


Analyze the relationship between polymorphism and interfaces in PHP object-oriented programming
The relationship between polymorphism and interface in PHP object-oriented programming
In PHP object-oriented programming, polymorphism (Polymorphism) is an important concept, which makes Objects of different classes can be used in a unified way. Polymorphism is realized through the implementation of interface (Interface). This article will use code examples to analyze the relationship between polymorphism and interfaces in PHP object-oriented programming.
In PHP, an interface is an abstract structure that defines a set of methods. Classes express themselves with certain behavioral capabilities by implementing interfaces. Interfaces are declared with the interface keyword and can contain the definition of abstract methods. A class implements an interface through the implements keyword and must implement all methods defined in the interface.
Below we use a zoo example to illustrate the relationship between polymorphism and interfaces. Suppose we have three animal species: dogs, cats, and birds, all of which have the ability to make sounds. We can define an Animal interface that contains a sound method. Then let these three animal classes implement this interface and define their own specific behaviors in their respective sound methods.
interface Animal { public function sound(); } class Dog implements Animal { public function sound() { echo "汪汪汪!"; } } class Cat implements Animal { public function sound() { echo "喵喵喵!"; } } class Bird implements Animal { public function sound() { echo "唧唧喳喳!"; } }
In the above code, the Animal interface defines an abstract method sound(), which requires the class that implements the interface to implement this method. Then the Dog, Cat and Bird classes respectively implement the Animal interface and define their own sounding behaviors in the sound() method.
With the foundation of the above code, we can achieve polymorphism. We can create a zoo class that receives a parameter of type Animal and calls its sound method. Since the Dog, Cat, and Bird classes all implement the Animal interface, they can all be passed as parameters to the Zoo class and make their own sounds.
class Zoo { public function makeSound(Animal $animal) { $animal->sound(); } } $dog = new Dog(); $cat = new Cat(); $bird = new Bird(); $zoo = new Zoo(); $zoo->makeSound($dog); // 输出:汪汪汪! $zoo->makeSound($cat); // 输出:喵喵喵! $zoo->makeSound($bird); // 输出:唧唧喳喳!
In the above code, we created a Zoo class and defined a makeSound method in it, which receives an Animal type parameter and calls its sound() method. Then we created a Dog instance, a Cat instance, and a Bird instance respectively, and passed them as parameters to the makeSound method of the Zoo class, and finally output the sounds they made respectively.
Through the above examples, we can see the relationship between polymorphism and interfaces. The Zoo class does not care about the specific animal type, as long as they implement the Animal interface and have a sound() method, they can be passed to the makeSound method. This approach makes the code more flexible and extensible, and we can easily add new animal classes and let them make their own sounds in the zoo.
To summarize, in PHP object-oriented programming, polymorphism is achieved through the implementation of interfaces. An interface provides a specification and constraint, which defines a set of methods. Classes express themselves with certain behavioral capabilities by implementing the interface. Polymorphism allows objects of different classes to be used in a unified way, increasing the flexibility and scalability of the code.
The above is the analysis of the relationship between polymorphism and interfaces in PHP object-oriented programming in this article. I hope it can be helpful to readers.
The above is the detailed content of Analyze the relationship between polymorphism and interfaces in PHP object-oriented programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


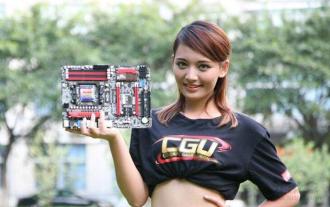
When we assemble the computer, although the installation process is simple, we often encounter problems in the wiring. Often, users mistakenly plug the power supply line of the CPU radiator into the SYS_FAN. Although the fan can rotate, it may not work when the computer is turned on. There will be an F1 error "CPUFanError", which also causes the CPU cooler to be unable to adjust the speed intelligently. Let's share the common knowledge about the CPU_FAN, SYS_FAN, CHA_FAN, and CPU_OPT interfaces on the computer motherboard. Popular science on the CPU_FAN, SYS_FAN, CHA_FAN, and CPU_OPT interfaces on the computer motherboard 1. CPU_FANCPU_FAN is a dedicated interface for the CPU radiator and works at 12V
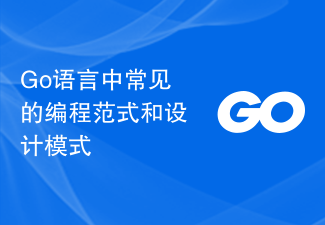
As a modern and efficient programming language, Go language has rich programming paradigms and design patterns that can help developers write high-quality, maintainable code. This article will introduce common programming paradigms and design patterns in the Go language and provide specific code examples. 1. Object-oriented programming In the Go language, you can use structures and methods to implement object-oriented programming. By defining a structure and binding methods to the structure, the object-oriented features of data encapsulation and behavior binding can be achieved. packagemaini
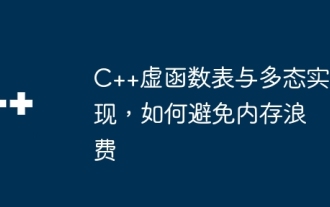
Virtual base classes optimize vtable memory overhead by allowing inheritance from multiple base classes without creating additional vtables. In the optimized code, the shape base class no longer has a virtual function table, and the circle and rectangle classes share the same virtual function table, thus reducing memory consumption.
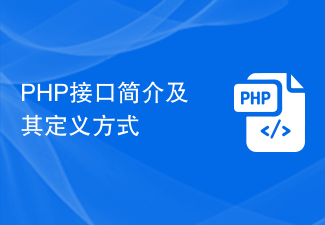
Introduction to PHP interface and how it is defined. PHP is an open source scripting language widely used in Web development. It is flexible, simple, and powerful. In PHP, an interface is a tool that defines common methods between multiple classes, achieving polymorphism and making code more flexible and reusable. This article will introduce the concept of PHP interfaces and how to define them, and provide specific code examples to demonstrate their usage. 1. PHP interface concept Interface plays an important role in object-oriented programming, defining the class application
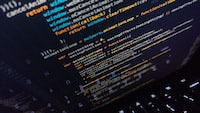
The reason for the error is in python. The reason why NotImplementedError() is thrown in Tornado may be because an abstract method or interface is not implemented. These methods or interfaces are declared in the parent class but not implemented in the child class. Subclasses need to implement these methods or interfaces to work properly. How to solve this problem is to implement the abstract method or interface declared by the parent class in the child class. If you are using a class to inherit from another class and you see this error, you should implement all the abstract methods declared in the parent class in the child class. If you are using an interface and you see this error, you should implement all methods declared in the interface in the class that implements the interface. If you are not sure which
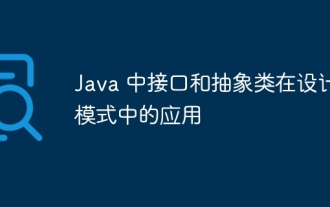
Interfaces and abstract classes are used in design patterns for decoupling and extensibility. Interfaces define method signatures, abstract classes provide partial implementation, and subclasses must implement unimplemented methods. In the strategy pattern, the interface is used to define the algorithm, and the abstract class or concrete class provides the implementation, allowing dynamic switching of algorithms. In the observer pattern, interfaces are used to define observer behavior, and abstract or concrete classes are used to subscribe and publish notifications. In the adapter pattern, interfaces are used to adapt existing classes. Abstract classes or concrete classes can implement compatible interfaces, allowing interaction with original code.
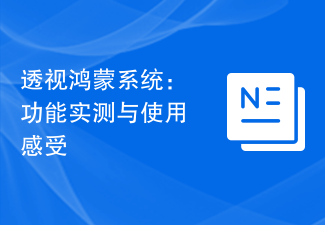
As a new operating system launched by Huawei, Hongmeng system has caused quite a stir in the industry. As a new attempt by Huawei after the US ban, Hongmeng system has high hopes and expectations. Recently, I was fortunate enough to get a Huawei mobile phone equipped with Hongmeng system. After a period of use and actual testing, I will share some functional testing and usage experience of Hongmeng system. First, let’s take a look at the interface and functions of Hongmeng system. The Hongmeng system adopts Huawei's own design style as a whole, which is simple, clear and smooth in operation. On the desktop, various

Java allows inner classes to be defined within interfaces and abstract classes, providing flexibility for code reuse and modularization. Inner classes in interfaces can implement specific functions, while inner classes in abstract classes can define general functions, and subclasses provide concrete implementations.
