


Technical details analysis and practical guide for PHP code testing function
Technical details analysis and practical guide for PHP code testing function
Introduction
In the software development process, code testing is a crucial part ring. In the field of PHP development, code testing is an indispensable technical means that can improve code quality, reduce the number of bugs, and enhance code maintainability. This article aims to explore the technical details of PHP code testing and provide practical guidelines so that readers can better understand and apply various techniques of PHP code testing.
1. Why code testing?
Code testing is to find errors and defects in the program to ensure the correctness and reliability of the program. By conducting code testing, problems can be discovered and resolved early, thereby reducing software maintenance costs and improving code quality. In PHP development, code testing is also to ensure the stable operation of the website or application and reduce security vulnerabilities.
2. Common PHP code testing techniques
- Unit testing
Unit testing refers to testing the smallest testable unit in the program, such as Function, method or class, etc. The purpose of unit testing is to verify that the unit operates correctly as expected. In PHP, we can use PHPUnit for unit testing.
The following is a simple example to demonstrate how to use PHPUnit for unit testing:
<?php class TestString extends PHPUnit_Framework_TestCase { public function testStringLength() { $string = "Hello, World!"; $this->assertEquals(13, strlen($string)); } } ?>
- Integration testing
Integration testing refers to testing multiple components in the program Units are tested in combination to verify that they work correctly together. In PHP, we can use PHPUnit's integration testing function to perform integration testing.
Here is an example that demonstrates how to use PHPUnit for integration testing:
<?php class TestCalculator extends PHPUnit_Framework_TestCase { public function testAddition() { $calculator = new Calculator(); $result = $calculator->add(2, 3); $this->assertEquals(5, $result); } public function testSubtraction() { $calculator = new Calculator(); $result = $calculator->subtract(5, 3); $this->assertEquals(2, $result); } } ?>
- Behavior-Driven Development (BDD)
BDD (Behavior-Driven Development ) is a behavior-centered development approach that emphasizes collaboration between teams and business experts. In PHP, we can use Behat for BDD testing. Behat uses the Gherkin language to describe test scenarios and verify that the code meets expected behavior through automated execution.
The following is an example that demonstrates how to use Behat for BDD testing:
feature file:
Feature: Login functionality In order to access the dashboard As a registered user I want to be able to login to the system Scenario: Successful login Given I am on the login page When I fill in "Email" with "test@example.com" And I fill in "Password" with "password" And I press "Login" Then I should see "Welcome, John Doe"
step definition file:
<?php use BehatBehatContextContext; use BehatBehatTesterExceptionPendingException; use BehatGherkinNodePyStringNode; use BehatGherkinNodeTableNode; /** * Defines application features from the specific context. */ class FeatureContext implements Context { /** * @Given I am on the login page */ public function iAmOnTheLoginPage() { // Visit login page } /** * @When I fill in :arg1 with :arg2 */ public function iFillInWith($field, $value) { // Fill in form field with value } /** * @When I press :arg1 */ public function iPress($button) { // Press form button } /** * @Then I should see :arg1 */ public function iShouldSee($text) { // Assert text is visible on page } } ?>
3. Practice Guide
- Use a testing framework that complies with specifications
When testing PHP code, it is very important to choose a testing framework that complies with specifications and has rich support. PHPUnit is one of the most commonly used testing frameworks in the PHP field. It is powerful and supports a variety of testing technologies. In addition, there are BDD testing frameworks such as Behat, which can help developers better understand business requirements and conduct behavior-driven development.
- Coverage testing
In addition to unit testing and integration testing, code coverage testing should also be performed. Test coverage reports allow you to evaluate the completeness of your tests and the robustness of your code. Xdebug is a commonly used PHP extension that provides test coverage functionality.
- Continuous Integration
Combining code testing with continuous integration tools (such as Jenkins, Travis CI, etc.) can achieve automated testing. The continuous integration tool automatically runs code tests every time the code is submitted or merged, providing real-time test feedback. This can greatly improve the team's development efficiency and code quality.
Conclusion
This article introduces the technical details and practical guidelines for PHP code testing. By conducting code testing, developers can find and resolve errors and defects in the program, thereby improving code quality and maintainability. I hope this article can help readers better understand and apply PHP code testing technology.
The above is the detailed content of Technical details analysis and practical guide for PHP code testing function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


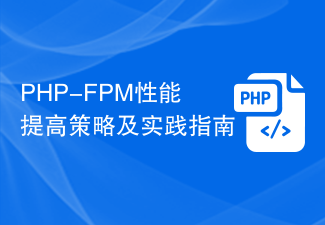
Introduction to PHP-FPM Performance Improvement Strategies and Practice Guide: With the rapid development of the Internet and the increasing number of website visits, it is particularly important to improve the performance of PHP applications. PHPFastCGIProcessManager (PHP-FPM) is a commonly used PHP process manager that can improve the performance of PHP applications through a series of strategies and practices. This article will introduce some PHP-FPM performance improvement strategies, combined with specific code examples, to help readers better understand
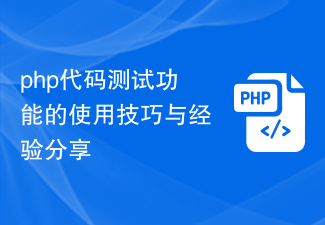
Tips and experience sharing on the use of PHP code testing function When developing PHP applications, code testing is a very important link. Code testing can check and verify the correctness of the code to ensure the stable operation of the program. This article will introduce some tips and experiences in PHP code testing to help developers better conduct code testing. Using the unit testing framework Unit testing is a test for each independent functional module in the program. Using a unit testing framework simplifies the testing process and provides some powerful assertion and test result reporting
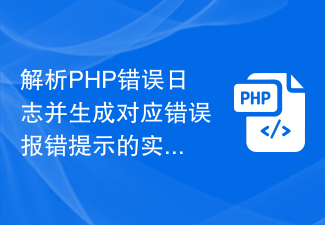
A practical guide for parsing PHP error logs and generating corresponding error reports. Error logs are a very important tool for developers. They can help us quickly locate and solve problems in the code. The PHP error log records various errors, warnings and prompts during the running of the program. By analyzing the error log, we can understand the problems in the program and take appropriate measures to repair them. This article will introduce how to parse PHP error logs and generate corresponding error prompts to help developers work more efficiently.
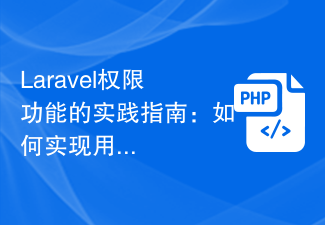
Practical guide for Laravel permission function: How to implement user permission approval process, specific code examples are required Introduction: In today's era of rapid development of the Internet, the management of system permissions has become more and more important. As a popular PHP development framework, Laravel provides a set of simple and powerful permission management functions that can help developers easily implement the user permission approval process. This article will introduce how to implement the user permission approval process in the Laravel framework and give specific code examples. 1. Permissions
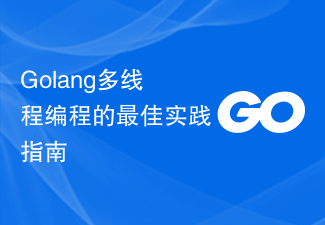
Best Practice Guide for Multi-Threaded Programming in Golang The Go language (Golang) is a fast, simple and powerful programming language with excellent concurrent programming capabilities. By supporting native goroutines and channels, Golang provides developers with a simple and efficient way to perform multi-threaded programming. This article will introduce the best practices of multi-threaded programming in Golang, including how to create and manage goroutines, how to use channels for inter-thread communication, and how to
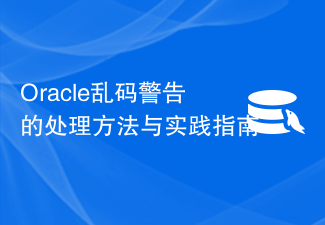
Oracle Garbled Code Warning Handling Methods and Practical Guidelines With the process of globalization, enterprises often encounter garbled code problems in database management. As the industry's leading relational database management system, Oracle database is inevitably prone to garbled warnings. This article will conduct an in-depth discussion on the problem of Oracle garbled characters, discuss common causes of garbled characters, processing methods and practical guidelines, and provide specific code examples for readers' reference. 1. Analysis of the causes of garbled codes. The causes of garbled codes in the Oracle database can be many.

JUnit Unit Testing Best Practice Guide Introduction: In software development, unit testing is one of the important means to ensure code quality and stability. JUnit is the most commonly used unit testing framework in Java. It is simple, easy to use and powerful. This article will introduce the best practices for JUnit unit testing, including writing maintainable test cases, using assertions, using annotations and naming conventions, etc. 1. Write maintainable test cases Writing maintainable test cases is the basis of JUnit unit testing. Here are some ways to write maintainable
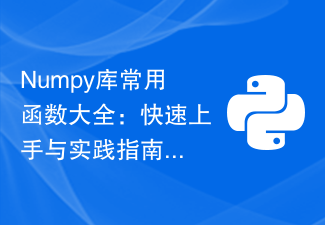
The Numpy library is one of the most commonly used data processing libraries in Python. It is widely loved by data analysts for its efficient and convenient operation methods. In the Numpy library, there are many commonly used functions that can help us complete data processing tasks quickly and efficiently. This article will introduce some commonly used Numpy functions, and provide code examples and practical application scenarios so that readers can get started with the Numpy library faster. 1. Create an array numpy.array function prototype: numpy.array(obj
