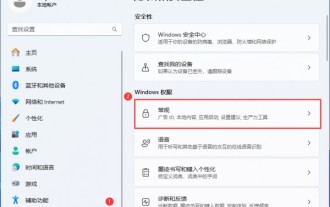
How do we set up and optimize performance after receiving a new computer? Users can directly open Privacy and Security, and then click General (Advertising ID, Local Content, Application Launch, Setting Recommendations, Productivity Tools or directly open Local Group Policy Just use the editor to operate it. Let me introduce to you in detail how to optimize settings and improve performance after receiving a new Win11 computer. How to optimize settings and improve performance after receiving a new Win11 computer. One: 1. Press the [Win+i] key combination to open Settings, then click [Privacy and Security] on the left, and click [General (Advertising ID, Local Content, App Launch, Setting Suggestions, Productivity) under Windows Permissions on the right Tools)】.Method 2
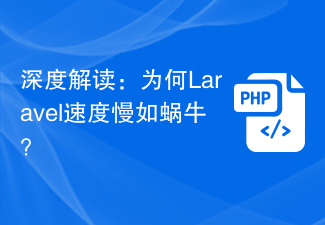
Laravel is a popular PHP development framework, but it is sometimes criticized for being as slow as a snail. What exactly causes Laravel's unsatisfactory speed? This article will provide an in-depth explanation of the reasons why Laravel is as slow as a snail from multiple aspects, and combine it with specific code examples to help readers gain a deeper understanding of this problem. 1. ORM query performance issues In Laravel, ORM (Object Relational Mapping) is a very powerful feature that allows

Decoding Laravel performance bottlenecks: Optimization techniques fully revealed! Laravel, as a popular PHP framework, provides developers with rich functions and a convenient development experience. However, as the size of the project increases and the number of visits increases, we may face the challenge of performance bottlenecks. This article will delve into Laravel performance optimization techniques to help developers discover and solve potential performance problems. 1. Database query optimization using Eloquent delayed loading When using Eloquent to query the database, avoid
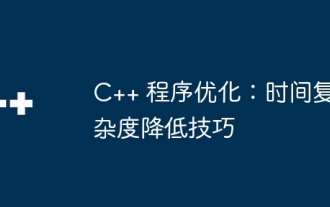
Time complexity measures the execution time of an algorithm relative to the size of the input. Tips for reducing the time complexity of C++ programs include: choosing appropriate containers (such as vector, list) to optimize data storage and management. Utilize efficient algorithms such as quick sort to reduce computation time. Eliminate multiple operations to reduce double counting. Use conditional branches to avoid unnecessary calculations. Optimize linear search by using faster algorithms such as binary search.
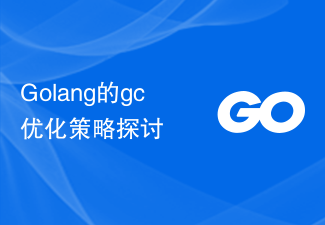
Golang's garbage collection (GC) has always been a hot topic among developers. As a fast programming language, Golang's built-in garbage collector can manage memory very well, but as the size of the program increases, some performance problems sometimes occur. This article will explore Golang’s GC optimization strategies and provide some specific code examples. Garbage collection in Golang Golang's garbage collector is based on concurrent mark-sweep (concurrentmark-s
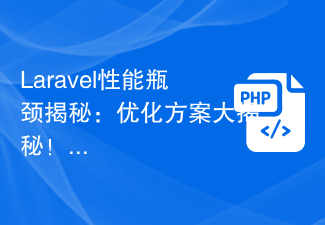
Laravel performance bottleneck revealed: optimization solution revealed! With the development of Internet technology, the performance optimization of websites and applications has become increasingly important. As a popular PHP framework, Laravel may face performance bottlenecks during the development process. This article will explore the performance problems that Laravel applications may encounter, and provide some optimization solutions and specific code examples so that developers can better solve these problems. 1. Database query optimization Database query is one of the common performance bottlenecks in Web applications. exist
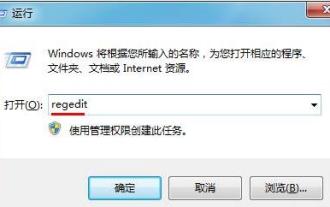
1. Press the key combination (win key + R) on the desktop to open the run window, then enter [regedit] and press Enter to confirm. 2. After opening the Registry Editor, we click to expand [HKEY_CURRENT_USERSoftwareMicrosoftWindowsCurrentVersionExplorer], and then see if there is a Serialize item in the directory. If not, we can right-click Explorer, create a new item, and name it Serialize. 3. Then click Serialize, then right-click the blank space in the right pane, create a new DWORD (32) bit value, and name it Star
