


Continuous integration and continuous deployment practices for PHP data caching
Continuous integration and continuous deployment practice of PHP data caching
Introduction:
In the modern software development process, continuous integration and continuous deployment have become very important. These concepts can make development teams more efficient, reduce errors, and accelerate the delivery of software products. As a commonly used programming language, PHP can also optimize the development process through continuous integration and continuous deployment. This article will introduce how to implement continuous integration and continuous deployment of data caching in PHP projects.
- Appropriate selection of caching mechanism
In PHP projects, data caching is an important means to improve performance. However, choosing the right caching mechanism is crucial. Currently, common PHP caching mechanisms include Memcached, Redis and APC. Based on factors such as project requirements and budget, it is necessary to choose a suitable caching mechanism. - Writing caching code
In a PHP project, data caching can be achieved by calling the API provided by the caching mechanism. For example, in Memcached, you can use theset()
function to store data into the cache and theget()
function to get data from the cache. The following is a simple sample code that shows how to use Memcached for caching operations:
$memcached = new Memcached(); $memcached->addServer("localhost", 11211); $key = "user_123"; $data = $memcached->get($key); if ($data === false) { // 缓存中不存在数据,从数据库中获取并存入缓存 $data = fetchDataFromDatabase(); $memcached->set($key, $data, 3600); // 数据有效期为1小时 } // 使用$data进行后续逻辑处理
- Writing cache integration tests
Continuous integration refers to automatically running a test before each code submission A series of tests to ensure the quality and functionality of the code. In PHP projects, you can write cache integration tests to ensure that the cache is working properly. The following is a simple cache integration test example:
public function testCacheIntegration() { $memcached = new Memcached(); $memcached->addServer("localhost", 11211); $key = "user_123"; $data = $memcached->get($key); // 断言缓存中的数据和从数据库获取的数据一致 $expectedData = fetchDataFromDatabase(); $this->assertEquals($expectedData, $data); }
- Automated deployment of cache configuration
Continuous deployment refers to automatically deploying code changes to the production environment. In PHP projects, automated deployment can be achieved through automated deployment tools (such as Jenkins). During the deployment process, you need to ensure that the cached configuration is also deployed correctly to the production environment.
You can write the cache-related configuration (such as Memcached's IP address and port number) and the deployment process of the cache code as a series of Shell scripts and add them to the automated deployment process. The following is an example of a simple cache deployment script:
#!/bin/bash # 部署缓存配置到生产环境 scp ./cache_config.php user@example.com:/var/www/config/ # 部署缓存代码到生产环境 rsync -r --delete ./cache_code/ user@example.com:/var/www/cache/
- Run cache integration tests and automated deployment
Finally, cache integration tests and automated deployment tasks can be configured into continuous integration and continuous deployment tools .
The continuous integration tool will automatically run cache integration tests every time the code is submitted. If the tests pass, the automated deployment tool will automatically deploy the code and cache configuration to the production environment. In this way, you can ensure that the cache works properly after every code modification and that the cache configuration is correctly deployed to the production environment.
Conclusion:
In PHP projects, through continuous integration and continuous deployment practices, the development process of data caching can be optimized. Choosing a suitable caching mechanism, writing caching code and cache integration tests, and automating the deployment of cache configurations are all key steps to ensure that data caching works properly. The practice of continuous integration and continuous deployment can improve development efficiency, reduce errors, and speed up the delivery of software products.
References:
- Memcached official documentation: https://www.php.net/manual/zh/book.memcached.php
- PHPUnit official documentation :https://phpunit.de/documentation.html
The above is the detailed content of Continuous integration and continuous deployment practices for PHP data caching. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


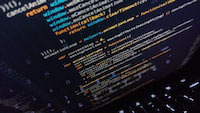
In modern software development, continuous integration (CI) has become an important practice to improve code quality and development efficiency. Among them, Jenkins is a mature and powerful open source CI tool, especially suitable for PHP applications. The following content will delve into how to use Jenkins to implement PHP continuous integration, and provide specific sample code and detailed steps. Jenkins installation and configuration First, Jenkins needs to be installed on the server. Just download and install the latest version from its official website. After the installation is complete, some basic configuration is required, including setting up an administrator account, plug-in installation, and job configuration. Create a new job On the Jenkins dashboard, click the "New Job" button. Select "Frees
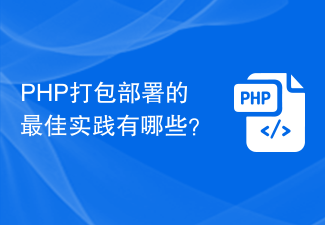
What are the best practices for PHP packaging and deployment? With the rapid development of Internet technology, PHP, as an open source programming language widely used in website development, more and more developers need to improve efficiency and stability in project deployment. This article will introduce several best practices for PHP packaging and deployment and provide relevant code examples. Use version control tools Version control tools such as Git, SVN, etc. can help developers effectively manage code changes. Use version control tools to easily track and roll back code, ensuring every deployment is
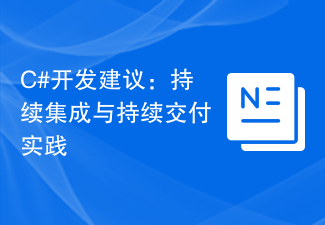
In the current software development process, continuous integration (ContinuousIntegration) and continuous delivery (ContinuousDelivery) have become key practices for development teams to improve product quality and speed up delivery. Whether you're a large software enterprise or a small team, you can benefit from both areas. This article will provide C# developers with some suggestions on continuous integration and continuous delivery practices. Automated builds and tests Automated builds and tests are the foundation of continuous integration. make
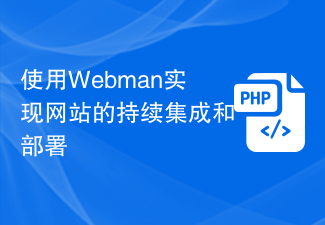
Using Webman to achieve continuous integration and deployment of websites With the rapid development of the Internet, the work of website development and maintenance has become more and more complex. In order to improve development efficiency and ensure website quality, continuous integration and deployment have become an important choice. In this article, I will introduce how to use the Webman tool to implement continuous integration and deployment of the website, and attach some code examples. 1. What is Webman? Webman is a Java-based open source continuous integration and deployment tool that provides
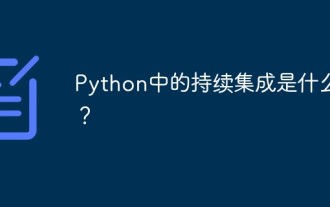
The Python language has become an indispensable part of modern software development, and continuous integration (CI) is part of the highly integrated and continuous delivery process, which can greatly improve the efficiency and quality of the development process. The purpose of CI is to minimize unnecessary errors by integrating code into a common code base and continuously running automated tests and static analysis tools. This article will discuss the principles of continuous integration in Python and its impact on the software development process. The Principle of Continuous Integration CI in Software Development
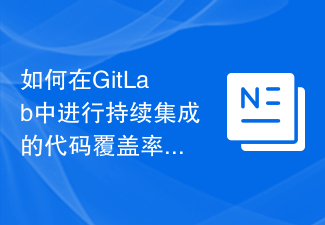
Title: Code coverage analysis and examples in GitLab continuous integration Introduction: As software development becomes more and more complex, code coverage analysis has become one of the important indicators to evaluate the quality of software testing. Using continuous integration to conduct code coverage analysis can help development teams monitor their code quality in real time and improve software development efficiency. This article will introduce how to perform continuous integration code coverage analysis in GitLab and provide specific code examples. 1. Code coverage analysis in GitLab 1.1 Code coverage

How to use React and Jenkins to build front-end applications with continuous integration and continuous deployment Introduction: In today's Internet development, continuous integration and continuous deployment have become important means for development teams to improve efficiency and ensure product quality. As a popular front-end framework, React, combined with Jenkins, a powerful continuous integration tool, can provide us with a convenient and efficient solution for building front-end applications for continuous integration and continuous deployment. This article will introduce in detail how to use React and Jenkins to support

In PHP development, maintaining code quality is crucial to improve software reliability, maintainability, and security. Continuously monitoring code quality proactively identifies issues, promotes early fixes, and prevents them from reaching production. In this article, we will explore how to set up a continuous monitoring pipeline for a PHP project using Jenkins and SonarQube. Jenkins: Continuous Integration Server Jenkins is an open source continuous integration server that automates the build, test and deployment process. It allows developers to set up jobs that will be triggered periodically and perform a series of tasks. For PHP projects, we can set up Jenkins jobs to complete the following tasks: check out the code from the version control system
