


How to handle large data volume calculations in Java back-end function development?
How to handle large data calculations in Java back-end function development?
With the rapid development of the Internet and technology, the amount of data in various applications is also increasing. In the development of Java back-end functions, processing calculations with large amounts of data is a common challenge. This article will introduce some effective methods for handling large data volume calculations and provide some code examples.
1. Use the distributed computing framework
The distributed computing framework can decompose the computing tasks of large amounts of data into multiple small tasks for parallel computing, thereby improving computing efficiency. Hadoop is a commonly used distributed computing framework that can divide a data set into multiple chunks and perform parallel calculations on multiple machines. The following is a sample code that uses Hadoop for large data volume calculations:
public class WordCount { public static class Map extends Mapper<LongWritable, Text, Text, IntWritable> { private final static IntWritable one = new IntWritable(1); private Text word = new Text(); public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { String line = value.toString(); StringTokenizer tokenizer = new StringTokenizer(line); while (tokenizer.hasMoreTokens()) { word.set(tokenizer.nextToken()); context.write(word, one); } } } public static class Reduce extends Reducer<Text, IntWritable, Text, IntWritable> { private IntWritable result = new IntWritable(); public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int sum = 0; for (IntWritable val : values) { sum += val.get(); } result.set(sum); context.write(key, result); } } public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); Job job = Job.getInstance(conf, "word count"); job.setJarByClass(WordCount.class); job.setMapperClass(Map.class); job.setCombinerClass(Reduce.class); job.setReducerClass(Reduce.class); job.setOutputKeyClass(Text.class); job.setOutputValueClass(IntWritable.class); FileInputFormat.addInputPath(job, new Path(args[0])); FileOutputFormat.setOutputPath(job, new Path(args[1])); System.exit(job.waitForCompletion(true) ? 0 : 1); } }
The above code is a simple word counting program that uses Hadoop for distributed calculations. By splitting the data set into chunks and running parallel tasks on multiple machines, calculations can be greatly sped up.
2. Use multi-threaded processing
In addition to using the distributed computing framework, you can also use multi-threading to process large amounts of data calculations. Java's multi-threading mechanism can perform multiple tasks at the same time, thereby improving computing efficiency. The following is a sample code that uses multi-threading to process large data calculations:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class BigDataProcessing { public static void main(String[] args) { int numberOfThreads = 10; // 设置线程数量 ExecutorService executor = Executors.newFixedThreadPool(numberOfThreads); // 待处理的数据集 List<Integer> data = new ArrayList<>(); for (int i = 0; i < 1000000; i++) { data.add(i); } // 创建任务,并提交给线程池 for (int i = 0; i < numberOfThreads; i++) { int startIndex = i * (data.size() / numberOfThreads); int endIndex = (i + 1) * (data.size() / numberOfThreads); Runnable task = new DataProcessingTask(data.subList(startIndex, endIndex)); executor.submit(task); } executor.shutdown(); } public static class DataProcessingTask implements Runnable { private List<Integer> dataChunk; public DataProcessingTask(List<Integer> dataChunk) { this.dataChunk = dataChunk; } public void run() { // 处理数据的逻辑 for (Integer data : dataChunk) { // 进行具体的计算操作 // ... } } } }
The above code uses Java's multi-threading mechanism to divide the large data set into several small pieces and assign them to multiple threads for processing. parallel computing. By reasonably adjusting the number of threads, CPU resources can be fully utilized and computing efficiency improved.
Summary:
The calculation of processing large amounts of data is an important issue in the development of Java back-end functions. This article introduces two effective methods for processing large data volume calculations, namely using a distributed computing framework and using multi-threaded processing. By rationally selecting applicable methods and combining them with actual needs, calculation efficiency can be improved and efficient data processing can be achieved.
The above is the detailed content of How to handle large data volume calculations in Java back-end function development?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
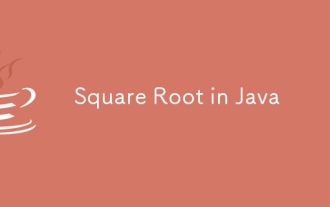
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
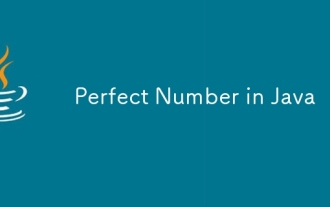
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
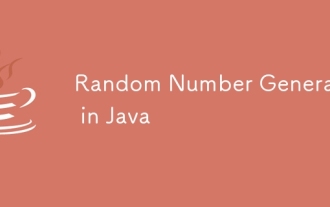
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
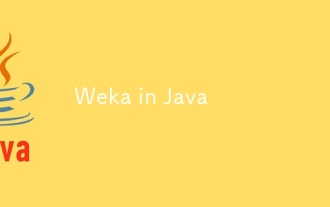
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
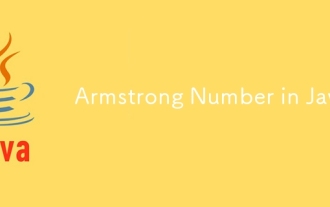
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
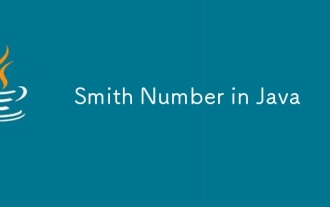
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
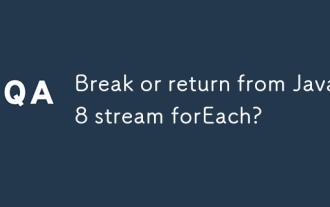
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
