An analysis of how to use Vue to optimize server-side communication
An analysis of how to use Vue to optimize server-side communication
Introduction:
As the complexity of front-end pages continues to increase, server-side communication has also become a important link. In order to improve performance and user experience, we need to optimize server-side communication. This article will introduce how to use the Vue framework to optimize server-side communication and provide some code examples.
1. Use Axios to send asynchronous requests
Axios is a Promise-based HTTP library that can send HTTP requests in browsers and Node.js. It has a cleaner API and better error handling mechanism, so the code for server-side communication can be greatly simplified. The following is a sample code for sending a GET request using Axios:
import axios from 'axios'; axios.get('/api/users') .then((response) => { console.log(response.data); }) .catch((error) => { console.log(error); });
Axios also supports other HTTP methods such as POST, PUT, and DELETE, which can be used in a similar way. By using Axios to send asynchronous requests, we can communicate with the server side more easily and process the returned data.
2. Use Vue’s computed attribute to cache data
In server-side communication, some data may be used in multiple places on the page. If it is re-obtained from the server every time it needs to be used, it will lead to poor performance. Poor and poor user experience. In order to avoid repeated server requests, we can use Vue's computed attribute to cache data. The following is a sample code:
import axios from 'axios'; import { computed, ref } from 'vue'; export default { setup() { const users = ref([]); const fetchUsers = () => { axios.get('/api/users') .then((response) => { users.value = response.data; }) .catch((error) => { console.log(error); }); }; fetchUsers(); // 使用computed属性缓存数据 const filteredUsers = computed(() => { return users.value.filter(user => user.age >= 18); }); return { filteredUsers, }; }, };
In the above code, after obtaining the user list, we filter the users in the computed attribute and only return users whose age is greater than or equal to 18 years old. In this way, filteredUsers can be used directly to get the filtered user list every time the page is rendered without sending the request again.
3. Use Vuex for state management and server-side data caching
For larger applications or situations where data needs to be shared between multiple components, you can use Vuex for state management and server-side data. cache. The following is a sample code:
import axios from 'axios'; import { createStore } from 'vuex'; const store = createStore({ state() { return { users: [], }; }, mutations: { setUsers(state, users) { state.users = users; }, }, actions: { fetchUsers(context) { axios.get('/api/users') .then((response) => { context.commit('setUsers', response.data); }) .catch((error) => { console.log(error); }); }, }, getters: { filteredUsers(state) { return state.users.filter(user => user.age >= 18); }, }, }); export default store;
In the above code, we define a state named users, use mutations to update the state, use actions to asynchronously obtain data and submit mutations to update the state. A calculated property named filteredUsers is defined in getters for caching filtered user data.
By using Vuex, we can cache server-side data into the application's state, thereby avoiding re-fetching the data every time and optimizing the performance and user experience of server-side communication.
Conclusion:
By using the Vue framework, we can use Axios to send asynchronous requests, use computed attributes to cache data, and use Vuex for state management and server-side data caching to optimize server-side communication. These optimizations not only improve performance but also improve user experience. I hope this article has provided you with some inspiration and help in optimizing server-side communication.
Reference: https://vuejs.org/
https://axios-http.com/
The above is the detailed content of An analysis of how to use Vue to optimize server-side communication. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


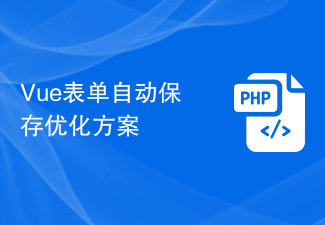
How to optimize form automatic saving in Vue development. In Vue development, form automatic saving is a common requirement. When the user fills out the form, we hope to automatically save the form data when the user leaves the page or closes the browser to prevent the user's input information from being lost. This article will introduce how to solve the form automatic saving problem in Vue development through optimization. Using the Vue component's lifecycle hook function The Vue component's lifecycle hook function provides the ability to execute custom logic during the component's life cycle. we can
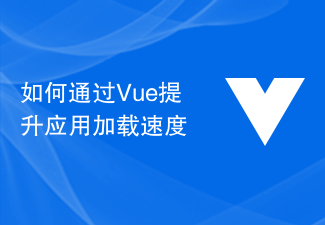
How to improve application loading speed with Vue Vue is a popular JavaScript framework for building user interfaces. Vue has rich features and powerful performance optimization options that can help us improve the loading speed of our application. This article will introduce some optimization techniques you can use with Vue to speed up the loading of your application. Use CDN to introduce Vue: By introducing Vue as an external resource, CDN can be used to improve loading speed. At the head of the HTML file, introduce Vue through the following code:
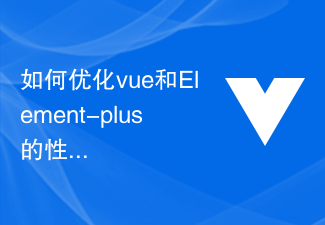
How to optimize the performance of Vue and ElementPlus and improve web page loading speed. In modern web applications, performance is a very important consideration. When we build applications using Vue.js and ElementPlus, we need to ensure that web pages load quickly to provide users with a good experience. This article will introduce some methods to optimize the performance of Vue and ElementPlus and provide corresponding code examples. Use the production environment build before deploying the web application,
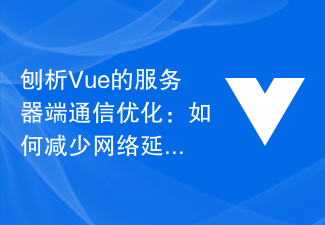
Analyzing Vue's server-side communication optimization: how to reduce network latency. In recent years, with the rapid development of the Internet, performance optimization of web applications has become an indispensable part of the design and development process. Among them, server-side communication optimization is one of the key factors to reduce network latency and improve user experience. This article will focus on the Vue framework and analyze how to optimize server-side communication in terms of reducing the number of HTTP requests, compressing files, and using cache to achieve faster loading speeds and better user experience. 1. Reduce HTTP requests
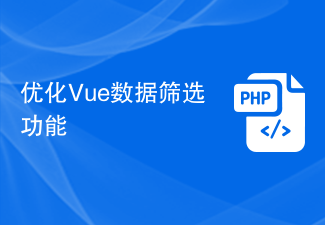
How to optimize the data filtering function in Vue development? In Vue development, data filtering is a common requirement. Whether it is displaying data to users or filtering data based on user selections, the data filtering function is an essential component. However, data filtering capabilities may face performance issues when processing large amounts of data or complex filtering criteria. This article will introduce some methods to optimize the data filtering function in Vue development to help developers improve application performance and user experience. Use computed properties for data filtering
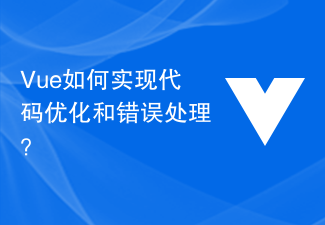
In the development process of Vue applications, in addition to realizing functions and user interaction experience, the performance and stability of the code are also very important. Therefore, in order to make Vue applications more efficient, stable, and easy to maintain, certain code optimization and error handling need to be performed. This article will introduce how Vue implements code optimization and error handling. 1. Optimize Vue applications. Use vue-cli to build applications. The Vue framework provides vue-cli scaffolding tools to quickly create Vue applications and components.
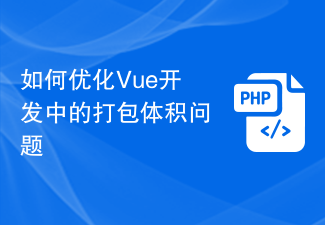
How to optimize the packaging volume problem in Vue development. With the continuous development of front-end technology, more and more developers choose to use Vue as the front-end framework to develop projects. Vue is easy to use, efficient and flexible, and is deeply loved by developers. However, in Vue development, the packaging size issue is a common challenge, especially in large projects. This article will introduce some methods to optimize the packaging volume problem in Vue development to help developers improve project performance and user experience. Modular system using ES6 modular ES6
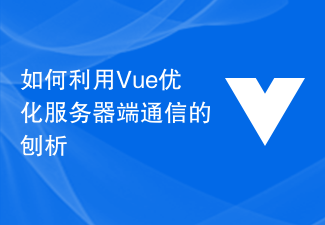
Introduction to how to use Vue to optimize server-side communication: As the complexity of front-end pages continues to increase, server-side communication has also become an important link. In order to improve performance and user experience, we need to optimize server-side communication. This article will introduce how to use the Vue framework to optimize server-side communication and provide some code examples. 1. Use Axios to send asynchronous requests. Axios is a Promise-based HTTP library that can send HTTP requests in browsers and Node.js.
