


Add, delete, modify and query operations of XML data in Python
Add, delete, modify and query operations of XML data in Python
XML (Extensible Markup Language) is a text format used to store and transmit data. In Python, we can use a variety of libraries to process XML data, the most commonly used of which is the xml.etree.ElementTree
library. This article will introduce how to use Python to add, delete, modify and query XML data, and illustrate it through code examples.
1. Introduction of modules
First, we need to introduce the xml.etree.ElementTree library to process XML data. You can use the following code to introduce it:
import xml.etree.ElementTree as ET
2. Parse XML files
Before operating on XML data, we first need to parse the XML file into an Element object. You can use the following code to parse XML files:
tree = ET.parse('data.xml') root = tree.getroot()
The above code will parse the data.xml file into an ElementTree object tree, and obtain the root element object root from the tree.
3. Search for elements
In XML, there can be hierarchical relationships between elements. We can find a specific element by its tag name and attributes. You can use the following code to find elements:
Find elements by tag name:
element = root.find('element_name')
Copy after loginFind elements by attributes:
element = root.find(".//element_name[@attribute='value']")
Copy after login
4. Traverse elements
In XML data, there may be multiple elements with the same tag name. We can get all these elements by traversing. You can use the following code to traverse elements:
for element in root.iter('element_name'): # 执行相关操作
The above code will traverse all child elements named element_name under the root element root and perform related operations on each element.
5. New elements
We can use the Element
method of the Element object to create a new element, and use the append
method to add it to the specified under the parent element. You can use the following code to add new elements:
new_element = ET.Element('new_element') parent_element.append(new_element)
The above code will create an element named new_element and add it to the parent element parent_element.
6. Modify the element
You can use the set
method of the Element object to modify the attribute value of the element. You can use the following code to modify the attribute value of an element:
element.set('attribute', 'new_value')
The above code will change the attribute value of the element to new_value.
7. Delete elements
You can use the remove
method of the Element object to delete the specified element. You can use the following code to delete elements:
parent_element.remove(element)
The above code will delete the specified element element from the parent_element parent element.
To sum up, we introduced how to use Python to add, delete, modify and query XML data and explained it through code examples. By mastering these basic operations, we can process XML data more conveniently and implement our own business logic.
The above is the detailed content of Add, delete, modify and query operations of XML data in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
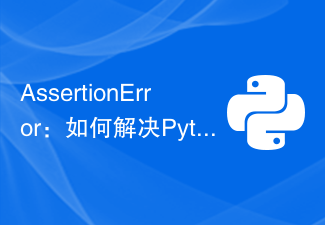
Assertions in Python are a useful tool for programmers to debug their code. It is used to verify that the internal state of the program meets expectations and raise an assertion error (AssertionError) when these conditions are false. During the development process, assertions are used during testing and debugging to check whether the status of the code matches the expected results. This article will discuss the causes, solutions, and how to correctly use assertions in your code. Cause of assertion error Assertion error pass
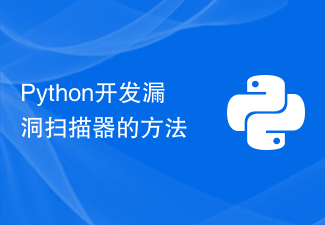
Overview of how to develop a vulnerability scanner through Python In today's environment of increasing Internet security threats, vulnerability scanners have become an important tool for protecting network security. Python is a popular programming language that is concise, easy to read and powerful, suitable for developing various practical tools. This article will introduce how to use Python to develop a vulnerability scanner to provide real-time protection for your network. Step 1: Determine Scan Targets Before developing a vulnerability scanner, you need to determine what targets you want to scan. This can be your own network or anything you have permission to test
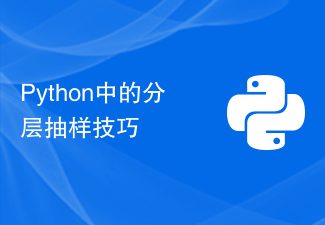
Stratified Sampling Technique in Python Sampling is a commonly used data collection method in statistics. It can select a portion of samples from the data set for analysis to infer the characteristics of the entire data set. In the era of big data, the amount of data is huge, and using full samples for analysis is both time-consuming and not economically practical. Therefore, choosing an appropriate sampling method can improve the efficiency of data analysis. This article mainly introduces stratified sampling techniques in Python. What is stratified sampling? In sampling, stratified sampling
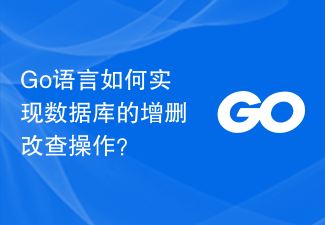
Go language is an efficient, concise and easy-to-learn programming language. It is favored by developers because of its advantages in concurrent programming and network programming. In actual development, database operations are an indispensable part. This article will introduce how to use Go language to implement database addition, deletion, modification and query operations. In Go language, we usually use third-party libraries to operate databases, such as commonly used sql packages, gorm, etc. Here we take the sql package as an example to introduce how to implement the addition, deletion, modification and query operations of the database. Assume we are using a MySQL database.
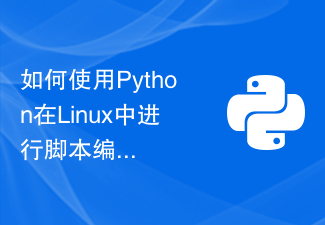
How to use Python to write and execute scripts in Linux In the Linux operating system, we can use Python to write and execute various scripts. Python is a concise and powerful programming language that provides a wealth of libraries and tools to make scripting easier and more efficient. Below we will introduce the basic steps of how to use Python for script writing and execution in Linux, and provide some specific code examples to help you better understand and use it. Install Python
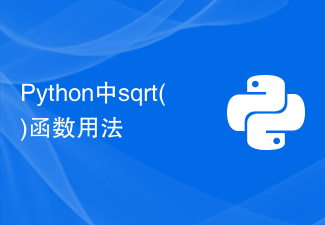
Usage and code examples of the sqrt() function in Python 1. Function and introduction of the sqrt() function In Python programming, the sqrt() function is a function in the math module, and its function is to calculate the square root of a number. The square root means that a number multiplied by itself equals the square of the number, that is, x*x=n, then x is the square root of n. The sqrt() function can be used in the program to calculate the square root. 2. How to use the sqrt() function in Python, sq
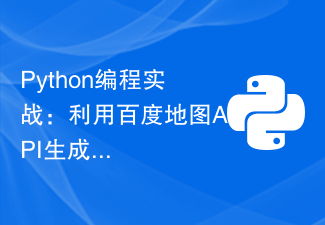
Python programming practice: How to use Baidu Map API to generate static map functions Introduction: In modern society, maps have become an indispensable part of people's lives. When working with maps, we often need to obtain a static map of a specific area for display on a web page, mobile app, or report. This article will introduce how to use the Python programming language and Baidu Map API to generate static maps, and provide relevant code examples. 1. Preparation work To realize the function of generating static maps using Baidu Map API, I
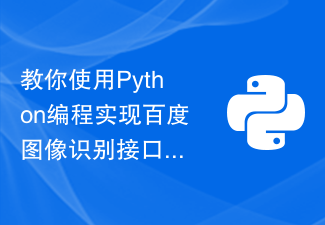
Teach you to use Python programming to implement the docking of Baidu's image recognition interface and realize the image recognition function. In the field of computer vision, image recognition technology is a very important technology. Baidu provides a powerful image recognition interface through which we can easily implement image classification, labeling, face recognition and other functions. This article will teach you how to use the Python programming language to realize the image recognition function by connecting to the Baidu image recognition interface. First, we need to create an application on Baidu Developer Platform and obtain
