


How to combine jsmind in a Vue project to achieve real-time sharing and collaborative editing of mind maps?
How to combine jsmind in a Vue project to achieve real-time sharing and collaborative editing of mind maps?
Mind mapping is a very effective tool that can help us organize and express our thinking. With the popularity of Vue, it has become possible to combine Vue and the jsmind library to achieve real-time sharing and collaborative editing of mind maps. In this article, we will introduce how to use the jsmind library in a Vue project to create mind maps and achieve real-time sharing and collaborative editing.
First, we need to install the jsmind library in the Vue project. You can use npm or yarn to install jsmind:
npm install jsmind --save
or
yarn add jsmind
After the installation is complete, we need to introduce the jsmind library into the Vue component.
import jsMind from 'jsmind'; import 'jsmind/style/jsmind-default.css';
Next, we need to initialize jsmind in Vue's life cycle function and create a mind map.
mounted() { const jsmindContainer = this.$refs.jsmindContainer; const mindMap = { "meta": { "name": "思维导图", "author": "你的名字", "version": "1.0" }, "format": "node_array", "data": [ {"id":"root","isroot":true,"topic":"主题"} ] }; this.jsMindInstance = new jsMind(jsmindContainer, mindMap); },
In the above code, we first obtain the container element jsmindContainer
, and then create a jsmind instance based on the mind map data we defined.
Now, we have successfully created a mind map. Next, let’s implement real-time sharing and collaborative editing.
In order to achieve real-time sharing and collaborative editing, we need to use WebSocket to establish a real-time communication connection. Assume that we have set up a WebSocket server and can connect to the server through ws://localhost:8080
.
In the Vue component, we can use the vue-native-websocket
library to initialize the WebSocket connection.
First, we need to install the vue-native-websocket
library:
npm install vue-native-websocket --save
or
yarn add vue-native-websocket
Next, in Vue’s main. In the js
file, we need to introduce the vue-native-websocket
library and configure the WebSocket connection.
import VueNativeSock from 'vue-native-websocket'; Vue.use(VueNativeSock, 'ws://localhost:8080', { format: 'json', reconnection: true, reconnectionAttempts: 5, reconnectionDelay: 3000 });
In the above code, we initialize a WebSocket connection and bind it to VueNativeSock
. At the same time, we also specified the server's address and some connection configuration.
Next, in the Vue component, we can use this.$socket
to access the WebSocket connection for real-time sharing and collaborative editing.
methods: { handleMindMapUpdate(data) { this.jsMindInstance.show(data); } }, sockets: { mindMapUpdate(data) { this.handleMindMapUpdate(data); } },
In the above code, we define a mindMapUpdate
event. When the server sends the event, we will call the handleMindMapUpdate
method to update the mind map.
The complete Vue component code is as follows:
<template> <div ref="jsmindContainer"></div> </template> <script> import jsMind from 'jsmind'; import 'jsmind/style/jsmind-default.css'; export default { mounted() { const jsmindContainer = this.$refs.jsmindContainer; const mindMap = { "meta": { "name": "思维导图", "author": "你的名字", "version": "1.0" }, "format": "node_array", "data": [ {"id":"root","isroot":true,"topic":"主题"} ] }; this.jsMindInstance = new jsMind(jsmindContainer, mindMap); }, methods: { handleMindMapUpdate(data) { this.jsMindInstance.show(data); } }, sockets: { mindMapUpdate(data) { this.handleMindMapUpdate(data); } } }; </script>
In the above code, we pass the container element div
of the mind map through ref
The property is bound to jsmindContainer
and a jsmind instance is created in the mounted
life cycle function.
Finally, we need to implement the functions of real-time sharing and collaborative editing of mind maps in the WebSocket server. When a new mind map data is received, we need to broadcast it to all clients connected to the server.
The following is a sample code for a simple Node.js WebSocket server:
const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', (ws) => { ws.on('message', (data) => { // 接收到新的思维导图数据 // 广播给所有连接到服务器的客户端 wss.clients.forEach((client) => { if (client.readyState === WebSocket.OPEN) { client.send(data); } }); }); });
In the above code, we iterate through all the clients connected to the WebSocket server and when new ideas are received When mapping data, send it to all clients.
Through the above code examples, we can combine jsmind in the Vue project to realize the real-time sharing and collaborative editing functions of mind maps. Real-time communication through WebSocket allows multiple users to edit and view the same mind map at the same time, greatly improving team collaboration efficiency.
The above is the detailed content of How to combine jsmind in a Vue project to achieve real-time sharing and collaborative editing of mind maps?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


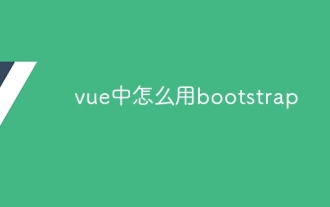
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
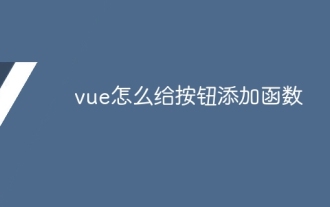
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
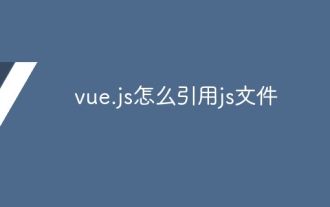
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
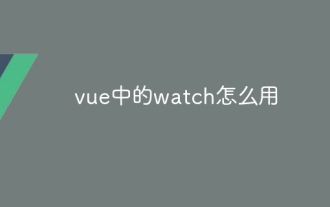
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
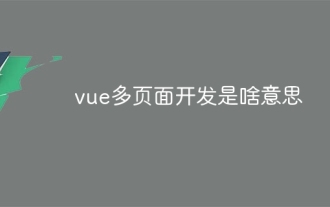
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
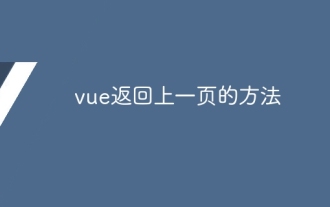
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
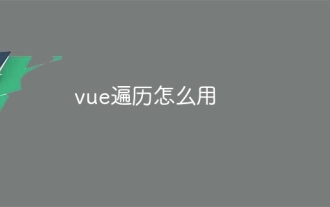
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
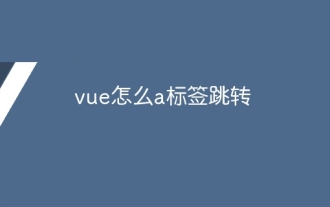
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
