


How to use load balancer to improve PHP's high concurrency processing capabilities
How to use load balancer to improve PHP’s high concurrent processing capabilities
With the rapid development of the Internet, the number of concurrent visits to Web applications is increasing. How to improve the application High concurrency processing capabilities have become the focus of developers. As a scripting language for developing web applications, PHP has relatively weak high-concurrency processing capabilities, so we need to take some measures to improve its performance.
Load balancer is a common solution that handles concurrent requests by distributing traffic to multiple servers. The following will introduce how to use load balancers to improve PHP's high concurrency processing capabilities, and give corresponding code examples.
Step 1: Build a load balancer
There are many ways to build a load balancer. The following is an example of using Nginx as a load balancer.
First, install Nginx:
apt-get install nginx
Then, edit the Nginx configuration file (usually located at /etc/nginx/nginx.conf) and set the load balancing strategy and back-end server:
http { upstream backend { server backend1.example.com; server backend2.example.com; server backend3.example.com; } server { listen 80; server_name example.com; location / { proxy_pass http://backend; } } }
In the above configuration, the upstream backend
part defines the address of the backend server, and the server
part defines the listening port and access path of the load balancer.
Restart Nginx to make the configuration take effect:
service nginx restart
At this point, the construction of the load balancer is completed. Next, we will introduce how to use load balancers in PHP to improve high-concurrency processing capabilities.
Step 2: PHP code optimization
In PHP code, high concurrency processing capabilities can be improved through multi-threading and asynchronous processing. Specific code examples are given below:
// 多线程处理 function process($url) { $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_TIMEOUT, 10); $result = curl_exec($ch); curl_close($ch); return $result; } $urls = array( 'http://backend1.example.com', 'http://backend2.example.com', 'http://backend3.example.com' ); $threads = array(); $results = array(); foreach ($urls as $url) { $thread = new Thread('process', $url); $threads[] = $thread; $thread->start(); } foreach ($threads as $thread) { $result = $thread->join(); $results[] = $result; } // 异步处理 $mh = curl_multi_init(); $handles = array(); foreach ($urls as $url) { $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_TIMEOUT, 10); curl_multi_add_handle($mh, $ch); $handles[] = $ch; } $running = null; do { curl_multi_exec($mh, $running); } while ($running > 0); $results = array(); foreach ($handles as $ch) { $result = curl_multi_getcontent($ch); curl_multi_remove_handle($mh, $ch); curl_close($ch); $results[] = $result; } curl_multi_close($mh);
In the above code, the process
function uses the curl function to send HTTP requests and process multiple requests asynchronously through multi-threading or asynchronously to improve PHP's high-concurrency processing. ability.
To sum up, by building a load balancer and optimizing PHP code, we can effectively improve PHP's high concurrency processing capabilities. Load balancers distribute traffic across multiple servers, while multi-threading and asynchronous processing can handle multiple requests at the same time, thereby increasing the concurrent processing capabilities of your application.
Of course, in addition to load balancer and code optimization, other optimization measures such as database optimization, static resource caching, etc. can also further improve the application's high concurrency processing capabilities. Hope this article can provide you with some useful reference.
The above is the detailed content of How to use load balancer to improve PHP's high concurrency processing capabilities. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


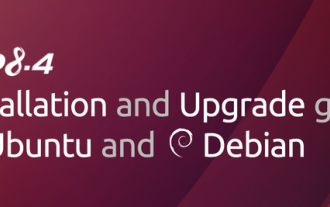
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
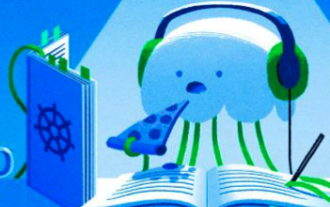
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
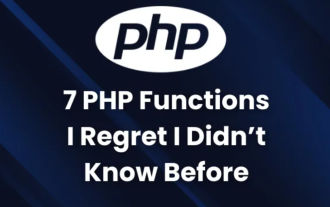
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
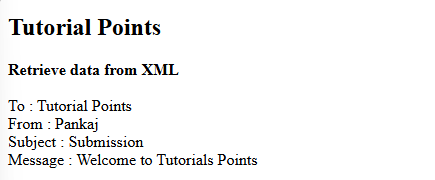
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
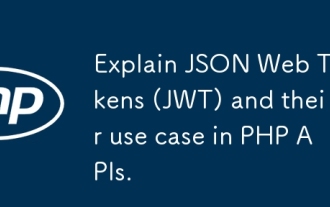
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
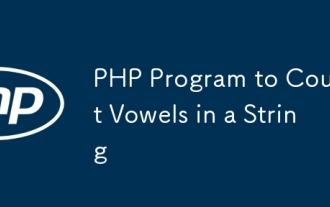
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
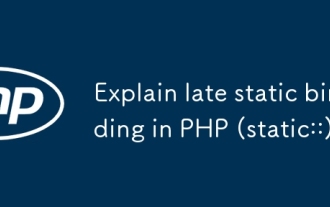
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
