


Use Python language to connect to Baidu's natural language processing interface to make the program intelligent
Use Python language to connect to Baidu’s natural language processing interface to make the program intelligent
With the rapid development of artificial intelligence, natural language processing has become a hot topic for research and development One of the hot spots. Baidu Natural Language Processing Interface is a powerful tool that can help us implement text sentiment analysis, lexical analysis, named entity recognition and other functions. This article will introduce how to use Python language to connect to Baidu's natural language processing interface, and demonstrate its intelligent capabilities through code examples.
First, we need to register and create a Baidu developer account, and then create a natural language processing application. During the process of creating an application, we can obtain the application's API Key and Secret Key, which will be used in subsequent code.
Next, we need to install Python’s request library requests and json parsing library json.
pip install requests pip install json
Next, we start writing code. First, we need to import the requests and json libraries.
import requests import json
Then, we define a function to obtain the access token of Baidu natural language processing interface. This token needs to be obtained through API Key and Secret Key, and the obtained token will be used in subsequent interface access.
def get_access_token(api_key, secret_key): url = 'https://aip.baidubce.com/oauth/2.0/token' headers = {'Content-Type': 'application/json; charset=UTF-8'} params = {'grant_type': 'client_credentials', 'client_id': api_key, 'client_secret': secret_key} response = requests.post(url, headers=headers, params=params) result = json.loads(response.text) if 'access_token' in result: return result['access_token'] else: return None
Next, we define a function to call the text sentiment analysis function of Baidu’s natural language processing interface. This function receives the text content as a parameter and returns the sentiment analysis results.
def text_sentiment_analysis(access_token, text): url = 'https://aip.baidubce.com/rpc/2.0/nlp/v1/sentiment_classify?access_token=' + access_token headers = {'Content-Type': 'application/json'} data = {'text': text} response = requests.post(url, headers=headers, data=json.dumps(data)) result = json.loads(response.text) if 'items' in result: sentiment = result['items'][0]['sentiment'] confidence = result['items'][0]['confidence'] return sentiment, confidence else: return None, None
Finally, we write the main program, call the above functions, and display the call results.
if __name__ == '__main__': api_key = 'your_api_key' secret_key = 'your_secret_key' access_token = get_access_token(api_key, secret_key) if access_token: text = input('请输入需要进行情感分析的文本:') sentiment, confidence = text_sentiment_analysis(access_token, text) if sentiment and confidence: print('文本情感分析结果:') print('情感:', sentiment) print('置信度:', confidence) else: print('调用百度自然语言处理接口失败!') else: print('获取百度自然语言处理接口的访问令牌失败!')
Through the above code example, we have implemented the call to Baidu's natural language processing interface and implemented the function of text sentiment analysis. We can call other interfaces according to our own needs to implement functions such as lexical analysis and named entity recognition.
To summarize, this article introduces how to use Python language to connect to Baidu’s natural language processing interface to achieve intelligent text analysis functions. This provides us with convenience and flexibility to implement more natural language processing tasks. I hope that through the introduction of this article, readers can flexibly use Baidu's natural language processing interface in their own projects to achieve more intelligent functions.
The above is the detailed content of Use Python language to connect to Baidu's natural language processing interface to make the program intelligent. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
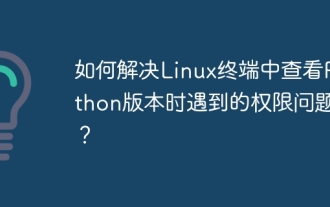
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
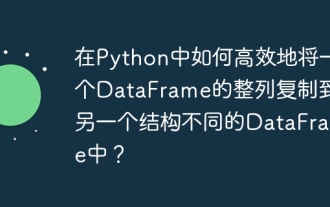
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
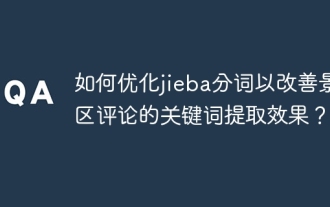
How to optimize jieba word segmentation to improve keyword extraction of scenic spot comments? When using jieba word segmentation to process scenic spot comment data, if the word segmentation results are ignored...
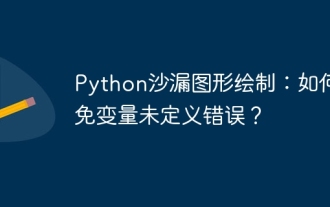
Getting started with Python: Hourglass Graphic Drawing and Input Verification This article will solve the variable definition problem encountered by a Python novice in the hourglass Graphic Drawing Program. Code...
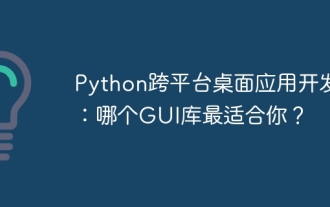
Choice of Python Cross-platform desktop application development library Many Python developers want to develop desktop applications that can run on both Windows and Linux systems...
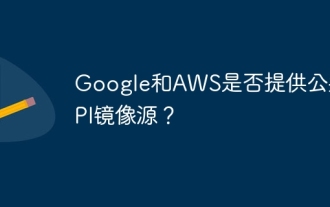
Many developers rely on PyPI (PythonPackageIndex)...
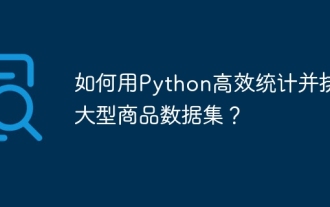
Data Conversion and Statistics: Efficient Processing of Large Data Sets This article will introduce in detail how to convert a data list containing product information to another containing...
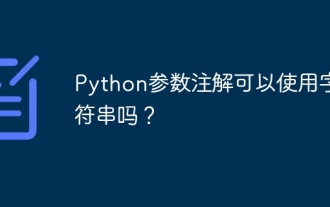
Alternative usage of Python parameter annotations In Python programming, parameter annotations are a very useful function that can help developers better understand and use functions...
