Building a real-time translation tool based on JavaScript
Building a real-time translation tool based on JavaScript
Introduction
With the growing demand for globalization and the frequent occurrence of transnational exchanges and exchanges, real-time translation Tools have become a very important application. We can leverage JavaScript and some existing APIs to build a simple but useful real-time translation tool. This article will introduce how to implement this function based on JavaScript, with code examples.
Implementation Steps
Step 1: Create HTML Structure
First, we need to create a simple HTML structure to house our real-time translation tool. Here is a sample HTML structure:
1 2 3 4 5 6 7 8 9 10 11 12 |
|
Step 2: Add styles
To beautify our real-time translation tool, we can add some basic CSS styles. The following is an example style:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
|
Step 3: Implement the translation function
Use JavaScript and Google Translate API to implement real-time translation function. First, add the following code at the bottom of the page:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
|
In the above code, we use Google Translate’s API for translation. We first loaded Google's JavaScript library, then initialized the translation tool and added an input event listener to the source text box. Whenever the user enters content, this event will be triggered and the translation function will be called to obtain the translation results.
Conclusion
With the above simple steps, we can build a real-time translation tool based on JavaScript. Users can enter text to be translated and it will be automatically translated into English or other target languages via the Google Translate API. This real-time translation tool can be easily applied to cross-language communication and communication.
Please note that the Google Translate API is used in the code examples, and corresponding subscription and authentication may be required in actual use. At the same time, in order to improve user experience, more features and optimizations can be added. However, the code examples above can serve as a basis for you to start building a real-time translation tool.
Reference
- Google Translate API Documentation - https://cloud.google.com/translate/docs/reference/
The above is the detailed content of Building a real-time translation tool based on JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


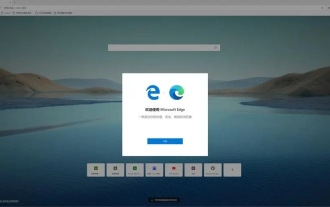
The edge browser comes with a translation function that allows users to translate anytime and anywhere, which brings great convenience to users. However, many users say that the built-in translation webpage is missing. Then the edge browser automatically What should I do if the translation page I brought is missing? Let this site introduce how to restore the translated web page that comes with the Edge browser if it is missing. How to restore the translation webpage that comes with the Edge browser is missing 1. Check whether the translation function is enabled: In the Edge browser, click the three dots icon in the upper right corner, and then select the "Settings" option. On the left side of the settings page, select the Language option. Make sure "Translate&rd"
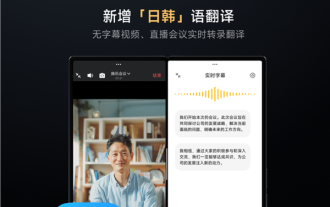
According to news on July 22, today, the official Weibo of Xiaomi ThePaper OS announced that Xiaoai Translation has been upgraded. Real-time subtitles have been added to Japanese and Korean translations, and subtitle-free videos and live conferences can be transcribed and translated in real time. Face-to-face simultaneous interpretation supports translation into 12 languages, including Chinese, English, Japanese, Korean, Russian, Portuguese, Spanish, Italian, French, German, Indonesian, and Hindi. The above functions currently only support the following three new phones: Xiaomi MIX Fold 4 Xiaomi MIX Flip Redmi K70 Extreme Edition It is reported that in 2021, Xiao Ai’s AI subtitles will be added to Japanese and Korean translations. AI subtitles use Xiaomi’s self-developed simultaneous interpretation technology to provide a faster, more stable and accurate subtitle reading experience. 1. According to the official statement, Xiaoai Translator can not only be used in audio and video venues
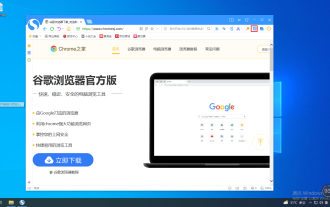
How does Sogou browser translate? When we usually use Sogou browser to check information, we will encounter some websites that are all in English. Because we can’t understand English, it is very difficult to browse the website. This is also very inconvenient. It doesn’t matter if you encounter this situation! Sogou Browser has a built-in translation button. With just one click, Sogou Browser will automatically translate the entire webpage for you? If you don’t know how to operate it, the editor has compiled the specific steps on how to translate it on Sogou Browser. If you don’t know how, follow me and read on! How to translate Sogou Browser 1. Open Sogou Browser, click the translation icon in the upper right corner 2. Select the type of translation text, and then enter the text that needs to be translated 3. Sogou Browser will automatically translate the text. At this point, the above Sogou Browsing operation is completed. How to translate all contents
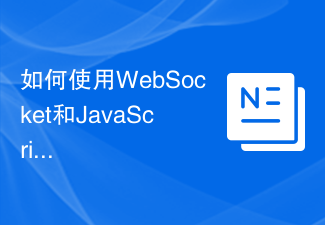
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
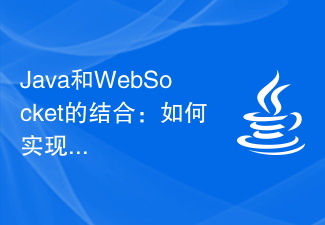
With the continuous development of Internet technology, real-time video streaming has become an important application in the Internet field. To achieve real-time video streaming, the key technologies include WebSocket and Java. This article will introduce how to use WebSocket and Java to implement real-time video streaming playback, and provide relevant code examples. 1. What is WebSocket? WebSocket is a protocol for full-duplex communication on a single TCP connection. It is used on the Web
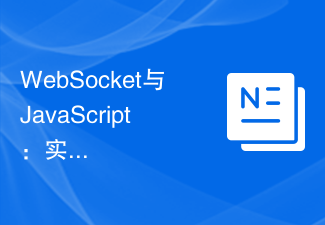
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
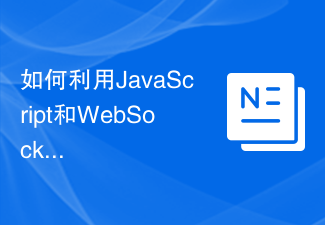
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
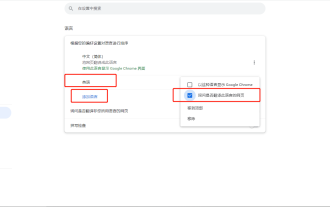
Browsers generally have built-in translation functions, so you don’t have to worry about not being able to understand when browsing foreign language websites! Google Chrome is no exception, but some users find that when they open the translation function of Google Chrome, there is no response or failure. What should they do? You can try the latest solution I found. Operation tutorial: Click the three dots in the upper right corner and click Settings. Click Add Language, add English and Chinese, and make the following settings for them. The English setting asks whether to translate web pages in this language. The Chinese setting displays web pages in this language, and Chinese must be moved to the top before it can be set as the default language. If you open the webpage and no translation option pops up, right-click and select Translate Chinese, OK.
