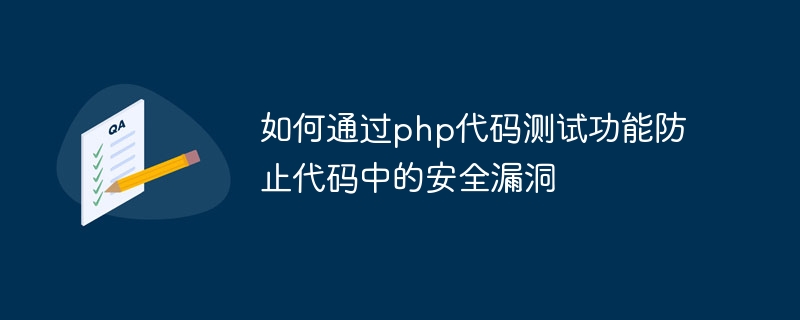
How to prevent security vulnerabilities in the code through the PHP code testing function
Introduction:
When developing web applications, security issues have always been a very important issue for developers. a matter of concern. Malicious attackers may exploit vulnerabilities in the code to conduct various attacks, such as injection attacks, cross-site scripting attacks, etc. To protect application security, we need to adequately test our code to find and fix security vulnerabilities. This article will introduce how to prevent security vulnerabilities in the code through PHP code testing functions.
1. Code review
In PHP development, code review is a very important security testing task. By reviewing the code, we can discover security risks and vulnerabilities so that we can repair and prevent them. Code review is mainly conducted from the following aspects:
- Verify user input: User input data is the most vulnerable place to attack. When using user input data, strict verification and filtering should be performed to avoid injection attacks through user input. The following is a simple example:
<?php
$name = $_GET['name'];
if (!preg_match("/^[a-zA-Z ]*$/",$name)) {
die("只允许字母和空格");
}
?>
Copy after login
- Prevent SQL injection: SQL injection is a common attack method, in which attackers perform illegal operations by entering malicious SQL statements. In order to prevent SQL injection, we need to use parameterized queries or precompiled statements to process user-entered data and ensure data security. The following is a simple example:
<?php
$stmt = $pdo->prepare('SELECT * FROM users WHERE username = :username');
$stmt->bindParam(':username', $username);
$stmt->execute();
?>
Copy after login
- Prevent cross-site scripting attacks: Cross-site scripting attacks (XSS) are a common attack method in which attackers insert malicious code into web pages. Script to steal user information. In order to prevent XSS attacks, we need to filter and escape the output data. The following is a simple example:
<?php
$name = $_GET['name'];
echo htmlspecialchars($name, ENT_QUOTES, 'UTF-8');
?>
Copy after login
2. Security testing tools
In addition to code review, we can also use some specialized tools to conduct security testing in order to comprehensively discover vulnerabilities in the code and safety hazards. The following are some common security testing tools:
- PHP Security Scanner: This is an open source tool specifically used for PHP security testing. It scans code for vulnerabilities, discovers possible security issues, and provides fix recommendations.
- OWASP ZAP: This is a powerful web application security testing tool that can conduct comprehensive security testing of web applications, including vulnerability scanning, security configuration inspection, etc.
- Kali Linux: This is a Linux distribution that integrates various security testing tools. Using Kali Linux, you can perform various security tests, including code security tests, through the command line interface or graphical interface.
3. Security Testing Practice
In addition to using code review and security testing tools, we can also conduct some security testing practices based on actual conditions to better discover and repair security issues. The following are some common security testing practices:
- Input boundary testing: When performing user input verification, we should conduct some boundary testing to ensure that our verification logic is accurate and reliable. For example, if the length range of the entered user name is 6-20 characters, we should test the case where 5 characters or more than 20 characters are entered.
- Authorization testing: When performing user authorization, we should test whether the authorization logic is correct in various situations. For example, test whether an ordinary user can access the administrator's permissions, or test whether a non-logged-in user can access pages that require login.
- Exception handling testing: We should test whether the processing logic under various abnormal situations is correct. For example, test the processing logic when the database connection fails, or test the processing logic when the file upload fails.
Conclusion:
Through code review, security testing tools and security testing practices, we can effectively prevent security vulnerabilities in the code and protect the security of web applications. Developers should develop the habit of adequately testing their code to ensure the security and stability of their applications.
The above is the detailed content of How to prevent security vulnerabilities in your code with php code testing functionality. For more information, please follow other related articles on the PHP Chinese website!