


Security analysis and protection strategies for PHP data caching
Security analysis and protection strategy of PHP data caching
1. Introduction
When developing web applications, data caching improves performance and response speed One of the commonly used techniques. However, due to the particularity of the caching mechanism, there may be security issues. This article will analyze the security of PHP data cache and provide corresponding protection strategies.
2. Security Analysis
- Cache penetration
Cache penetration means that malicious users bypass the cache and directly query the database by constructing malicious requests. Generally speaking, after receiving a request, the cache system will first check whether the corresponding data exists in the cache. If it does not exist, it will query the database and store the results in the cache. An attacker can construct query conditions so that the results will never be cached, thus querying the database every time, causing excessive pressure on the database.
Solution: Before querying the database, you can check the validity of the request parameters to verify the legality of the user's request. For example, for user IDs, regular expressions or filters can be used to limit and exclude abnormal or illegal parameters.
Code example:
// 将用户ID作为缓存Key $cacheKey = 'user_' . $userId; // 判断缓存中是否存在数据 if ($cache->exists($cacheKey)) { // 从缓存中获取数据 $data = $cache->get($cacheKey); } else { // 参数合法性检查 if (preg_match('/^d+$/', $userId)) { // 从数据库查询数据 $data = $db->query('SELECT * FROM users WHERE id = ?', [$userId]); // 将查询结果存入缓存 $cache->set($cacheKey, $data); } else { // 参数非法,返回错误消息 $data = 'Invalid user ID'; } }
- Cache penetration protection and Bloom filter
The above method implements the legality check of user ID, but for other queries conditions, there are still potential safety hazards. In order to solve the cache penetration problem more completely, you can use Bloom Filter to determine whether the query conditions exist in the cache. Bloom filter is a data structure based on hash function, which can determine whether an element belongs to a set. It has the advantages of efficient query performance and space consumption.
Solution: Before querying the database, use the hash value of the query condition as the input of the Bloom filter and determine whether it exists in the Bloom filter. If the Bloom filter determines that it does not exist, the query failure will be returned directly, avoiding the query operation on the database.
Code example:
// 使用布隆过滤器库 require_once 'bloom_filter.php'; // 创建布隆过滤器实例 $bf = new BloomFilter(); // 将查询条件的哈希值插入布隆过滤器 $bf->add(hash('md5', $condition)); // 判断查询条件是否存在于布隆过滤器 if ($bf->contains(hash('md5', $condition))) { // 从缓存中获取数据 $data = $cache->get($cacheKey); } else { // 参数非法,返回错误消息 $data = 'Invalid condition'; }
- Cache breakdown
Cache breakdown means that after a certain hot data cache fails, a large number of requests access the database at the same time, causing database pressure is too big. An attacker can intentionally expire hotspot data, causing cache penetration.
Solution: In order to avoid cache breakdown, you can set a never-expiry policy for hotspot data. At the same time, when the cache fails, use a mutex (Mutex) to avoid concurrent queries to the database. Only one request queries the database, and other requests wait. search result.
Code example:
// 获取缓存锁 $lockKey = 'cache_lock_' . $cacheKey; if ($cache->add($lockKey, 1, 10)) { // 查询数据库 $data = $db->query('SELECT * FROM hot_data WHERE id = ?', [$cacheKey]); // 将查询结果存入缓存,并设置过期时间 $cache->set($cacheKey, $data, 60); // 释放缓存锁 $cache->delete($lockKey); } else { // 等待其他请求查询结果 usleep(1000); // 从缓存中获取数据 $data = $cache->get($cacheKey); }
3. Summary
Although PHP data caching can improve performance, security issues also need to be paid attention to. By analyzing issues such as cache penetration and cache breakdown, corresponding protection strategies can be adopted to ensure cache security. In actual development, according to specific needs and scenarios, the above methods and other security technologies can be used comprehensively to ensure the security of PHP data cache.
The above is the detailed content of Security analysis and protection strategies for PHP data caching. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


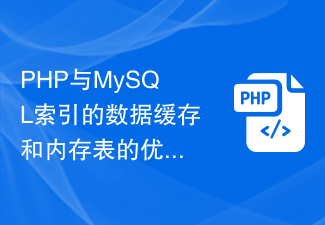
Optimization strategies for data caching and in-memory tables of PHP and MySQL indexes and their impact on query performance Introduction: PHP and MySQL are a very common combination when developing and optimizing database-driven applications. In the interaction between PHP and MySQL, index data caching and memory table optimization strategies play a crucial role in improving query performance. This article will introduce the optimization strategies for data caching and memory tables of PHP and MySQL indexes, and explain their impact on query performance in detail with specific code examples.
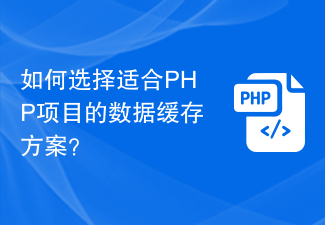
How to choose a data caching solution suitable for PHP projects? With the rapid development of the Internet and the advent of the big data era, how to efficiently handle data access and caching has become an important issue for PHP projects. As a common performance optimization method, data caching can effectively improve the response speed and user experience of the website. However, when choosing a data caching solution suitable for PHP projects, we need to consider a series of factors, including cache type, data access mode, caching strategy, etc. This article will discuss how to choose from these aspects
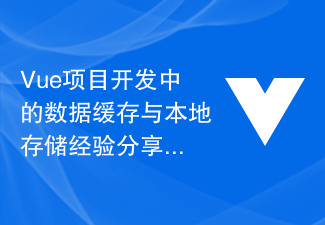
Data caching and local storage experience sharing in Vue project development In the development process of Vue project, data caching and local storage are two very important concepts. Data caching can improve application performance, while local storage can achieve persistent storage of data. In this article, I will share some experiences and practices in using data caching and local storage in Vue projects. 1. Data caching Data caching is to store data in memory so that it can be quickly retrieved and used later. In Vue projects, there are two commonly used data caching methods:
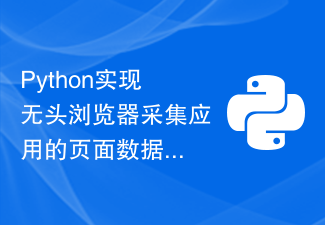
Analysis of page data caching and incremental update functions for headless browser collection applications implemented in Python Introduction: With the continuous popularity of network applications, many data collection tasks require crawling and parsing web pages. The headless browser can fully operate the web page by simulating the behavior of the browser, making the collection of page data simple and efficient. This article will introduce the specific implementation method of using Python to implement the page data caching and incremental update functions of a headless browser collection application, and attach detailed code examples. 1. Basic principles: headless
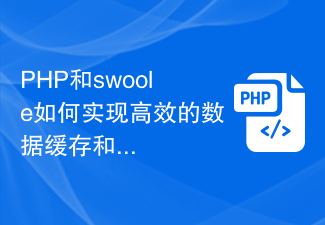
How do PHP and swoole achieve efficient data caching and storage? Overview: In web application development, data caching and storage are a very important part. PHP and swoole provide an efficient method to cache and store data. This article will introduce how to use PHP and swoole to achieve efficient data caching and storage, and give corresponding code examples. 1. Introduction to swoole: swoole is a high-performance asynchronous network communication engine developed for PHP language. It can
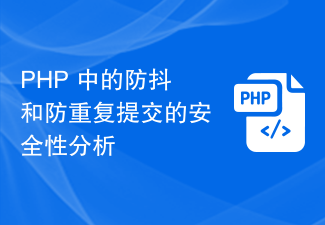
Security Analysis of Anti-Shake and Anti-Duplicate Submission in PHP Introduction: With the development of websites and applications, web forms have become one of the important ways to interact with users. After the user fills out the form and clicks the submit button, the server will receive and process the submitted data. However, due to network delays or user misoperations, the form may be submitted multiple times. Repeated submissions will not only increase the load on the server, but may also cause various security issues, such as repeated data insertion, unauthorized operations, etc. In order to solve these problems, we can use anti-shake
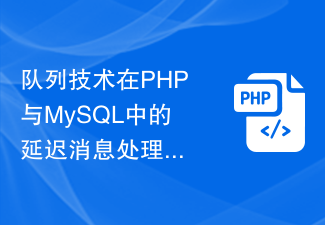
Application of queue technology in delayed message processing and data caching in PHP and MySQL Introduction: With the rapid development of the Internet, the demand for real-time data processing is getting higher and higher. However, traditional database operation methods often cause performance bottlenecks when processing large amounts of real-time data. In order to solve this problem, queue technology came into being, which can help us implement asynchronous processing of data and improve system performance and response speed. This article will introduce the application of queue technology in delayed message processing and data caching in PHP and MySQL, and through specific code
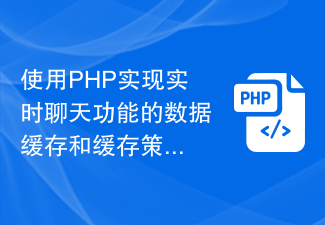
Data caching and caching strategies for real-time chat function using PHP Introduction: In modern social media and Internet applications, real-time chat function has become an important part of user interaction. In order to provide an efficient real-time chat experience, data caching and caching strategies have become the focus of developers. This article will introduce data caching and caching strategies for implementing real-time chat functionality using PHP, and provide relevant code examples. 1. The role of data caching Data caching is to reduce the burden on the database and improve the response speed of the system. in live chat
