


Detailed explanation of the page data synchronization and update function of Python to implement headless browser collection application
Detailed explanation of the page data synchronization and update function of Python to implement headless browser collection applications
With the rapid development of the Internet, more and more applications require and Web pages for data interaction. When implementing such a function, a common way is to use a headless browser to simulate user operations in order to obtain data on the web page. This article will introduce in detail how to use Python and a headless browser to implement the application's page data synchronization and update functions, and provide corresponding code examples.
- Environment preparation
First, we need to install Python related libraries, including selenium and webdriver_manager. You can use the pip command to install these libraries:
pip install selenium pip install webdriver_manager
In addition, we also need to download the headless browser driver corresponding to the operating system, such as the Chrome browser driver, which can be found at https://sites.google.com Download from /a/chromium.org/chromedriver/.
- Initialize the headless browser
Next, we need to use the headless browser to open the web page and obtain the corresponding data. In Python, we can use the selenium library to achieve this function.
from selenium import webdriver from selenium.webdriver.chrome.options import Options from webdriver_manager.chrome import ChromeDriverManager # 设置无头浏览器的配置 chrome_options = Options() chrome_options.add_argument("--headless") # 打开无头模式 # 初始化无头浏览器 driver = webdriver.Chrome(ChromeDriverManager().install(), options=chrome_options) # 打开网页 driver.get("https://www.example.com")
Through the above code, we successfully initialized a headless browser and opened the "https://www.example.com" web page. The address of the web page can be modified according to actual needs.
- Get page data
Once the page is opened successfully, we can use the headless browser method to obtain the data on the page. For example, we can get all the links and print them out.
# 获取页面上的所有链接 links = driver.find_elements_by_tag_name("a") # 打印链接 for link in links: print(link.get_attribute("href"))
Through the above code, we successfully obtained the href attributes of all links on the page and printed them out.
- Page data synchronization and update
In practical applications, we may need to regularly update the data on the page. To this end, we can encapsulate the above functions into a function and use a timer to call this function regularly.
import time # 定义获取页面数据的函数 def get_page_data(): # 打开网页 driver.get("https://www.example.com") # 获取页面上的所有链接 links = driver.find_elements_by_tag_name("a") # 打印链接 for link in links: print(link.get_attribute("href")) # 定义定时器,每隔5秒钟调用一次get_page_data函数 while True: get_page_data() time.sleep(5) # 休眠5秒钟
Through the above code, we successfully implemented the synchronization and update functions of page data. The headless browser will regularly open the web page and obtain the data, and then we can process it accordingly according to the needs.
Summary:
This article details how to use Python and a headless browser to implement the page data synchronization and update functions of the application. We first installed the relevant libraries and drivers and initialized the headless browser. Then, we used the headless browser method to obtain the data on the page and demonstrated how to update the page data regularly. I hope the content of this article will be helpful to readers and can be used in practical applications.
Code example:
from selenium import webdriver from selenium.webdriver.chrome.options import Options from webdriver_manager.chrome import ChromeDriverManager import time # 设置无头浏览器的配置 chrome_options = Options() chrome_options.add_argument("--headless") # 打开无头模式 # 初始化无头浏览器 driver = webdriver.Chrome(ChromeDriverManager().install(), options=chrome_options) # 定义获取页面数据的函数 def get_page_data(): # 打开网页 driver.get("https://www.example.com") # 获取页面上的所有链接 links = driver.find_elements_by_tag_name("a") # 打印链接 for link in links: print(link.get_attribute("href")) # 定义定时器,每隔5秒钟调用一次get_page_data函数 while True: get_page_data() time.sleep(5) # 休眠5秒钟
The above is the detailed content of Detailed explanation of the page data synchronization and update function of Python to implement headless browser collection application. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


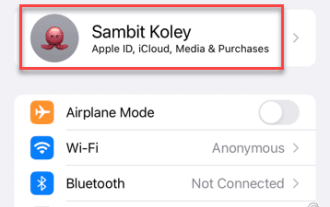
Does your iPhone show "iCloud Storage Full" whenever you download some files or airdrop something? The free plan of iCloud storage is limited to only 5GB. Therefore, the first thing you should check is the current iCloud storage situation on your phone. If there is still enough storage space and you receive a notification, these solutions will help you troubleshoot. Fix 1 – Delete iCloud Backup Remove the existing version of iCloud backup from your phone settings. Step 1 – Open Settings. Step 2 – You will find your Apple ID at the top of the Settings panel. Click on it to open it. Step 3 – Turn on “iCloud” to open iCloud settings. Step 4 – Down
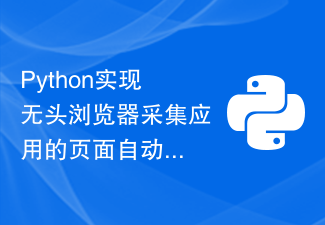
Python implements automatic page refresh and scheduled task function analysis for headless browser collection applications. With the rapid development of the network and the popularization of applications, the collection of web page data has become more and more important. The headless browser is one of the effective tools for collecting web page data. This article will introduce how to use Python to implement the automatic page refresh and scheduled task functions of a headless browser. The headless browser adopts a browser operation mode without a graphical interface, which can simulate human operation behavior in an automated way, thereby enabling the user to access web pages, click buttons, and fill in information.
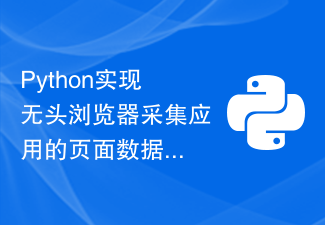
Analysis of page data caching and incremental update functions for headless browser collection applications implemented in Python Introduction: With the continuous popularity of network applications, many data collection tasks require crawling and parsing web pages. The headless browser can fully operate the web page by simulating the behavior of the browser, making the collection of page data simple and efficient. This article will introduce the specific implementation method of using Python to implement the page data caching and incremental update functions of a headless browser collection application, and attach detailed code examples. 1. Basic principles: headless
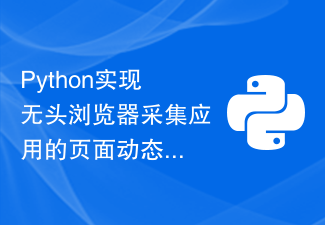
Python implements the dynamic loading and asynchronous request processing functions of headless browser collection applications. In web crawlers, sometimes it is necessary to collect page content that uses dynamic loading or asynchronous requests. Traditional crawler tools have certain limitations in processing such pages, and cannot accurately obtain the content generated by JavaScript on the page. Using a headless browser can solve this problem. This article will introduce how to use Python to implement a headless browser to collect page content using dynamic loading and asynchronous requests.
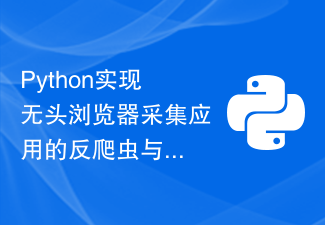
Python implements anti-crawler and anti-detection function analysis and response strategies for headless browser collection applications. With the rapid growth of network data, crawler technology plays an important role in data collection, information analysis and business development. However, the accompanying anti-crawler technology is also constantly upgrading, which brings challenges to the development and maintenance of crawler applications. To deal with anti-crawler restrictions and detection, headless browsers have become a common solution. This article will introduce the analysis and analysis of Python's anti-crawler and anti-detection functions for headless browser collection applications.
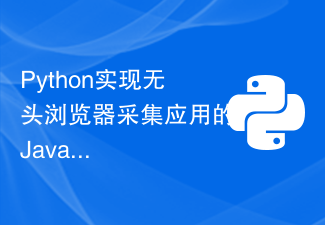
Title: Python implements JavaScript rendering and dynamic page loading functions for headless browser acquisition applications Analysis text: With the popularity of modern web applications, more and more websites use JavaScript to implement dynamic loading of content and data rendering. This is a challenge for crawlers because traditional crawlers cannot parse JavaScript. To handle this situation, we can use a headless browser to parse JavaScript and get dynamically by simulating real browser behavior
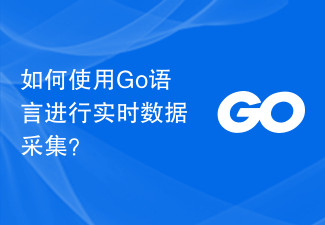
With the continuous development of Internet of Things technology, real-time data collection has become an indispensable part of the digital era. Among various programming languages, Go language has become an ideal choice for real-time data collection with its efficient concurrency performance and concise syntax. This article will introduce how to use Go language for real-time data collection. 1. Selection of data collection framework Before using Go language for real-time data collection, we need to choose a data collection framework that suits us. The more popular data collection frameworks currently on the market include

Detailed explanation of page content parsing and structuring functions for headless browser collection applications implemented in Python Introduction: In today's era of information explosion, the amount of data on the Internet is huge and messy. Nowadays, many applications need to collect data from the Internet, but traditional web crawler technology often needs to simulate browser behavior to obtain the required data, and this method is not feasible in many cases. Therefore, headless browsers become a great solution. This article will introduce in detail how to use Python to implement headless browser collection of application pages.
