File Uploading and Processing in Laravel: Managing User Uploaded Files
File Uploading and Processing in Laravel: Managing User Uploaded Files
Introduction:
File uploading is a very common feature in modern web applications need. In the Laravel framework, file uploading and processing becomes very simple and efficient. This article will introduce how to manage user-uploaded files in Laravel, including verification, storage, processing, and display of file uploads.
1. File upload
File upload refers to uploading files from the client to the server. In Laravel, file uploads are very easy to handle. First, we need to create a form for file upload and specify the enctype attribute of the form as "multipart/form-data" to support file upload.
Code example 1: File upload form
<form action="/upload" method="post" enctype="multipart/form-data"> @csrf <input type="file" name="file" id="file"> <input type="submit" value="上传"> </form>
On the server side, we can use the store
method to save the uploaded file to the specified directory. The store
method will automatically handle the generation of file names and paths for us, as well as the uniqueness of files to ensure that no file name conflicts occur.
Code example 2: File upload processing
public function upload(Request $request) { $file = $request->file('file'); if ($file->isValid()) { $path = $file->store('uploads'); // 保存成功,返回文件路径 return $path; } else { // 文件上传失败 return '文件上传失败'; } }
2. File storage
In Laravel, file storage is managed on disk. Laravel provides two file system disks by default: local disk and cloud storage disk.
- Local disk
Local disk refers to storing files in the local file system of the server. By default, Laravel uses thelocal
driver to operate on local disks. - Cloud Storage Disk
Cloud storage disk refers to storing files on cloud platforms, such as Amazon S3, Rackspace, etc. Laravel supports simple configuration via configuration files to use cloud storage disks.
Code Example 3: File Storage Configuration
// config/filesystems.php 'disks' => [ ... 'local' => [ 'driver' => 'local', 'root' => storage_path('app'), ], 's3' => [ 'driver' => 's3', 'key' => env('AWS_ACCESS_KEY_ID'), 'secret' => env('AWS_SECRET_ACCESS_KEY'), 'region' => env('AWS_DEFAULT_REGION'), 'bucket' => env('AWS_BUCKET'), ], ... ],
3. File Processing
In Laravel, we can perform various processing operations on uploaded files, such as resizing, Generate thumbnails, watermarks, etc. Laravel provides the Intervention Image
library to facilitate us to process images.
First, we need to install the Intervention Image library.
Code example 4: Install the Intervention Image library
composer require intervention/image
Then, we can use the Intervention Image library method in the file upload processing code for file processing.
Code example 5: File processing example (generating thumbnails)
public function upload(Request $request) { $file = $request->file('file'); if ($file->isValid()) { // 保存原图 $path = $file->store('uploads'); // 生成缩略图 $thumbnail = Image::make($file)->resize(200, 200)->save('uploads/thumbnails/'.$file->hashName()); // 保存成功,返回文件路径和缩略图路径 return [ 'path' => $path, 'thumbnail' => $thumbnail->path(), ]; } else { // 文件上传失败 return '文件上传失败'; } }
4. File display
In Laravel, we can use the path of the file to display the file. In the view, we can use the asset
function to generate the URL of the file and then display it in the web page.
Code Example 6: File Display
<img src="{{ asset($path) }}" alt="文件">
Conclusion:
By using the Laravel framework, we can easily manage files uploaded by users. This article describes methods for verifying, storing, processing, and displaying file uploads, and provides corresponding code examples. Hope this helps readers when dealing with file uploading and management in Laravel.
The above is the detailed content of File Uploading and Processing in Laravel: Managing User Uploaded Files. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


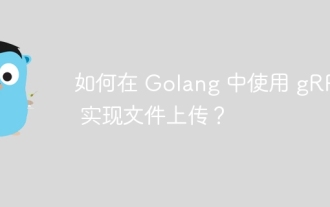
How to implement file upload using gRPC? Create supporting service definitions, including request and response messages. On the client, the file to be uploaded is opened and split into chunks, then streamed to the server via a gRPC stream. On the server side, file chunks are received and stored into a file. The server sends a response after the file upload is completed to indicate whether the upload was successful.
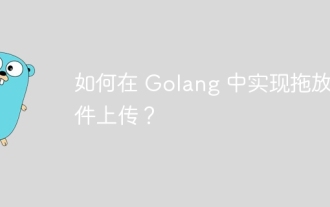
How to implement drag and drop file upload in Golang? Enable middleware; handle file upload requests; create HTML code for the drag and drop area; add JavaScript code for handling drag and drop events.
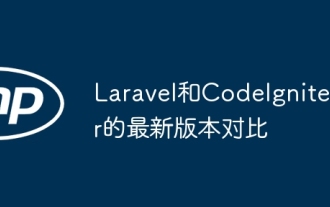
The latest versions of Laravel 9 and CodeIgniter 4 provide updated features and improvements. Laravel9 adopts MVC architecture and provides functions such as database migration, authentication and template engine. CodeIgniter4 uses HMVC architecture to provide routing, ORM and caching. In terms of performance, Laravel9's service provider-based design pattern and CodeIgniter4's lightweight framework give it excellent performance. In practical applications, Laravel9 is suitable for complex projects that require flexibility and powerful functions, while CodeIgniter4 is suitable for rapid development and small applications.
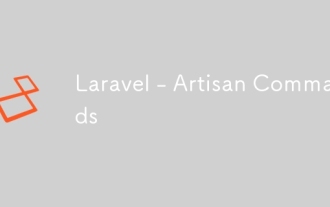
Laravel - Artisan Commands - Laravel 5.7 comes with new way of treating and testing new commands. It includes a new feature of testing artisan commands and the demonstration is mentioned below ?
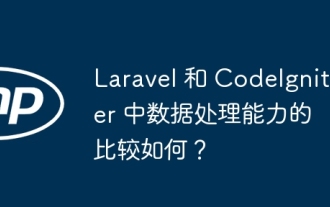
Compare the data processing capabilities of Laravel and CodeIgniter: ORM: Laravel uses EloquentORM, which provides class-object relational mapping, while CodeIgniter uses ActiveRecord to represent the database model as a subclass of PHP classes. Query builder: Laravel has a flexible chained query API, while CodeIgniter’s query builder is simpler and array-based. Data validation: Laravel provides a Validator class that supports custom validation rules, while CodeIgniter has less built-in validation functions and requires manual coding of custom rules. Practical case: User registration example shows Lar
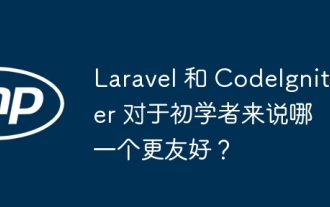
For beginners, CodeIgniter has a gentler learning curve and fewer features, but covers basic needs. Laravel offers a wider feature set but has a slightly steeper learning curve. In terms of performance, both Laravel and CodeIgniter perform well. Laravel has more extensive documentation and active community support, while CodeIgniter is simpler, lightweight, and has strong security features. In the practical case of building a blogging application, Laravel's EloquentORM simplifies data manipulation, while CodeIgniter requires more manual configuration.
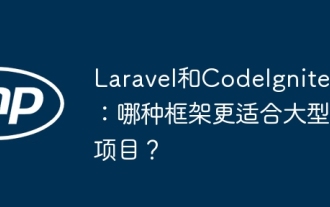
When choosing a framework for large projects, Laravel and CodeIgniter each have their own advantages. Laravel is designed for enterprise-level applications, offering modular design, dependency injection, and a powerful feature set. CodeIgniter is a lightweight framework more suitable for small to medium-sized projects, emphasizing speed and ease of use. For large projects with complex requirements and a large number of users, Laravel's power and scalability are more suitable. For simple projects or situations with limited resources, CodeIgniter's lightweight and rapid development capabilities are more ideal.
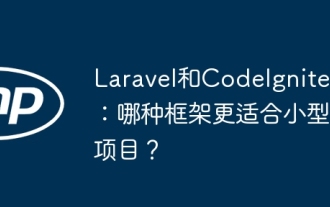
For small projects, Laravel is suitable for larger projects that require strong functionality and security. CodeIgniter is suitable for very small projects that require lightweight and ease of use.
