Implement RSS subscription function using PHP and XML
Use PHP and XML to implement RSS subscription function
RSS (Really Simple Syndication) is a standard format for publishing and subscribing to website updates. It is based on XML and gets the latest content through the subscriber's RSS reader. In this article, we will introduce how to use PHP and XML to implement a simple RSS subscription function.
- Create XML file
First, we need to create an XML file to store the content we want to publish. Assume that what we want to publish is the article title and link. The structure of the XML file is as follows:
<?xml version="1.0" encoding="utf-8"?> <rss version="2.0"> <channel> <title>我的网站</title> <link>https://www.example.com</link> <description>这是我的网站</description> <item> <title>文章标题1</title> <link>https://www.example.com/article1</link> </item> <item> <title>文章标题2</title> <link>https://www.example.com/article2</link> </item> </channel> </rss>
Save the above content as the "rss.xml" file.
- PHP reads XML and outputs the content
Next, we use PHP to read the XML file and output the content. This allows the latest content to be displayed dynamically to subscribers. The following is a simple PHP code example:
<?php $xml = simplexml_load_file("rss.xml"); echo "<h1 id="xml-channel-title">" . $xml->channel->title . "</h1>"; echo "<p>" . $xml->channel->description . "</p>"; foreach ($xml->channel->item as $item) { echo "<h2 id="item-title">" . $item->title . "</h2>"; echo "<p><a href='" . $item->link . "'>阅读全文</a></p>"; } ?>
In the above code, we use the simplexml_load_file function to load the XML file, and use the object operator "->" to access the content of the XML node. First, we output the title and description of the channel, then use a loop to output the title and link of each article.
- Subscribers can use RSS readers to get the latest content
Subscribers can use various RSS readers to get the latest content. They simply provide a link to the XML file to subscribe to the website's content. For example, assuming the link to our XML file is "https://www.example.com/rss.xml", subscribers can add that link to their favorite RSS reader.
- Update the content of the XML file
When publishing a new article, we need to update the content of the XML file. We can add new articles to XML files through PHP. The following is a simple PHP code example:
<?php $xml = simplexml_load_file("rss.xml"); $newItem = $xml->channel->addChild("item"); $newItem->addChild("title", "新文章标题"); $newItem->addChild("link", "https://www.example.com/new-article"); $xml->asXML("rss.xml"); ?>
In the above code, we first load the XML file, then use the addChild function to add a new item node and set its title and link. Finally, we use the asXML function to save the updated XML to a file.
Through the above steps, we can use PHP and XML to implement a simple RSS subscription function. Subscribers can use an RSS reader to get the latest content and have it dynamically displayed to them as it is updated. At the same time, publishers can dynamically update the content of XML files through PHP in order to provide the latest articles to subscribers. In this way, we can easily publish and subscribe to the updated content of the website.
The above is the detailed content of Implement RSS subscription function using PHP and XML. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
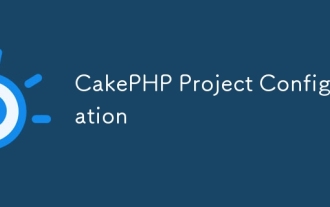
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
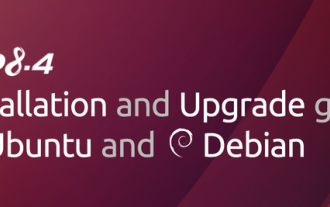
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
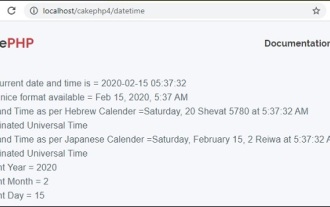
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
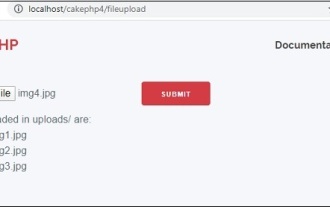
To work on file upload we are going to use the form helper. Here, is an example for file upload.
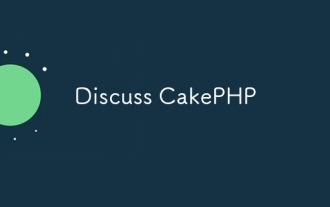
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
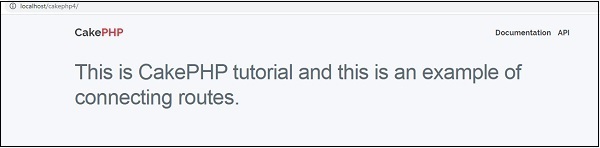
In this chapter, we are going to learn the following topics related to routing ?
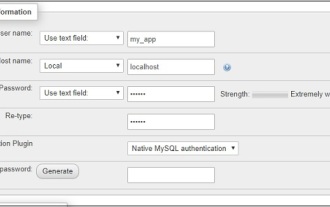
Working with database in CakePHP is very easy. We will understand the CRUD (Create, Read, Update, Delete) operations in this chapter.
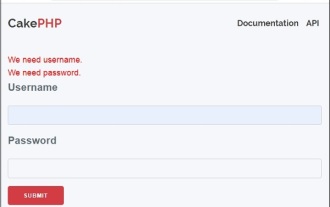
Validator can be created by adding the following two lines in the controller.
