


Practical Guide to Developing Brain Map Functions with PHP and Vue
Practical Guide to Developing Brain Map Functions with PHP and Vue
Introduction:
Brain mapping is a popular way of organizing information that can organize complex thinking or knowledge Relationships are presented graphically. In modern Internet application development, adding brain mapping functions can improve user experience and data visualization effects. This article will introduce how to use PHP and Vue to develop mind mapping functions, and provide practical guidance and code examples.
Technical introduction:
- PHP: PHP is a server-side scripting language used to develop web applications. In this article, we will use PHP to handle database interactions and API requests.
- Vue: Vue is a popular JavaScript framework used for building front-end user interfaces. In this article, we will use Vue to implement the front-end component of the brain map.
Development preparation:
Before we start, we need to ensure that the development environment of PHP and Vue has been installed. The required packages can be downloaded and installed on the official website.
Step 1: Database design
First, we need to design a database to store the relevant data of the brain map. The following is a simplified database table design example:
CREATE TABLE `nodes` ( `id` int(11) NOT NULL AUTO_INCREMENT, `title` varchar(255) NOT NULL, `parent_id` int(11) DEFAULT NULL, PRIMARY KEY (`id`), FOREIGN KEY (`parent_id`) REFERENCES `nodes` (`id`) ); CREATE TABLE `edges` ( `id` int(11) NOT NULL AUTO_INCREMENT, `from_node_id` int(11) NOT NULL, `to_node_id` int(11) NOT NULL, PRIMARY KEY (`id`), FOREIGN KEY (`from_node_id`) REFERENCES `nodes` (`id`), FOREIGN KEY (`to_node_id`) REFERENCES `nodes` (`id`) );
Step 2: Backend Development
Next, we start developing the backend code. First, create a PHP file named "api.php". In this file, we will implement the server-side API interface.
<?php // 连接数据库 $db = new mysqli('localhost', 'username', 'password', 'database'); // 获取所有节点 function getAllNodes() { global $db; $result = $db->query("SELECT * FROM `nodes`"); $nodes = array(); while ($row = $result->fetch_assoc()) { $nodes[] = $row; } return $nodes; } // 获取所有边 function getAllEdges() { global $db; $result = $db->query("SELECT * FROM `edges`"); $edges = array(); while ($row = $result->fetch_assoc()) { $edges[] = $row; } return $edges; } // 设置HTTP响应标头 header('Content-Type: application/json'); // 处理API请求 $method = $_SERVER['REQUEST_METHOD']; $path = $_SERVER['REQUEST_URI']; if ($method === 'GET' && $path === '/api/nodes') { echo json_encode(getAllNodes()); } else if ($method === 'GET' && $path === '/api/edges') { echo json_encode(getAllEdges()); } else { http_response_code(404); echo json_encode(array('error' => 'Not Found')); } ?>
Step 3: Front-end development
Now, we start developing the front-end code. Create a Vue component named "MindMap.vue". In this component, we will use Vue's data binding and componentization technology to implement the interactive function of the brain map.
<template> <div> <div id="mindmap"></div> </div> </template> <script> export default { mounted() { // 从API获取数据 fetch('/api/nodes') .then(response => response.json()) .then(nodes => { // 使用数据来绘制脑图 const mindmap = new d3.Mindmap('#mindmap') .nodes(nodes) .edges(this.edges) .render(); }); }, computed: { edges() { // 从API获取边 return fetch('/api/edges') .then(response => response.json()) .then(edges => edges); } } } </script> <style> #mindmap { width: 800px; height: 600px; } </style>
Step Four: Run the Application
Now, we have completed the development of the back-end code and the front-end code. You can run the following command in the command line to start the local server and view the running effect of the application:
php -S localhost:8000
Open the browser and visit "http://localhost:8000" to view the mind mapping function of the application .
Conclusion:
This article introduces a practical guide on how to use PHP and Vue to develop brain mapping functions, and provides relevant code examples. By studying this article, you can learn how to use PHP and Vue to implement database interaction and front-end componentization functions of brain maps. I hope this article can be helpful to your development work, and I wish you happy development!
The above is the detailed content of Practical Guide to Developing Brain Map Functions with PHP and Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


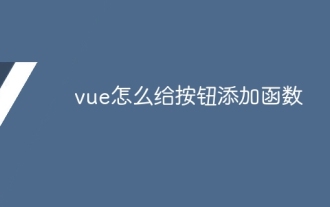
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.

In PHP, the final keyword is used to prevent classes from being inherited and methods being overwritten. 1) When marking the class as final, the class cannot be inherited. 2) When marking the method as final, the method cannot be rewritten by the subclass. Using final keywords ensures the stability and security of your code.

The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
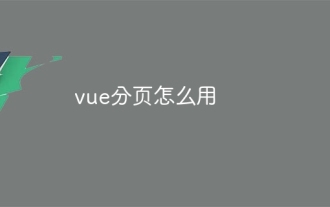
Pagination is a technology that splits large data sets into small pages to improve performance and user experience. In Vue, you can use the following built-in method to paging: Calculate the total number of pages: totalPages() traversal page number: v-for directive to set the current page: currentPage Get the current page data: currentPageData()
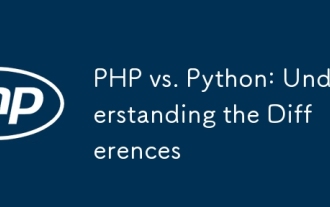
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
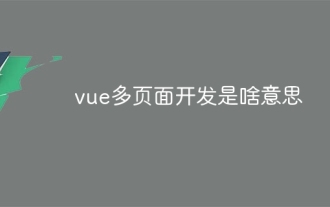
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.

Function interception in Vue is a technique used to limit the number of times a function is called within a specified time period and prevent performance problems. The implementation method is: import the lodash library: import { debounce } from 'lodash'; Use the debounce function to create an intercept function: const debouncedFunction = debounce(() => { / Logical / }, 500); Call the intercept function, and the control function is called at most once in 500 milliseconds.
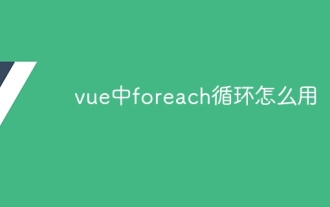
The foreach loop in Vue.js uses the v-for directive, which allows developers to iterate through each element in an array or object and perform specific operations on each element. The syntax is as follows: <template> <ul> <li v-for="item in items>>{{ item }}</li> </ul> </template>&am
