


PHP error: What should I do if I use an undefined constant as a property name?
PHP error: What should I do if I use an undefined constant as a property name?
In PHP development, we often use classes and objects to organize and manage code. In the process of defining a class, the attributes of the class (i.e. member variables) play an important role in saving data. However, when we use properties, sometimes an error occurs when using undefined constants as property names. This article explains the causes of this error and provides several solutions.
First, let's look at a simple example to demonstrate this problem. Suppose we have a class called "Person" that represents information about a person. In this class, we want to define an attribute to save the name of the person. We might define the attribute like this:
class Person {
const NAME = "John Doe";
}
Then, We can get the person's name by accessing this property:
$person = new Person();
echo $person->NAME; //Output: John Doe
The above code seems to be fine, but when we use undefined constants as property names, an error will appear. Suppose we want to get the person's age through the constant "AGE":
echo $person->AGE; //Error: Undefined property: Person::$AGE
This error appears The reason is that when we use an undefined constant as a property name, PHP will treat it as an undefined property when accessing the property. At this point, PHP will throw an "Undefined property" error.
There are many ways to solve this problem, we will introduce them one by one:
- Use string literals to access properties
The simplest solution The method is to use a string literal (that is, a string directly using the property name) to access the property. Modify the above example and the code is as follows:
$person = new Person();
echo $person->{'AGE'}; //Output: John Doe
is in In this method, we use curly braces to enclose the property name, and use quotes (either single or double quotes) to enclose the string literal. This way, PHP will treat it as a string rather than an undefined constant.
- Use class constants to access properties
Another solution is to use class constants to access properties. In the Person class, we can define a constant named "AGE" and then access the properties through this constant. The code example is as follows:
class Person {
const NAME = "John Doe"; const AGE = 30;
}
$person = new Person();
echo $person::AGE; //Output: 30
By using class constants, we can directly access properties without causing errors. This approach is more self-explanatory in the code and easier to maintain.
- Use the magic method __get() to handle undefined attributes
The last solution is to use the magic method __get() to handle undefined attribute access. In the Person class, we can implement the __get() method and determine whether the attribute is undefined inside the method. If the property is undefined, we can throw an exception or return a default value. The code example is as follows:
class Person {
const NAME = "John Doe"; public function __get($name) { if ($name == 'AGE') { return 30; } // 处理其他未定义的属性 throw new Exception("Undefined property: Person::$$name"); }
}
$person = new Person();
echo $person->AGE; //Output: 30
By implementing the __get() method, we can customize the processing logic for undefined attributes so that relevant processing can be performed before reporting an error.
Summary:
In PHP development, using undefined constants as property names is an error-prone point. However, we can solve this problem by using string literals, using class constants, and using the magic method __get(). Choosing the appropriate approach requires judgment based on specific needs and code structure. No matter which method is used, the readability and maintainability of the code need to be ensured.
I hope this article can help you solve the error problem of using undefined constants as attribute names and make PHP development smoother!
The above is the detailed content of PHP error: What should I do if I use an undefined constant as a property name?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


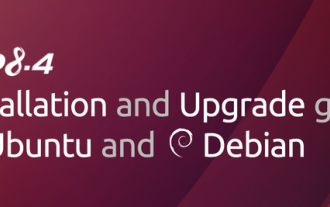
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
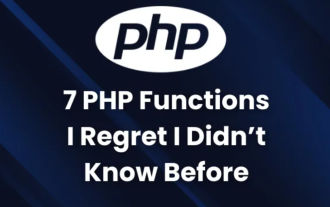
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
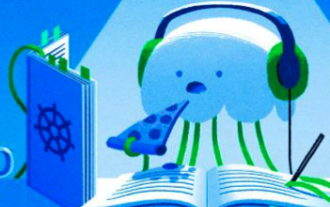
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
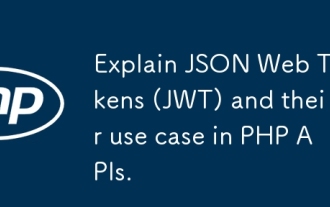
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
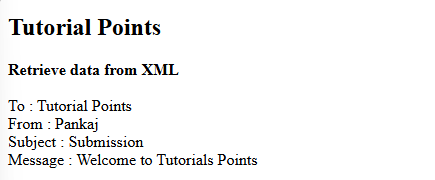
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
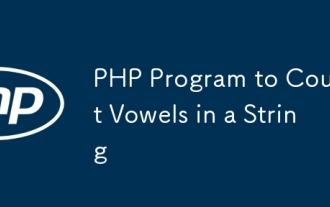
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
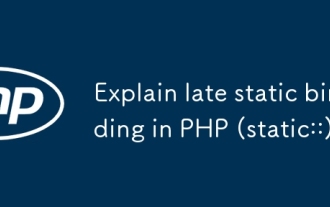
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
