Recommended visual test reporting tools for Golang
Golang’s visual test reporting tool recommendation
Introduction:
In the software development process, testing is an indispensable link. A good test report can help developers better analyze and understand test results, thereby optimizing software quality. This article will introduce several visual test reporting tools in Golang and demonstrate their usage through sample code.
- GoConvey
GoConvey is a powerful Golang testing framework that provides an easy-to-use and beautiful visual test reporting interface. Using GoConvey can greatly simplify the test code writing and result analysis process.
Sample code:
package main import ( "testing" . "github.com/smartystreets/goconvey/convey" ) func TestAddition(t *testing.T) { Convey("Given two numbers", t, func() { a := 5 b := 3 Convey("When adding them together", func() { result := a + b Convey("The result should be correct", func() { So(result, ShouldEqual, 8) }) }) }) }
In the sample code, we introduce GoConvey’s testing framework and assertion library. Using the Convey function to organize the test case, given two numbers, we add them and assert whether the result is correct or not. If everything is OK, the test results will appear in green in GoConvey's visual test reporting interface.
- Ginkgo & Gomega
Ginkgo is another popular Golang testing framework that is used in conjunction with the Gomega assertion library to provide BDD-like test writing and visual reporting capabilities.
Sample code:
package main import ( "testing" . "github.com/onsi/ginkgo" . "github.com/onsi/gomega" ) func TestAddition(t *testing.T) { RegisterFailHandler(Fail) RunSpecs(t, "Addition Suite") } var _ = Describe("Addition", func() { Context("Given two numbers", func() { a := 5 b := 3 It("should add them together correctly", func() { result := a + b Expect(result).To(Equal(8)) }) }) })
In the sample code, we use the Ginkgo testing framework and Gomega assertion library to write test cases. Use the Describe function to describe the test scenario, and then perform specific test operations in the It function. Test results will be displayed in an easy-to-understand manner on Ginkgo's visual test reporting interface.
- Testify
Testify is another popular testing tool library in Golang. It provides a rich set of assertion functions and utility functions, which can help developers write clear and readable tests. Test code and generate beautiful test reports.
Sample code:
package main import ( "testing" "github.com/stretchr/testify/assert" ) func TestAddition(t *testing.T) { a := 5 b := 3 result := a + b assert.Equal(t, 8, result) }
In the sample code, we use the assertion function assert.Equal of the Testify library to determine whether two values are equal. If the assertion fails, Testify will display the failure message in the test report, otherwise, it will display the test passing message.
Conclusion:
In Golang, we can use visual test reporting tools such as GoConvey, Ginkgo & Gomega, and Testify to improve testing efficiency and readability. Through these tools, developers can better organize test cases and analyze test results, thereby improving software quality. I hope the introduction in this article will help you choose the appropriate visual test reporting tool in your Golang project.
The above is the detailed content of Recommended visual test reporting tools for Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


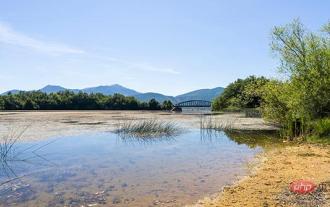
1. Introduction Plotly is a very famous and powerful open source data visualization framework. It displays information by building interactive charts based on browser display in the form of web, and can create dozens of beautiful charts and maps. 2. Drawing grammar rules 2.1 Offline drawing method There are two ways to draw images in Plotly, online and offline. Because online drawing requires registering an account to obtain an APIkey, which is more troublesome, this article only introduces the offline drawing method. There are two methods for offline drawing: plotly.offline.plot() and plotly.offline.iplot(). The former generates an image file in html format in the current working directory in an offline way and opens it automatically;
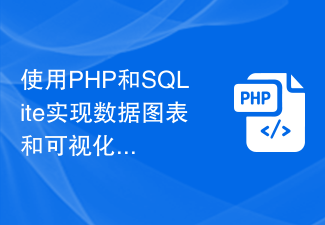
Using PHP and SQLite to implement data charts and visualization overview: With the advent of the big data era, data charts and visualizations have become an important way to display and analyze data. In this article, we will introduce how to use PHP and SQLite to implement data charts and visualization functions. Take an example as an example to show how to read data from a SQLite database and use a common data chart library to display the data. Preparation: First, you need to ensure that PHP and SQLite databases have been installed. If it is not installed, you can
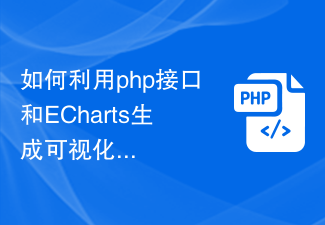
In today's context where data visualization is becoming more and more important, many developers hope to use various tools to quickly generate various charts and reports so that they can better display data and help decision-makers make quick judgments. In this context, using the Php interface and ECharts library can help many developers quickly generate visual statistical charts. This article will introduce in detail how to use the Php interface and ECharts library to generate visual statistical charts. In the specific implementation, we will use MySQL
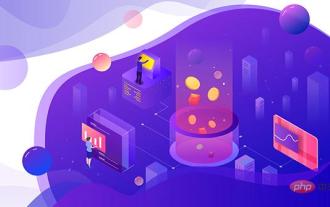
Introduction The proliferation of programming and technology applied to finance is inevitable, and growth never seems to decline. One of the most interesting parts of applied programming is the interpretation and visualization of historical or real-time stock data. Now, for visualizing general data in python, modules like matplotlib, seaborn etc. come into play, however, when it comes to visualizing financial data, Plotly will be the first choice as it provides in-built functions with interactive visuals. Here I would like to introduce an unsung hero, which is nothing more than a brother library of the mplfinance library matplotlib. We all know how versatile the matplotlib package is and can easily plot any type of data.
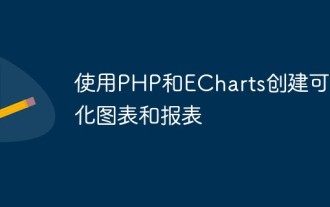
With the advent of the big data era, data visualization has become an important tool for corporate decision-making. Various strange data visualization tools emerge in endlessly, among which ECharts has received widespread attention and application due to its powerful functions and good user experience. As a mainstream server-side language, PHP also provides rich data processing and chart display functions. This article will introduce how to use PHP and ECharts to create visual charts and reports. Introduction to ECharts ECharts is an open source visual chart library developed by
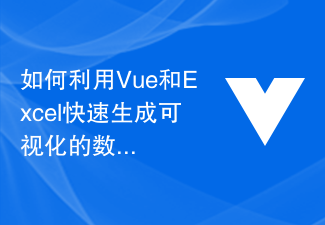
How to use Vue and Excel to quickly generate visual data reports. With the advent of the big data era, data reports have become an indispensable part of corporate decision-making. However, the traditional way of producing data reports is cumbersome and inefficient. Therefore, we need a more convenient method to generate visual data reports. This article will introduce how to use the Vue framework and Excel tables to quickly generate visual data reports, and attach corresponding code examples. First, we need to create a Vue-based project. Can use Vue
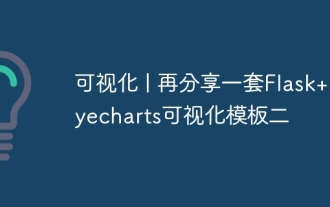
In this issue, I will share with you a set of <Flask+Pyecharts Visual Template 2> suitable for beginners. I hope it will be helpful to you.
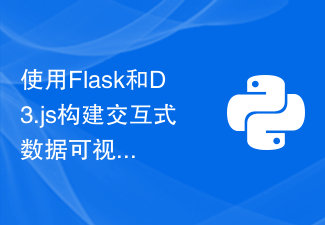
In recent years, data analysis and data visualization have become indispensable skills in many industries and fields. It is very important for data analysts and researchers to present large amounts of data in front of users and allow users to understand the meaning and characteristics of the data through visualization. To meet this need, it has become a trend to use D3.js to build interactive data visualizations in web applications. In this article, we'll cover how to build interactive data visualizations for the web using Flask and D3.js
