


How to perform image classification on pictures using Python
How to use Python to classify images
With the development of the fields of image processing and machine learning, image classification has become an important task. As a flexible and powerful programming language, Python provides many tools and libraries to make image classification easier and more efficient. This article will introduce how to use Python to classify images and provide relevant code examples.
- Preparation work:
Before we start, we need to install the corresponding Python library. Among them, the most important are the OpenCV and Keras libraries. You can install it via pip using the following command:
pip install opencv-python pip install keras
In addition, we also need to download a pre-trained model suitable for image classification. In this article, we will use the ResNet50 model. You can download it using the following command:
python -m keras.applications.resnet50
- Load image data:
First, we need to load the image data into the program. We can use thecv2.imread()
function of the OpenCV library to read image files. The following is a sample code to load image data:
import cv2 def load_image(image_path): img = cv2.imread(image_path) img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) # 转换为RGB格式 return img image = load_image('image.jpg')
- Preprocess image data:
Before doing image classification, we need to preprocess the image data to adapt it to our Model. Typically, we perform scaling, regularization, or cropping of images. The following is a sample code to scale and regularize an image:
import cv2 import numpy as np def preprocess_image(image): image = cv2.resize(image, (224, 224)) # 缩放图像为224x224像素 image = image.astype("float32") # 将图像数据类型转换为float32 image /= 255 # 归一化图像数据 return image preprocessed_image = preprocess_image(image)
- Loading a pre-trained model:
Before doing image classification, we need to load a pre-trained model. In this article, we will use the ResNet50 model. Here is the sample code to load the pre-trained model:
from keras.applications.resnet50 import ResNet50 from keras.preprocessing import image from keras.applications.resnet50 import preprocess_input, decode_predictions import numpy as np model = ResNet50(weights='imagenet')
- Do image classification:
Now, we are ready to classify the images. We will use the loaded model to predict the preprocessed image data and output the classification results. Here is the sample code for image classification:
def classify_image(image): x = image[np.newaxis, ...] # 将图像数据加一维以适应模型输入 predictions = model.predict(x) # 进行图像分类 decoded_predictions = decode_predictions(predictions, top=3)[0] # 解码预测结果 return decoded_predictions predictions = classify_image(preprocessed_image) print(predictions)
- Explanation of results:
Finally, we will explain the prediction results. Based on the pre-trained model we loaded, the model will return the top three predictions and their corresponding probabilities. Here is sample code that explains the prediction results:
def explain_predictions(predictions): for pred in predictions: print(f"类别:{pred[1]},概率:{pred[2]*100}%") explain_predictions(predictions)
At this point, we have completed the process of classifying images using Python. By loading the pre-trained model and pre-processing the image data, we can easily perform image classification and interpret the prediction results.
Summary:
This article introduces how to use Python to classify images. By installing the OpenCV and Keras libraries, we can load and preprocess image data. Using a pre-trained model, we can classify images and interpret the predictions. I hope this article can help readers understand how to use Python for image classification and provide a reference for further exploration in the fields of image processing and machine learning.
References:
- https://docs.opencv.org/master/d6/d00/tutorial_py_root.html
- https://keras.io /api/applications/
- https://numpy.org/doc/stable/
The above is the detailed content of How to perform image classification on pictures using Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


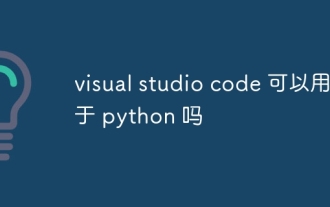
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
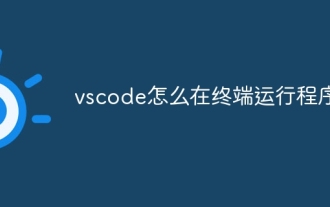
In VS Code, you can run the program in the terminal through the following steps: Prepare the code and open the integrated terminal to ensure that the code directory is consistent with the terminal working directory. Select the run command according to the programming language (such as Python's python your_file_name.py) to check whether it runs successfully and resolve errors. Use the debugger to improve debugging efficiency.
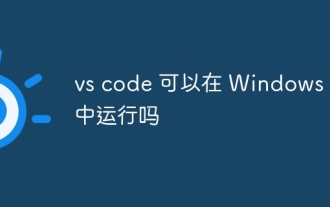
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
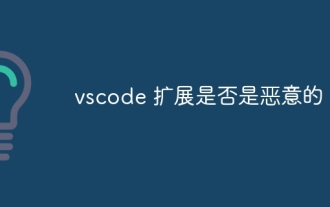
VS Code extensions pose malicious risks, such as hiding malicious code, exploiting vulnerabilities, and masturbating as legitimate extensions. Methods to identify malicious extensions include: checking publishers, reading comments, checking code, and installing with caution. Security measures also include: security awareness, good habits, regular updates and antivirus software.
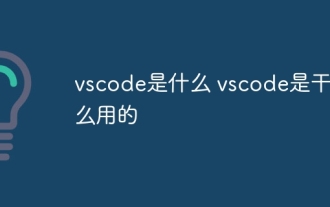
VS Code is the full name Visual Studio Code, which is a free and open source cross-platform code editor and development environment developed by Microsoft. It supports a wide range of programming languages and provides syntax highlighting, code automatic completion, code snippets and smart prompts to improve development efficiency. Through a rich extension ecosystem, users can add extensions to specific needs and languages, such as debuggers, code formatting tools, and Git integrations. VS Code also includes an intuitive debugger that helps quickly find and resolve bugs in your code.
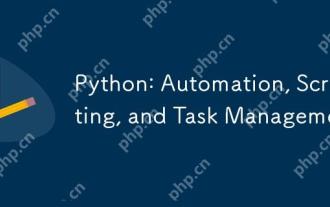
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
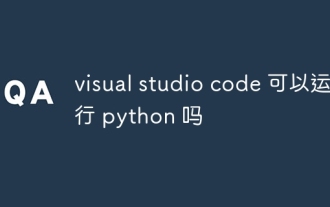
VS Code not only can run Python, but also provides powerful functions, including: automatically identifying Python files after installing Python extensions, providing functions such as code completion, syntax highlighting, and debugging. Relying on the installed Python environment, extensions act as bridge connection editing and Python environment. The debugging functions include setting breakpoints, step-by-step debugging, viewing variable values, and improving debugging efficiency. The integrated terminal supports running complex commands such as unit testing and package management. Supports extended configuration and enhances features such as code formatting, analysis and version control.
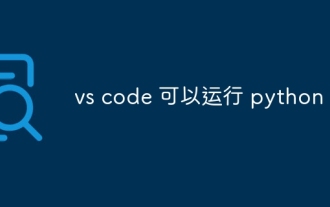
Yes, VS Code can run Python code. To run Python efficiently in VS Code, complete the following steps: Install the Python interpreter and configure environment variables. Install the Python extension in VS Code. Run Python code in VS Code's terminal via the command line. Use VS Code's debugging capabilities and code formatting to improve development efficiency. Adopt good programming habits and use performance analysis tools to optimize code performance.
