


Interpret and apply namespace rules in PHP code specifications
Interpret and apply the namespace rules in PHP code specifications
When developing large projects, good code specifications play an important role in the maintainability and readability of the code. plays a vital role. The namespace rules in PHP code specifications can help us better organize and manage code.
1. The role of namespace
Namespace (Namespace) is a feature introduced by PHP starting from version 5.3. It is mainly used to solve problems when there are duplicate-named classes or functions in the code. Naming conflict issue. By using namespaces, we can divide the code according to functions or modules to avoid naming conflicts and improve the maintainability and reusability of the code.
2. Definition and use of namespace
In PHP, the namespace is defined using the keyword namespace. Here is an example:
namespace MyProject; class MyClass { // class code here } function myFunction() { // function code here }
In the above example, we defined a namespace named "MyProject" and defined a "MyClass" class and a "myFunction" function within the namespace. When using these codes elsewhere, you need to introduce the corresponding namespace first.
3. Namespace naming rules
In order to follow good programming habits and code specifications, we need to abide by some namespace naming rules. Here are some common rules:
- Namespace names should start with a capital letter and use camel case naming. For example: "MyProject".
- The name of the namespace should correspond to the file path where the code belongs. For example, the namespace "MyProject" should be defined in the "MyProject.php" file.
- If the namespace represents a class library or framework, it can be named using the root namespace and sub-namespaces. For example, if we develop a class library named "MyProject", we can use "MyProjectDatabase" as the namespace.
- Each level (hierarchy) in the namespace should be stored in a separate folder. For example, the namespace "MyProjectDatabase" can be stored in the "Database" folder.
4. Import and use of namespaces
When using code in other namespaces, we can import the corresponding namespace through the use keyword. The following is an example:
namespace MyApp; use MyProjectMyClass; use AnotherProjectAnotherClass as AliasedClass; $myObj = new MyClass(); $anotherObj = new AliasedClass();
In the above example, we first import the two namespaces "MyProjectMyClass" and "AnotherProjectAnotherClass", and then use the alias "AliasedClass" to access the "AnotherClass" class.
By using the import function of namespace, we can more conveniently use classes and functions in other namespaces in the code. At the same time, imported classes can be represented by full namespace paths or aliases.
5. Best practices for namespaces
- Avoid using the global namespace to import (that is, using the use keyword to import the root namespace). This can easily cause naming conflicts and namespace confusion.
- Define the namespace of the current file at the beginning of each file. This makes the code clearer and improves readability.
- Use meaningful namespaces. The name of a namespace should reflect the function or module of the code it contains.
- The structure of the namespace should be as concise and easy to understand as possible. Don't make nested namespaces so complex that they confuse other developers.
6. Conclusion
By complying with the namespace rules in the PHP code specification, we can better organize and manage the code and avoid naming conflicts and duplicate definitions. When using namespaces, we need to define reasonable namespace names and follow naming conventions. At the same time, use appropriate import methods to improve the readability and maintainability of the code. Good namespace specifications can make our code clearer, easier to understand, and easier to maintain.
The above is the interpretation and application of the namespace rules in the PHP code specification. I hope it can bring you some help in development. thanks for reading!
The above is the detailed content of Interpret and apply namespace rules in PHP code specifications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


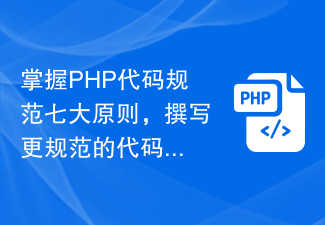
To understand the seven principles of PHP code specifications and write more standardized code, specific code examples are required. Introduction: PHP is a popular programming language that is widely used in the field of web development. Writing well-formed code is key to developing high-quality applications. This article will introduce the seven principles of PHP code specifications and provide specific code examples to help developers write more standardized PHP code. 1. Naming conventions Good naming conventions are the basis for writing standardized code. The following are several principles of naming conventions: Class names and interface names use camel case starting with an uppercase letter.
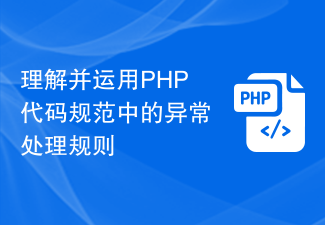
Understand and apply the exception handling rules in PHP code specifications. Exception handling is a very important part of programming. It can effectively help us find, locate and solve errors in the program. The PHP code specification provides a standard set of exception handling rules, which is very helpful for writing code that is readable, maintainable and reliable. This article describes these rules and illustrates them with code examples. 1. When to use exception handling Before understanding the exception handling rules, we must first clarify when to use exception handling. Exception handling should be used to handle
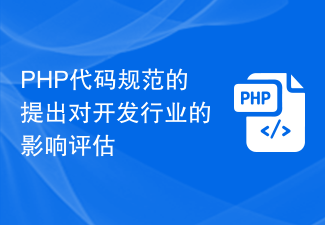
Assessment of the impact of the introduction of PHP code specifications on the development industry. With the continuous development of the software development industry, code specifications have become an important means to improve code quality, readability and maintainability. In the field of PHP development, the introduction of PHP code specifications has had a positive impact on the entire development industry. This article will evaluate the impact of the proposed PHP code specification on the development industry from several aspects, and illustrate it with code examples. Improve code quality. Code specifications can improve code quality through unified naming conventions, code structure and comment specifications.
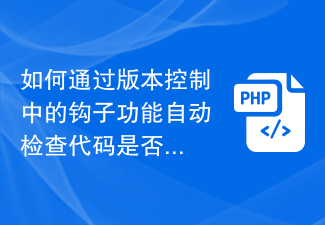
How to automatically check whether the code complies with the latest PHP code specifications through the hook function in version control? As team collaboration and development become increasingly common, the unification of code specifications has become particularly important. In PHP development, following the latest PHP code specifications can improve the readability and maintainability of the code, thereby improving the team's development efficiency. This article will introduce how to automatically check whether the code complies with the latest PHP code specifications through the hook function in version control, and provide corresponding code examples. 1. What is the hook function of version control version control
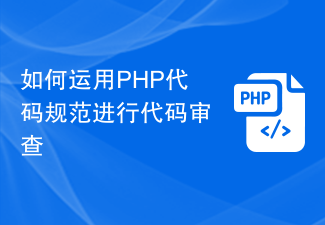
How to use PHP code specifications for code review Introduction: PHP is a widely used development language. Its flexibility and powerful functions make many developers love to use it to build websites and applications. However, due to the flexibility of PHP, it is easy to have problems with code irregularities and low quality. In order to ensure the readability, maintainability and scalability of the code, we need to use PHP code specifications for code review. This article will introduce some commonly used PHP code specifications and provide corresponding code examples. I hope you can conduct code review.
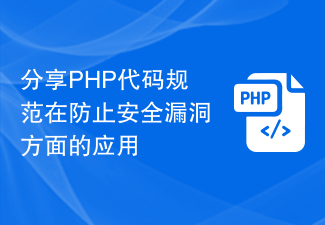
Introduction to the application of PHP code specifications in preventing security vulnerabilities: With the development of Internet applications, security issues have become an aspect that our developers must pay attention to. In web development, PHP is a widely used programming language and one of the main targets of hackers. In order to ensure that the developed applications are safe and reliable, it is not only necessary to pay attention to the security configuration of the server environment, but also to pay attention to security from the code level. In this article, I will focus on the application of PHP code specifications in preventing security vulnerabilities and provide a
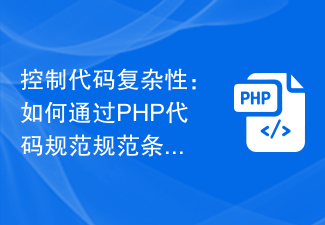
Controlling code complexity: How to standardize conditional judgments through PHP code specifications Introduction: When writing code, an important goal is to keep the code readable and maintainable, and conditional judgments are one of the most common parts of the code. Reasonable specification and optimized condition judgment can reduce the complexity of the code and improve the readability and maintainability of the code. This article will introduce some best practices for PHP code specification to help you better standardize conditional judgments and reduce code complexity. Use explicit Boolean values in conditional judgments. Using explicit Boolean values will cause the code to
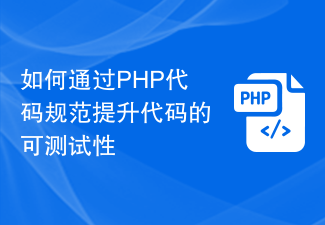
How to improve code testability through PHP code specifications Summary: It is very important for developers to write testable code. This article will introduce how to improve the testability of your code by following some PHP coding standards, with code examples. Introduction: In modern software development, testability has become a very important element. Testable code enables faster debugging and fixing problems, while also delivering higher quality software. In PHP development, we can improve
