How to handle autofill and autocomplete in PHP forms
How to deal with autofill and autocomplete in PHP forms
With the development of the Internet, people are increasingly relying on autofill and autocomplete features to simplify their Operations on the Website. Implementing these functions in PHP forms is not complicated. This article will briefly introduce how to use PHP to handle auto-fill and auto-complete of forms.
Before we begin, we need to clarify what autofill and autocomplete are. Autofill refers to automatically filling in the fields in a form for users based on their previous input or history. For example, when a user enters an email address, possible email addresses are automatically filled in based on the user's previous input. Auto-complete refers to automatically providing possible options to the user during their input process. For example, when the user enters a city name, possible city names are automatically displayed for the user to choose.
First, we need a database to store autofill and autocomplete data. Let's take the city name as an example. First, create a database table named "city", which contains a field named "city_name" to store the city name.
CREATE TABLE city (
city_name VARCHAR(255) NOT NULL
);
Next, we need to prepare some city name data to populate the database. We can add data manually or import it using a CSV file.
INSERT INTO city (city_name) VALUES
('北京'), ('上海'), ('广州'), ('深圳'), ('杭州');
Now that we have the database and data ready, we can start processing the form. First, we need an input box to receive user input.
It should be noted that we put the autocomplete attribute of the input box Set to "off" to prevent the browser's default autocomplete function from interfering with our autocomplete function.
Next, we use AJAX to implement the auto-fill and auto-complete functions. After we enter text in the input box, we obtain the matching city name through AJAX and display it in the drop-down list.
<script><br>$ (document).ready(function(){</p><div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>$('#city').keyup(function(){
var query = $(this).val();
if(query != ''){
$.ajax({
url:"fetch.php",
method:"POST",
data:{query:query},
success:function(data){
$('#cityList').fadeIn();
$('#cityList').html(data);
}
});
}
});
$(document).on('click', 'li', function(){
$('#city').val($(this).text());
$('#cityList').fadeOut();
});</pre><div class="contentsignin">Copy after login</div></div><p>});<br></script>
In the above code, we use the jQuery library to simplify the use of AJAX . After the user enters text, we send the entered text to the "fetch.php" file for processing through AJAX, and display the returned city name in the drop-down list. After the user clicks on a city name, we fill the city name into the input box and hide the drop-down list.
So, how does the "fetch.php" file handle requests? Next, let's write the code for the "fetch.php" file.
//Connect to the database
$dsn = "mysql:host=localhost;dbname=test;charset=utf8";
$username = "root";
$password = "";
$options = array(
PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION, PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC
);
try{
$db = new PDO($dsn, $username, $password, $options);
}catch(PDOException $e){
die("连接数据库失败:" . $e->getMessage());
}
// Get the parameters of the AJAX request
$query = $_POST['query'] ?? '';
// Query the matching city name in the database
$sql = "SELECT * FROM city WHERE city_name LIKE :query";
$stmt = $db->prepare($sql);
$stmt->bindValue(':query', ' %'.$query.'%');
$stmt->execute();
$result = $stmt->fetchAll();
// Return the list of city names
if($stmt->rowCount() > 0){
foreach($result as $row){ echo "<li>". $row['city_name'] . "</li>"; }
}else{
echo "<li>无匹配城市</li>";
}
?>
in the above In the code, we first connect to the database. Then, we get the parameters of the AJAX request and use the LIKE statement to query the matching city name in the database. Finally, we return the query results to the front end.
At this point, we have completed the implementation of the auto-fill and auto-complete functions in the PHP form. Users only need to enter part of the city name, and the matching city name will be automatically obtained and displayed in the drop-down list. After the user selects a city name, it is automatically filled in the input box. In this way, we not only provide a convenient input method, but also improve the user experience.
In short, PHP provides powerful features to handle autofill and autocomplete in forms. Through reasonable database design and front-end technology, we can easily implement these functions and bring a better interactive experience to users. I hope this article has been helpful for you to understand and use autofill and autocomplete in PHP forms.
The above is the detailed content of How to handle autofill and autocomplete in PHP forms. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



How to implement auto-complete and auto-fill through Vue and ElementPlus Introduction: In modern web development, form auto-complete and auto-fill are an important functional requirement. It can improve user experience and reduce the tediousness and errors of repeated input. This article will introduce how to use Vue and ElementPlus to implement auto-complete and auto-fill functions, and provide corresponding code examples. 1. What is autocomplete and autofill? Autocomplete (Autocomplete): When the user enters a text
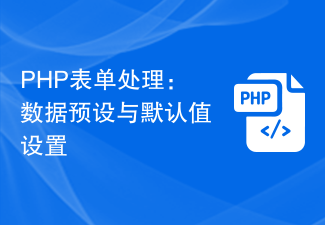
PHP form processing: data presets and default value settings When developing web applications, forms are an inevitable part. When a user submits a form, we need to process this data and act accordingly. This article will focus on how to handle presets and default value settings for form data in PHP. Data preset Data preset refers to setting default values for input fields in the form when the form is loaded. In this way, when users fill out the form, they can see that some fields already have default values, which is convenient for users to operate. In PHP, you can use HTML
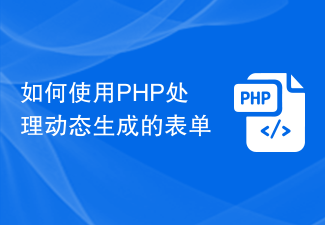
How to handle dynamically generated forms using PHP In web development, forms are one of the most common elements for interacting with users. In some cases, we may need to generate a form dynamically, changing the content and structure of the form according to the user's needs or options. PHP is a powerful back-end programming language that can help us process dynamically generated form data. This article will introduce how to use PHP to handle dynamically generated forms. First, we need to understand how to dynamically generate a form. In HTML, you can use PHP code to embed H
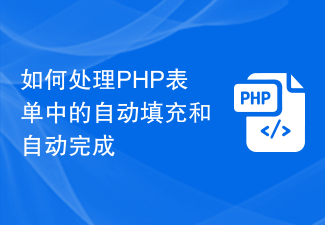
How to Handle Autofill and Autocomplete in PHP Forms As the Internet develops, people increasingly rely on autofill and autocomplete features to simplify their operations on the website. Implementing these functions in PHP forms is not complicated. This article will briefly introduce how to use PHP to handle auto-fill and auto-complete of forms. Before we begin, we need to clarify what autofill and autocomplete are. Autofill refers to automatically filling in the fields in a form for users based on their previous input or history. For example, when the user enters an email
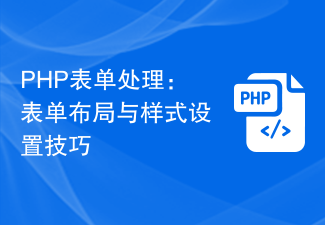
PHP form processing: form layout and styling skills Introduction: In web development, forms are one of the important components for interacting with users. The layout and style setting of the form will not only affect the user experience, but also directly affect the correct transmission and processing of data. This article will introduce some layout and styling techniques in PHP form processing and provide practical code examples. 1. Form layout skills: Use HTML and CSS for layout: In PHP, we can use HTML and CSS to layout the form.
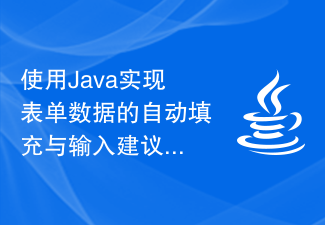
Using Java to implement automatic filling of form data and input suggestions In recent years, with the development of the Internet, filling in form data has become a part of our daily lives. However, filling in a large amount of form data often causes some trouble for users, especially when entering repeated data. In order to improve the user's filling efficiency and experience, we can use Java language to implement automatic filling of form data and input suggestions. This article will introduce how to use Java to implement this function and provide code examples for reference. First, we need
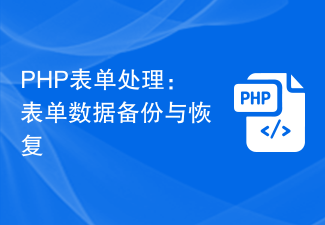
PHP form processing: Form data backup and recovery Introduction In the process of website development, forms are a very common way of interaction. Users submit data to the server for processing by filling in the form. However, sometimes users may lose form data due to network problems, browser crashes, or other unexpected situations, which will cause trouble to the user experience. Therefore, in order to improve the user experience, we can implement automatic backup and recovery functions of form data through PHP to ensure that the data filled in by users will not be lost. Form data backup when user is in table
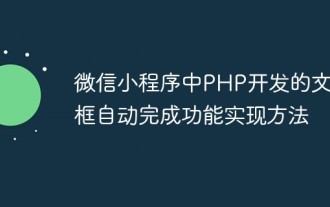
With the popularity of WeChat mini programs, various development needs are also increasing. Among them, the text box auto-complete function is one of the commonly used functions in mini programs. Although the WeChat applet provides some native components, some special needs still require secondary development. This article will introduce how to use PHP language to implement the automatic completion function of text boxes in WeChat applet. Preparation Before starting development, you need to prepare some basic environments and tools. First, you need to install the PHP environment. Secondly, you need to obtain your own Ap in the WeChat applet background
