


PHP website access speed optimization: How to reduce page redirections?
PHP website access speed optimization: How to reduce page redirects?
Overview:
When developing and optimizing a PHP website, improving the website's access speed is a key consideration. Page redirects are a common performance issue that cause additional HTTP requests and delays, impacting the user experience. This article will explain how to optimize the access speed of your PHP website by reducing page redirects and provide some code examples.
- Check and fix invalid URL jumps:
Page redirections are usually caused by invalid URL jumps. This may be due to a programming error or configuration issue. Start by checking all URL redirects on your website and making sure they are valid and necessary.
For example, the following code snippet demonstrates how to check whether a URL is valid and perform a redirection:
1 2 3 4 5 |
|
In this example, we use the filter_var() function to verify URL validity. The redirect operation is only performed when the URL is valid.
- Avoid redundant redirects:
Sometimes, page redirects are caused by redundant steps in program logic. By optimizing program logic, we can reduce unnecessary redirects and thereby increase website access speed.
Here is an example that demonstrates how to optimize website access speed by avoiding redundant redirects:
1 2 3 4 5 6 7 8 9 10 11 12 |
|
In this example, we based on the user's login status and permissions Redirect. By optimizing the program logic, we can optimize the above code to:
1 2 3 4 5 6 7 8 9 10 11 12 |
|
By avoiding redundant redirects, we reduce the complexity of the code and improve the access speed of the website.
- Use 301 redirect:
301 redirect refers to a "permanent redirect", which tells search engines and browsers that the URL has been permanently moved to another URL. For URLs that change frequently, using 301 redirects can reduce unnecessary HTTP requests and delays.
The following code demonstrates how to optimize the access speed of the website through 301 redirection:
1 2 3 |
|
In this example, we use the header() function to send the HTTP response header , indicating that the URL has been permanently moved to another URL.
- Use caching to avoid repeated redirections:
For frequently visited pages, we can use caching to avoid repeated redirect requests. By caching URLs that have already been redirected, we can directly return the redirect results in future requests without redirecting again.
Here is an example that demonstrates how to use caching to avoid duplicate redirect requests:
1 2 3 4 5 6 7 8 9 10 11 12 |
|
In this example, we first try to get the redirect URL from the cache. If the URL exists in the cache, the redirect operation is performed directly. If the URL does not exist in the cache, the redirect logic is executed and the result is saved to the cache for future use.
Conclusion:
By reducing page redirections, we can significantly improve the access speed of the PHP website, thus improving the user experience. By checking for and fixing invalid URL redirects, avoiding redundant redirects, using 301 redirects, and using caching to avoid repeated redirect requests, we can optimize your site's performance and deliver faster page load times. When developing and optimizing a PHP website, we should always pay attention to and consider how to reduce page redirections to improve website access speed.
The above is an article about optimizing PHP website access speed. I hope it will be helpful to developers.
The above is the detailed content of PHP website access speed optimization: How to reduce page redirections?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


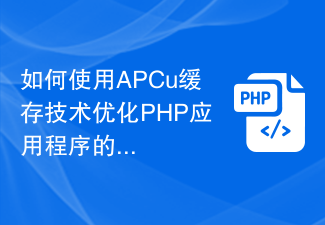
At present, PHP has become one of the most popular programming languages in Internet development, and the performance optimization of PHP programs has also become one of the most pressing issues. When handling large-scale concurrent requests, a delay of one second can have a huge impact on the user experience. Today, APCu (AlternativePHPCache) caching technology has become one of the important methods to optimize PHP application performance. This article will introduce how to use APCu caching technology to optimize the performance of PHP applications. 1. APC
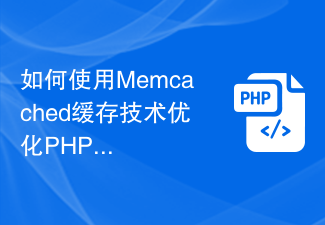
With the development of the Internet, PHP applications have become more and more common in the field of Internet applications. However, high concurrent access by PHP applications can lead to high CPU usage on the server, thus affecting the performance of the application. In order to optimize the performance of PHP applications, Memcached caching technology has become a good choice. This article will introduce how to use Memcached caching technology to optimize the CPU usage of PHP applications. Introduction to Memcached caching technology Memcached is a
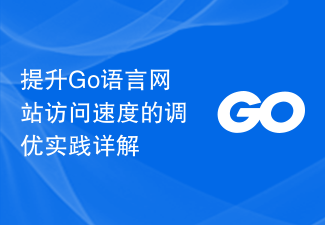
Detailed explanation of tuning practices to improve Go language website access speed Abstract: In the rapidly developing Internet era, website access speed has become one of the important factors for users to choose a website. This article will introduce in detail how to use Go language to optimize website access speed, including practical experience in optimizing network requests, using cache, and concurrent processing. The article will also provide code examples to help readers better understand and apply these optimization techniques. 1. Optimize network requests In website development, network requests are an inevitable link. And optimizing network requests can
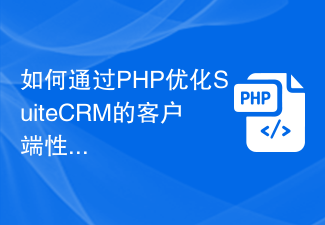
Overview of How to Optimize SuiteCRM's Client-Side Performance with PHP: SuiteCRM is a powerful open source customer relationship management (CRM) system, but performance issues can arise when handling large amounts of data and concurrent users. This article will introduce some methods to optimize SuiteCRM client performance through PHP programming techniques, and attach corresponding code examples. Using appropriate data queries and indexes Database queries are one of the core operations of a CRM system. In order to improve query performance, appropriate data query
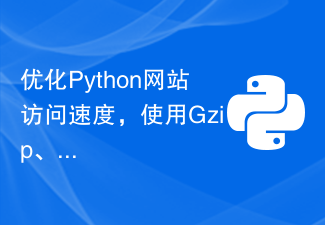
Optimize Python website access speed and use compression algorithms such as Gzip and Deflate to reduce data transmission. With the development of the Internet, website access speed has become one of the important indicators of user experience. When developing Python websites, we often face a problem, that is, how to reduce the amount of data transferred, thereby increasing the access speed of the website. This article will introduce how to use compression algorithms such as Gzip and Deflate to optimize the access speed of Python websites. In Python we can use the following
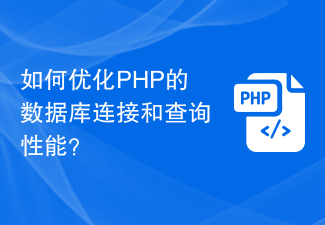
How to optimize PHP's database connection and query performance? The database is an indispensable part of web development, and PHP, as a widely used server-side scripting language, its connection to the database and query performance are crucial to the performance of the entire system. This article will introduce some tips and suggestions for optimizing PHP database connection and query performance. Use persistent connections: In PHP, a database connection is established every time a database query is executed. Persistent connections can reuse the same database connection in multiple queries, thereby reducing
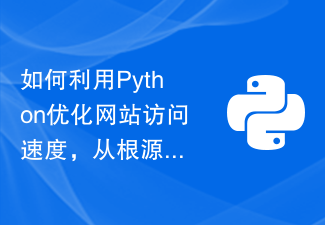
How to use Python to optimize website access speed and solve performance bottlenecks from the root cause? Abstract: With the rapid development of the Internet, website access speed has become one of the important indicators of user experience. This article will introduce how to use Python to optimize website access speed and solve performance bottlenecks from the root cause. Specifically, it includes the use of concurrent requests, caching technology, the use of asynchronous programming, and the use of performance monitoring tools. 1. Use concurrent requests. In traditional serial requests, each request blocks the thread, resulting in longer response time. And using concurrent requests can be done in
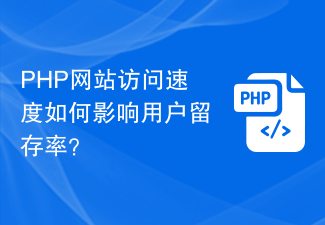
How does PHP website access speed affect user retention rate? With the rapid development of the Internet, users have higher and higher requirements for website experience. Among the many factors that affect user experience, website access speed is undoubtedly one of the most important. As a commonly used web development language, PHP needs to pay attention to its performance and speed while ensuring complete functions. This article will explore the impact of PHP website access speed on user retention rate and propose some optimization methods. First, let’s understand what user retention is. Simply put, users stay
