


An analysis of how to use Vue to implement scalable server-side communication
Analysis on how to use Vue to achieve scalable server-side communication
With the development of the Internet, server-side communication has become indispensable in modern application development part. In order to achieve scalable and flexible server-side communication, we can use the Vue framework to simplify the development process. This article will introduce how to implement scalable server-side communication through Vue, and demonstrate the specific implementation method through code examples.
First, we need to create a Vue instance to manage the related logic of server communication. We can handle various server request and response logic in Vue's life cycle hook function. The following is a simple sample code:
// 创建Vue实例 new Vue({ data: { serverData: null }, created() { // 在created钩子函数中发起服务器请求 this.fetchServerData(); }, methods: { fetchServerData() { // 使用axios库发送GET请求 axios.get('/api/somedata') .then(response => { // 保存服务器响应的数据 this.serverData = response.data; }) .catch(error => { console.error(error); }); }, sendDataToServer(data) { // 使用axios库发送POST请求 axios.post('/api/somedata', data) .then(response => { // 处理服务器返回的数据 console.log(response.data); }) .catch(error => { console.error(error); }); } } });
In the above code, we define a serverData
attribute in Vue's data
option to save the server return The data. In the created
hook function, we call the fetchServerData
method to initiate a GET request, and save the data returned by the server to serverData# in the
then callback function. ##Attributes.
methods option, we define a
sendDataToServer method to send POST requests to the server and process the data returned by the server.
// 创建Vue实例 new Vue({ data: { serverData: null, computedData: null }, created() { // 在created钩子函数中发起服务器请求 this.fetchServerData(); }, computed: { processData() { // 使用computed属性实时计算服务器返回的数据 return this.transformData(this.serverData); } }, watch: { processData(newValue) { // 监听computed属性的变化,并更新computedData属性 this.computedData = newValue; } }, methods: { fetchServerData() { //... }, transformData(data) { // 数据处理逻辑 } } });
computedattribute
processData, Used to calculate the value of the
serverData attribute in real time. We also added a
watcher to listen for changes in the
processData attribute and update the value of the
computedData attribute when it changes.
The above is the detailed content of An analysis of how to use Vue to implement scalable server-side communication. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


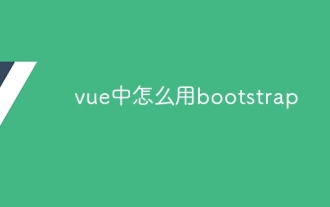
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
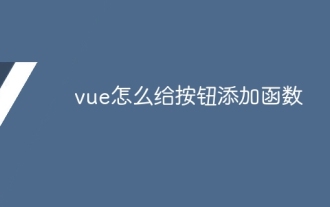
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
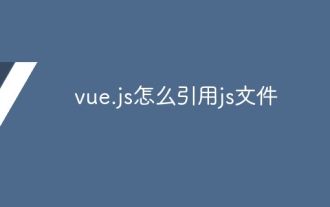
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
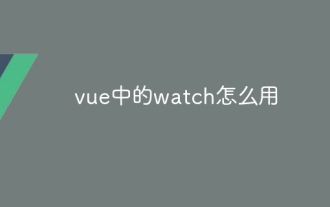
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
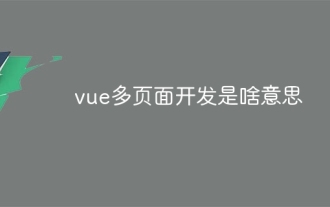
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
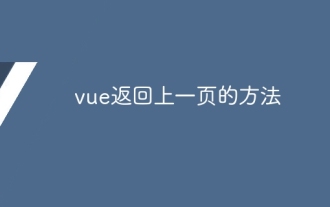
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
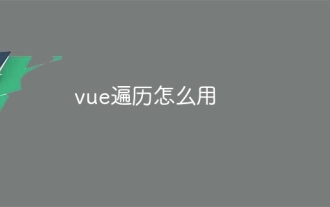
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
