How to use Vue form processing to implement complex form layout
How to use Vue form processing to implement complex form layout
In developing web applications, forms are a very common element. In some cases, we need to implement some more complex form layouts to meet business needs. Using Vue.js as the front-end framework, we can easily handle complex form layouts and implement two-way binding of data.
In this article, we will introduce how to use Vue form processing to implement complex form layout, and attach corresponding code examples.
- Using Vue componentization idea
When dealing with complex form layout, each component of the form can be split into different Vue components, each component is responsible for the corresponding function. The advantage of this is that it can improve the maintainability and reusability of the code. For example, we can split the form header, form body and form tail into different Vue components.
The following is a sample code:
<template> <div> <FormHeader></FormHeader> <FormBody></FormBody> <FormFooter></FormFooter> </div> </template> <script> import FormHeader from './components/FormHeader.vue'; import FormBody from './components/FormBody.vue'; import FormFooter from './components/FormFooter.vue'; export default { components: { FormHeader, FormBody, FormFooter } } </script>
- Using Vue form components
Vue.js provides a series of convenient form components, such as< input>
,<select>
,<textarea>
, etc., can easily construct form input elements.
The following is a sample code:
<template> <div> <label for="name">姓名:</label> <input type="text" id="name" v-model="formData.name"> <label for="age">年龄:</label> <input type="number" id="age" v-model="formData.age"> <label for="gender">性别:</label> <select id="gender" v-model="formData.gender"> <option value="male">男</option> <option value="female">女</option> </select> </div> </template> <script> export default { data() { return { formData: { name: '', age: '', gender: '' } } } } </script>
The above code demonstrates how to use the Vue form component to build a simple form input element and implement two-way binding of data. Through the v-model
directive, the input element is bound to the form data. When the value of the input element changes, the form data will be updated accordingly.
- Form Validation
When dealing with complex form layouts, form validation is an essential link. Vue.js provides some built-in form validation instructions, which can easily perform form validation.
The following is a sample code:
<template> <div> <label for="name">姓名:</label> <input type="text" id="name" v-model="formData.name" required> <span v-if="!formData.name">姓名不能为空</span> <label for="age">年龄:</label> <input type="number" id="age" v-model="formData.age" min="18" max="60"> <span v-if="formData.age < 18 || formData.age > 60">年龄必须在18岁到60岁之间</span> <label for="gender">性别:</label> <select id="gender" v-model="formData.gender" required> <option value="">请选择</option> <option value="male">男</option> <option value="female">女</option> </select> <span v-if="!formData.gender">性别不能为空</span> </div> </template> <script> export default { data() { return { formData: { name: '', age: '', gender: '' } } } } </script>
The above code demonstrates how to perform simple form validation in a Vue form. Form input can be restricted by adding corresponding validation instructions, such as required
, min
, and max
, and passing v-if
The command matches the conditional judgment and displays the corresponding error message.
Summary:
Using Vue form processing to implement complex form layout can greatly improve development efficiency and code maintainability. Through Vue componentization ideas, Vue form components and form validation, we can easily build a powerful and complex form layout. I hope this article will help you deal with complex form layout in Vue development!
The above is the detailed content of How to use Vue form processing to implement complex form layout. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


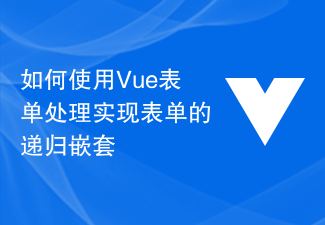
How to use Vue form processing to implement recursive nesting of forms Introduction: As the complexity of front-end data processing and form processing continues to increase, we need a flexible way to handle complex forms. As a popular JavaScript framework, Vue provides us with many powerful tools and features to handle recursive nesting of forms. This article will introduce how to use Vue to handle such complex forms, and attach code examples. 1. Recursive nesting of forms In some scenarios, we may need to deal with recursive nesting.

How to dynamically bind and update form data in Vue With the continuous development of front-end development, forms are an interactive element that we often use. In Vue, dynamic binding and updating of forms is a common requirement. This article will introduce how to dynamically bind and update form data in Vue, and provide specific code examples. 1. Dynamic binding of form data Vue provides the v-model instruction to achieve two-way binding of form data. Through the v-model directive, we can compare the value of the form element with the Vue instance
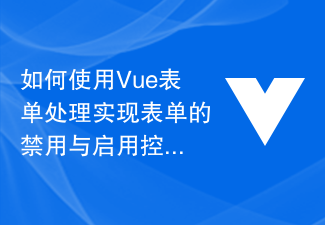
How to use Vue form processing to implement disabling and enabling control of forms. In web development, forms are one of the indispensable components. Sometimes, we need to control the disabled and enabled status of a form based on specific conditions. Vue provides a concise and effective way to handle this situation. This article will introduce in detail how to use Vue to implement disabling and enabling control of the form. First, we need to create a basic Vue instance and a form. Here is a basic HTML and Vue code example: <divid=&
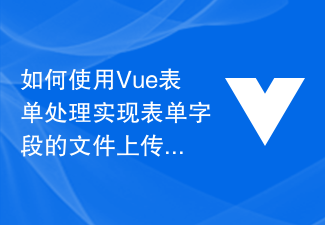
How to use Vue form processing to implement file upload of form fields Preface: In web applications, file upload is a very common requirement. Sometimes, we need users to upload files as part of the form fields, such as uploading user avatars, uploading pictures in comments, etc. It is very simple to use Vue to process and implement file uploads of form fields. In this article, we will introduce how to implement file upload using Vue form processing and provide code examples. Create a Vue component First, we need to create a Vue for file upload
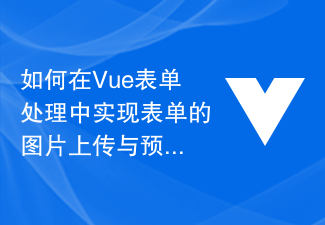
How to implement form image upload and preview in Vue form processing Introduction: In modern web applications, form processing is a very common requirement. One common requirement is to allow users to upload images and preview them in a form. As a front-end framework, Vue.js provides us with a wealth of tools and methods to achieve this requirement. In this article, I will show you how to implement image upload and preview functions in Vue form processing. Step 1: Define Vue components First, we need to define a Vue group
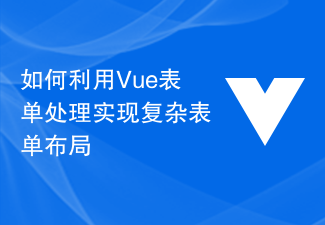
How to use Vue form processing to implement complex form layout In developing web applications, forms are a very common element. In some cases, we need to implement some more complex form layouts to meet business needs. Using Vue.js as the front-end framework, we can easily handle complex form layouts and implement two-way binding of data. In this article, we will introduce how to use Vue form processing to implement complex form layout, and attach corresponding code examples. Use Vue componentization ideas to process complex forms

How to use Vue form processing to implement form state management. In web development, forms are an important part of how users interact with web pages. In the Vue framework, user experience and development efficiency can be improved by properly handling the state of the form. This article will explore how to use Vue form processing to implement form state management and illustrate it with code examples. Using two-way binding in Vue Vue provides a two-way binding method, which can easily achieve synchronous updates of form elements and data. Using v-mod on form elements
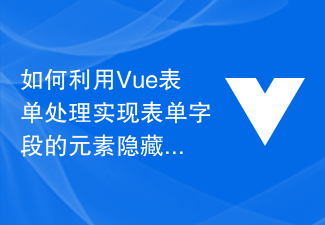
How to use Vue form processing to hide and display elements of form fields Introduction: In front-end development, processing forms is a common and important task. Sometimes we need to hide and display form field elements based on user selection or other conditions. It is very simple to implement this function in Vue. This article will introduce how to use Vue form processing to hide and display elements of form fields, and attach code examples for reference. 1. Use the v-if instruction to hide and display the v-if instruction in Vue according to
