


Using Laravel for queue processing and task scheduling: improving application performance
Using Laravel for queue processing and task scheduling: improving application performance
Introduction:
With the development of applications, we are often faced with processing a large number of tasks and requested challenges. In order to improve application performance and responsiveness, the Laravel framework provides a powerful queue processing and task scheduling system. This article will introduce how to use Laravel's queue function to handle asynchronous tasks and schedule recurring tasks to improve application performance and stability.
1. Introduction to Laravel Queue Function
Laravel provides a queue service with good abstraction, which can add tasks that need to be executed asynchronously to the queue, and then process them by background processes or queue workers. This asynchronous task execution mode is called "queue", which can effectively separate some non-real-time or time-consuming tasks from the main request process, allowing the application to respond to requests faster.
Advantages of Laravel queue:
- Asynchronous execution: After adding a task to the queue, a response can be returned to the user immediately, and the task will be executed asynchronously in the background.
- Improve concurrency: Since tasks are put into the queue, multiple tasks can be processed at the same time, which improves the concurrent processing capabilities of the application.
- Fault tolerance processing: When the task execution fails, Laravel will put the task back into the queue to ensure that the task can be executed successfully.
- Safe and stable: By placing tasks into the queue, some sensitive operations or time-consuming operations can be avoided from blocking the normal request process.
- Microservice support: Queue services can be decoupled from other microservices to improve application scalability.
2. Configuring Laravel queue
- Configuring the driver
In Laravel’s configuration fileconfig/queue.php
, you can configure the queue Drivers. Laravel supports multiple types of queue drivers, such as database, redis, beanstalkd, etc.
Example configuration:
'default' => env('QUEUE_CONNECTION', 'redis'), 'connections' => [ // Redis 驱动配置 'redis' => [ 'driver' => 'redis', 'connection' => 'default', 'queue' => env('Redis_QUEUE', 'default'), 'retry_after' => 90, 'block_for' => null, ], ],
- Start the queue worker
In Laravel, queue tasks need to be executed by a queue worker (Queue Worker). You can use Laravel's own Artisan commandphp artisan queue:work
to start the queue worker and configure it as needed.
Example command:
php artisan queue:work --queue=queue-name --tries=3
This command will start a queue worker, listen to the specified queue (queue-name
), and when the task execution fails Maximum of 3 attempts.
3. Use Laravel queue to process tasks
- Create and distribute tasks
First, we need to create a task class. You can use Laravel's own Artisan commandphp artisan make:job
to create a new queue task class.
Example task class:
<?php namespace AppJobs; use IlluminateBusQueueable; use IlluminateContractsQueueShouldQueue; use IlluminateFoundationBusDispatchable; use IlluminateQueueInteractsWithQueue; use IlluminateQueueSerializesModels; class ProcessPodcast implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; protected $podcast; /** * Create a new job instance. * * @param Podcast $podcast * @return void */ public function __construct(Podcast $podcast) { $this->podcast = $podcast; } /** * Execute the job. * * @return void */ public function handle() { // 处理任务逻辑 } }
By implementing the ShouldQueue
interface, the task class will become a task class that can be processed by the queue.
Then, we can use the queue's dispatch
method to distribute the task to the queue and wait for asynchronous processing.
Example distribution task:
use AppJobsProcessPodcast; ProcessPodcast::dispatch($podcast);
- Listen to the queue and execute the task
When the queue worker starts, it will listen to the specified queue. Once a new task enters the queue, Thehandle
method of the task will be executed.
In the handle
method of the task, write the logic code that needs to be executed asynchronously.
Example task processing logic:
public function handle() { // 执行异步任务 // ... // 执行完成后,任务将从队列中移除 }
Through the above steps, we can add tasks that need to be executed asynchronously to the queue, and have them processed and executed by queue workers.
4. Use Laravel task scheduling function
In addition to the queue processing function, Laravel also provides a task scheduling function, which can execute a task regularly or repeat a task a specified number of times.
- Create task scheduling
Use Laravel’s own Artisan commandphp artisan make:command
to create a task scheduling class.
Example task scheduling class:
<?php namespace AppConsoleCommands; use IlluminateConsoleCommand; class SendEmails extends Command { /** * The name and signature of the console command. * * @var string */ protected $signature = 'emails:send'; /** * The console command description. * * @var string */ protected $description = 'Send reminder emails to all users'; /** * Execute the console command. * * @return mixed */ public function handle() { // 任务调度逻辑 } }
- Configuring task scheduling
In Laravel’s configuration fileapp/Console/Kernel.php
, You can configure task scheduling and scheduling frequency to be executed periodically.
Example configuration:
protected $commands = [ CommandsSendEmails::class, ]; protected function schedule(Schedule $schedule) { $schedule->command('emails:send') ->dailyAt('01:00'); }
The above configuration indicates that the emails:send
task will be executed at 1 am every day.
- Start the task scheduler
Add a command to regularly execute the Laravel task scheduler in the server's crontab so that scheduled tasks can be executed regularly.
Example crontab command:
* * * * * cd /path-to-your-project && php artisan schedule:run >> /dev/null 2>&1
Through the above steps, we can execute a task regularly or repeat a task a specified number of times, thereby improving the automation and stability of the application.
Conclusion:
Through Laravel's queue processing and task scheduling functions, we can separate some time-consuming tasks and repetitive tasks from the main request process, improving the performance and responsiveness of the application. . At the same time, it also provides exception handling and fault-tolerance processing mechanisms to ensure that tasks can be successfully executed and ensure application stability. I hope the content of this article can help you better use Laravel to improve the performance and stability of your application.
The above is the detailed content of Using Laravel for queue processing and task scheduling: improving application performance. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
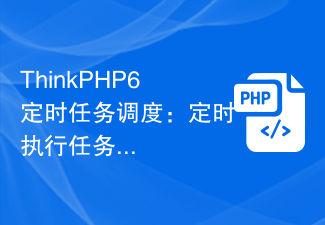
ThinkPHP6 scheduled task scheduling: scheduled task execution 1. Introduction In the process of web application development, we often encounter situations where certain repetitive tasks need to be executed regularly. ThinkPHP6 provides a powerful scheduled task scheduling function, which can easily meet the needs of scheduled tasks. This article will introduce how to use scheduled task scheduling in ThinkPHP6, and provide some code examples to help understand. 2. Configure scheduled tasks, create scheduled task files, and create a comman in the app directory of the project.
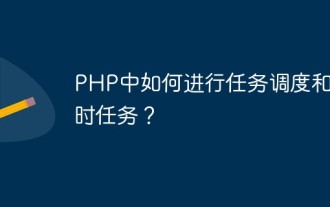
In web development, many websites and applications need to perform certain tasks regularly, such as cleaning up junk data, sending emails, etc. In order to automate these tasks, developers need to implement task scheduling and timed task functions. This article will introduce how to implement task scheduling and timed tasks in PHP, as well as some commonly used third-party libraries and tools. 1. Task Scheduling Task scheduling refers to executing certain tasks according to specified times or events. In PHP, cron timer or similar mechanism can be used to implement task scheduling. Typically, task scheduling

SpringBoot is a very popular Java development framework. It not only has the advantage of rapid development, but also has many built-in practical functions. Among them, task scheduling and scheduled tasks are one of its commonly used functions. This article will explore SpringBoot's task scheduling and timing task implementation methods. 1. Introduction to SpringBoot task scheduling SpringBoot task scheduling (TaskScheduling) refers to executing some special tasks at a specific point in time or under certain conditions.
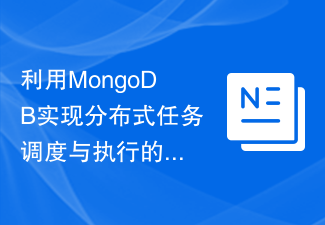
MongoDB is an open source NoSQL database with high performance, scalability and flexibility. In distributed systems, task scheduling and execution are a key issue. By utilizing the characteristics of MongoDB, distributed task scheduling and execution solutions can be realized. 1. Requirements Analysis for Distributed Task Scheduling In a distributed system, task scheduling is the process of allocating tasks to different nodes for execution. Common task scheduling requirements include: 1. Task request distribution: Send task requests to available execution nodes.
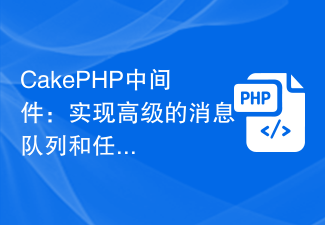
CakePHP Middleware: Implementing Advanced Message Queuing and Task Scheduling With the rapid development of the Internet, we are faced with the challenge of handling a large number of concurrent requests and task scheduling. The traditional request response model can no longer meet our needs. In order to better solve this problem, CakePHP introduces the concept of middleware and provides rich functions to implement advanced message queue and task scheduling. Middleware is one of the core components of CakePHP applications and can add custom logic to the request processing flow. through middleware
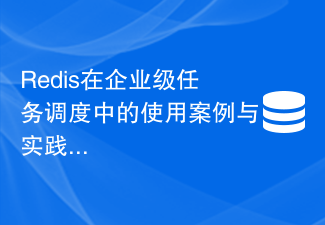
With the complexity of enterprise-level applications and the expansion of business scale, task scheduling has become an indispensable and important task. The ensuing problem is how to manage and schedule a large number of tasks, coordinate different business processes, and ensure the stability and reliability of the system. In order to solve this problem, Redis, as a high-performance data structure database, is used by more and more enterprises as the central node for task scheduling to manage and schedule increasingly complex task processes. This article takes the use cases and practices of Redis in enterprise-level task scheduling as an example.
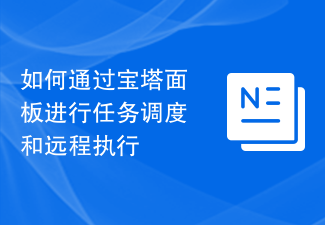
More and more personal websites and small businesses are choosing to use Pagoda Panel for server management. As a well-known server control panel in China, Pagoda Panel has many practical functions, including support for task scheduling and remote execution. These features can simplify the server management process to a great extent and improve management efficiency. This article will introduce how to perform task scheduling and remote execution through the Pagoda Panel. First, we need to understand what task scheduling and remote execution are. Task scheduling refers to executing specified tasks at a specific time, such as

Using Redis to implement distributed task scheduling With the expansion of business and the development of the system, many businesses need to implement distributed task scheduling to ensure that tasks can be executed on multiple nodes at the same time, thereby improving the stability and availability of the system. As a high-performance memory data storage product, Redis has the characteristics of distribution, high availability, and high performance, and is very suitable for implementing distributed task scheduling. This article will introduce how to use Redis to implement distributed task scheduling and provide corresponding code examples. 1. Redis base
