How to handle data deduplication and merging in PHP forms
How to handle data deduplication and merging in PHP forms
When developing web applications, forms are one of the important ways for users to interact with the website. When processing form data, we often encounter data duplication and merging problems. This article will introduce how to use PHP to handle data deduplication and merging in forms.
1. Data deduplication
When users submit a form, they sometimes submit the same data repeatedly due to misoperation or other reasons, which requires deduplication of the data. The following is a simple example that demonstrates how to remove duplicate data:
<?php // 假设用户提交的表单数据保存在一个数组中 $data = $_POST['data']; // 使用array_unique函数去除数组中的重复数据 $uniqueData = array_unique($data); // 输出去重后的数据 foreach ($uniqueData as $item) { echo $item . "<br>"; } ?>
In the above code, we first obtain the form data submitted by the user through the $_POST variable and save it in an array. Then, use the array_unique function to perform a deduplication operation on the array, and save the deduplication result in the $uniqueData variable. Finally, the $uniqueData array is traversed through a foreach loop and the deduplicated data is output.
2. Data Merger
Sometimes, users may submit form data multiple times, and we need to merge these data to obtain a complete data set. Here is an example that shows how to merge form data:
<?php // 假设用户提交的表单数据保存在一个数组中 $data = $_POST['data']; // 使用array_merge函数将多个数组合并为一个数组 $mergedData = array_merge(...$data); // 输出合并后的数据 foreach ($mergedData as $item) { echo $item . "<br>"; } ?>
In the above code, we assume that the form data submitted by the user is saved in an array and save the array in the $data variable. Then, multiple subarrays in the $data array are merged into a single array by using the array_merge function, and the result is saved in the $mergedData variable. Finally, we traverse the $mergedData array through a foreach loop and output the merged data.
3. Comprehensive application of data deduplication and merging
Sometimes, we need to deduplicate and merge form data at the same time. Here is an example that shows how to use array_unique and array_merge functions together to accomplish this task:
<?php // 假设用户提交的表单数据保存在一个数组中 $data = $_POST['data']; // 使用array_unique函数去除数组中的重复数据 $uniqueData = array_unique($data); // 使用array_merge函数将多个数组合并为一个数组 $mergedData = array_merge(...$uniqueData); // 输出去重和合并后的数据 foreach ($mergedData as $item) { echo $item . "<br>"; } ?>
In the above code, we first get the form data submitted by the user through the $_POST variable and save it in in an array. Then, use the array_unique function to perform a deduplication operation on the array, and save the deduplication result in the $uniqueData variable. Next, use the array_merge function to merge multiple subarrays in the $uniqueData array into one array, and save the result in the $mergedData variable. Finally, we traverse the $mergedData array through a foreach loop and output the deduplicated and merged data.
Summary:
The above is how to use PHP to process form data deduplication and merging. By properly using the array_unique and array_merge functions, we can easily deduplicate and merge form data. This is very helpful in improving the efficiency and accuracy of data processing. In actual use, we can flexibly apply it according to specific needs to meet different business needs.
The above is the detailed content of How to handle data deduplication and merging in PHP forms. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


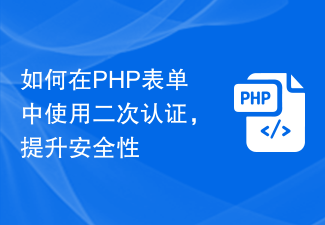
Nowadays, in the era of digitalization and networking, security has become one of the important factors that cannot be ignored in the Internet world. Especially in business scenarios with high data sensitivity, how to improve the security of websites, applications and user data is particularly important. Using two-step authentication in PHP forms to enhance security is a feasible solution. Two-Factor Authentication (2FA), also known as double authentication and multi-factor authentication, refers to the process where the user completes the regular account password.
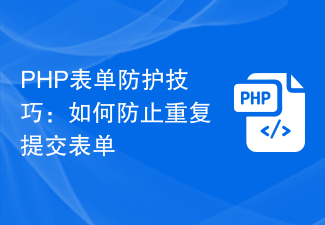
When using PHP forms for data submission, the problem of repeated form submission often occurs. This can lead to inaccurate data or, worse, system crashes. Therefore, it is very important to understand how to prevent duplicate submissions. In this article, I will introduce some PHP form protection techniques to help you effectively prevent repeated form submission problems. 1. Add a token to the form Adding a token to the form is a common way to prevent repeated submissions. The principle of this method is to add a hidden field to the form, which contains
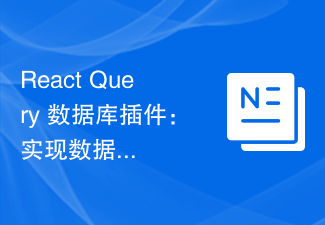
ReactQuery is a powerful data management library that provides many functions and features for working with data. When using ReactQuery for data management, we often encounter scenarios that require data deduplication and denoising. In order to solve these problems, we can use the ReactQuery database plug-in to achieve data deduplication and denoising functions in a specific way. In ReactQuery, you can use database plug-ins to easily process data
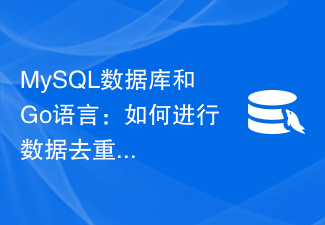
MySQL database and Go language: How to deduplicate data? In actual development work, it is often necessary to deduplicate data to ensure the uniqueness and correctness of the data. This article will introduce how to use MySQL database and Go language to deduplicate data, and provide corresponding sample code. 1. Use MySQL database for data deduplication. MySQL database is a popular relational database management system and has good support for data deduplication. The following introduces two ways to use MySQL database to perform data processing.
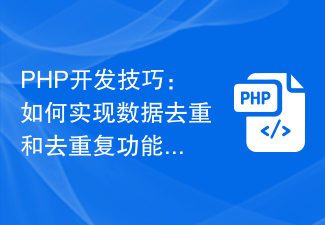
PHP development skills: How to implement data deduplication and deduplication functions. In actual development, we often encounter situations where we need to deduplicate or deduplicate data collections. Whether it is data in the database or data from external data sources, there may be duplicate records. This article will introduce some PHP development techniques to help developers implement data deduplication and deduplication functions. 1. Array-based data deduplication. If the data exists in the form of an array, we can use the array_unique() function to achieve it.
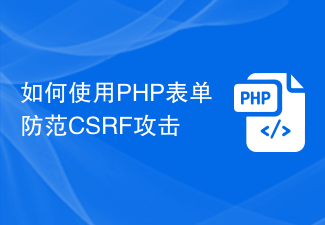
With the continuous development of network technology, security issues have increasingly become an issue that cannot be ignored in network application development. Among them, the cross-site request forgery (CSRF) attack is a common attack method. Its main purpose is to use the user to initiate an illegal request to the background by allowing the user to initiate a malicious request in the browser when the user is logged in to the website. This leads to server-side security vulnerabilities. In PHP applications, using form validation is an effective means of preventing CSRF attacks. Add CSRFToken to verify CSRF attacks
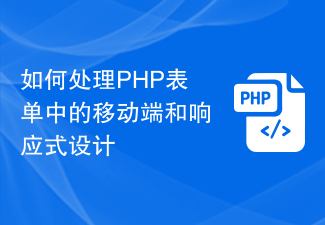
How to deal with mobile and responsive design in PHP forms. With the popularity and frequency of mobile devices increasing, and more and more users using mobile devices to access websites, adapting to mobile has become an important issue. When dealing with PHP forms, we need to consider how to achieve a mobile-friendly interface and responsive design. This article explains how to handle mobile and responsive design in PHP forms and provides code examples. 1. Responsive forms using HTML5 HTML5 provides some new features that can easily implement responsive forms.
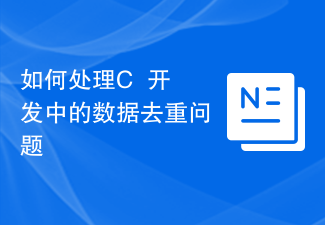
How to deal with the problem of data deduplication in C++ development. In the daily C++ development process, we often encounter situations where we need to deal with data deduplication. Whether you are deduplicating data in one container or between multiple containers, you need to find an efficient and reliable method. This article will introduce some common data deduplication techniques to help readers deal with data deduplication problems in C++ development. 1. Sorting deduplication method Sorting deduplication method is a common and simple data deduplication method. First, store the data to be deduplicated in a container, and then
