


RESTful API Development in Laravel: Building Scalable and Maintainable Services
RESTful API Development in Laravel: Building Extensible and Maintainable Services
Introduction:
In web development, RESTful API has become the One of the main methods of maintainable services. As a powerful PHP development framework, Laravel provides a wealth of tools and functions to simplify the development process of RESTful APIs. This article will introduce how to use Laravel to build an extensible and maintainable RESTful API, and provide some code examples to help readers understand better.
1. Design routing:
In Laravel, routing is the mapping relationship between URL and corresponding controller method. In the design of RESTful API, we usually follow a set of standardized URL paths to express different states and operations of resources. For example, for user resources, we can use the following URL path:
- GET /users: Get all users
- GET /users/{id}: Get details of a specific id user
- POST /users: Create a new user
- PUT /users/{id}: Update the information of a specific id user
- DELETE /users/{id}: Delete a specific user The user with the id
In Laravel, you can use the following code example to define the route:
Route::get('users', 'UserController@index'); Route::get('users/{id}', 'UserController@show'); Route::post('users', 'UserController@store'); Route::put('users/{id}', 'UserController@update'); Route::delete('users/{id}', 'UserController@destroy');
2. Write the controller method:
In Laravel, the controller is the handler Where the business logic is. Each API request will correspond to a controller method. The following is sample code in UserController:
use AppModelsUser; use IlluminateHttpRequest; class UserController extends Controller { public function index() { $users = User::all(); return response()->json($users); } public function show($id) { $user = User::find($id); return response()->json($user); } public function store(Request $request) { $user = User::create($request->all()); return response()->json($user, 201); } public function update(Request $request, $id) { $user = User::findOrFail($id); $user->update($request->all()); return response()->json($user); } public function destroy($id) { User::destroy($id); return response()->json(null, 204); } }
The above code sample introduces several commonly used controller methods. For example, the index() method is used to obtain all users, the show() method is used to obtain user information with a specific id, the store() method is used to create a new user, the update() method is used to update the information of a specific id user, and destroy () method is used to delete users with specific ids.
3. Data verification:
In RESTful API development, data verification is a very important part. Laravel provides powerful validation features to easily validate incoming request data. The following is a sample code:
public function store(Request $request) { $validatedData = $request->validate([ 'name' => 'required', 'email' => 'required|unique:users', 'password' => 'required', ]); $user = User::create($validatedData); return response()->json($user, 201); }
The validate() method in the above code example will verify the data in the request and return the verified data. In this example, we verify that the name, email, and password fields are required, and ensure that the email field is unique in the users table.
4. Authentication and authorization:
When building a RESTful API, authentication and authorization are inevitable issues. Laravel provides us with a simple and flexible authentication and authorization mechanism. The following is a sample code:
use IlluminateSupportFacadesAuth; // 登录接口 public function login(Request $request) { $credentials = $request->only('email', 'password'); if (Auth::attempt($credentials)) { $user = Auth::user(); $token = $user->createToken('API Token')->accessToken; return response()->json(['access_token' => $token]); } else { return response()->json(['error' => 'Unauthorized'], 401); } } // 需要认证的接口 public function secureMethod() { $user = Auth::user(); return response()->json($user); }
In the above sample code, the login() method is used for login authentication and returns an access token for authorization in subsequent requests. The secureMethod() method is used for interfaces that only allow authorized users to access.
Conclusion:
This article explains how to use Laravel to build a scalable and maintainable RESTful API. By designing routes, writing controller methods, data validation, and authentication and authorization, we can easily build efficient and secure API services. I hope this article will be helpful to readers in RESTful API development.
The above is a Chinese article within 1500 words, titled: RESTful API development in Laravel: Building scalable and maintainable services.
The above is the detailed content of RESTful API Development in Laravel: Building Scalable and Maintainable Services. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


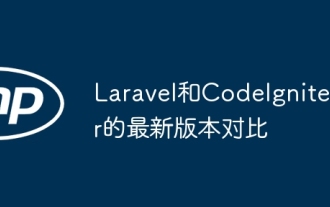
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
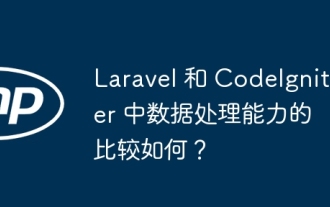
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
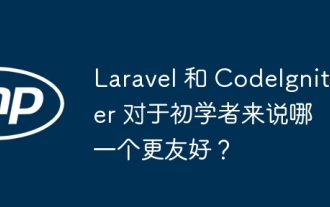
For beginners, CodeIgniter has a gentler learning curve and fewer features, but covers basic needs. Laravel offers a wider feature set but has a slightly steeper learning curve. In terms of performance, both Laravel and CodeIgniter perform well. Laravel has more extensive documentation and active community support, while CodeIgniter is simpler, lightweight, and has strong security features. In the practical case of building a blogging application, Laravel's EloquentORM simplifies data manipulation, while CodeIgniter requires more manual configuration.
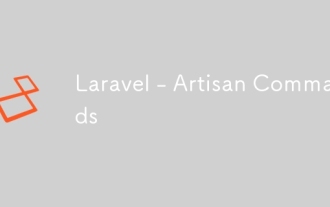
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
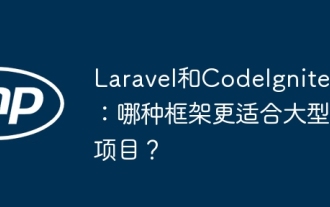
When choosing a framework for large projects, Laravel and CodeIgniter each have their own advantages. Laravel is designed for enterprise-level applications, offering modular design, dependency injection, and a powerful feature set. CodeIgniter is a lightweight framework more suitable for small to medium-sized projects, emphasizing speed and ease of use. For large projects with complex requirements and a large number of users, Laravel's power and scalability are more suitable. For simple projects or situations with limited resources, CodeIgniter's lightweight and rapid development capabilities are more ideal.
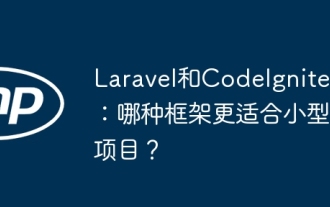
For small projects, Laravel is suitable for larger projects that require strong functionality and security. CodeIgniter is suitable for very small projects that require lightweight and ease of use.
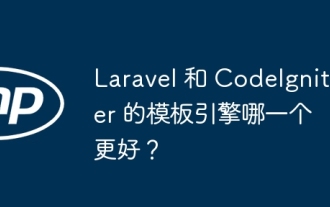
Comparing Laravel's Blade and CodeIgniter's Twig template engine, choose based on project needs and personal preferences: Blade is based on MVC syntax, which encourages good code organization and template inheritance. Twig is a third-party library that provides flexible syntax, powerful filters, extended support, and security sandboxing.
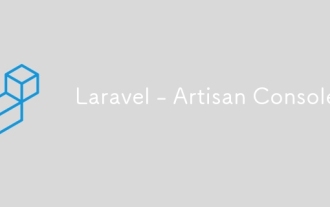
Laravel - Artisan Console - Laravel framework provides three primary tools for interaction through command-line namely: Artisan, Ticker and REPL. This chapter explains about Artisan in detail.
