


Golang image processing: How to extract feature points and color analysis of images
Golang image processing: How to extract feature points and color analysis of images
Introduction
With the development of the Internet and mobile devices, image processing Technology plays an increasingly important role in various fields. In image processing, feature point extraction and color analysis are two very common and critical tasks. This article will introduce how to use Golang to extract feature points and color analysis of images, and provide corresponding code examples.
Image feature point extraction
Image feature point extraction refers to finding the key points representing the local features of the object from the image. These key points can be used for image matching, image recognition, target tracking and other applications. In Golang, we can use the github.com/anthonynsimon/bild/feature/brisk
package to extract feature points of images. Here is a simple example:
package main import ( "image" "image/color" "log" "os" "github.com/anthonynsimon/bild/feature/brisk" "github.com/anthonynsimon/bild/imgio" "github.com/anthonynsimon/bild/transform" ) func main() { // 打开图像文件 imageFile, err := os.Open("input.jpg") if err != nil { log.Fatal(err) } defer imageFile.Close() // 解码图像 inputImage, _, err := image.Decode(imageFile) if err != nil { log.Fatal(err) } // 缩放图像以提高速度和准确性 scaledImage := transform.Resize(inputImage, 300, 0, transform.Linear) // 提取特征点 features := brisk.Detect(scaledImage, nil) // 在图像上绘制特征点 outputImage := imgio.CloneImage(inputImage) drawFeatures(outputImage, features) // 保存结果图像 outputFile, err := os.Create("output.jpg") if err != nil { log.Fatal(err) } defer outputFile.Close() // 编码并保存图像 err = imgio.JPEGEncoder(100).Encode(outputFile, outputImage) if err != nil { log.Fatal(err) } } // 在图像上绘制特征点 func drawFeatures(img draw.Image, features []brisk.Feature) { drawer := draw.Draw(img, img.Bounds(), img, image.ZP, draw.Src) for _, feature := range features { drawer.DrawRect(feature.Rectangle, color.RGBA{255, 0, 0, 255}) } }
In this example, we first open the image file using the Open
function and decode the image using the Decode
function. Then, we use the Resize
function to scale the image, which can improve the speed and accuracy of feature point extraction. Next, we use the Detect
function to extract feature points, and use the DrawRect
function to draw the feature points on the original image. Finally, we encode and save the resulting image in JPEG format using the Encode
function.
Image color analysis
Image color analysis refers to the statistics and analysis of different colors appearing in images. Color information is very important in image processing and can be used for tasks such as image classification and object recognition. In Golang, we can use the github.com/anthonynsimon/bild/analysis
package to perform color analysis. Here is a simple example:
package main import ( "image" "log" "os" "github.com/anthonynsimon/bild/analysis" "github.com/anthonynsimon/bild/imgio" ) func main() { // 打开图像文件 imageFile, err := os.Open("input.jpg") if err != nil { log.Fatal(err) } defer imageFile.Close() // 解码图像 inputImage, _, err := image.Decode(imageFile) if err != nil { log.Fatal(err) } // 进行颜色分析 colors := analysis.ExtractColors(inputImage, 10) // 打印结果 for _, color := range colors { log.Printf("Color: %v, Frequency: %v", color.Color, color.Frequency) } }
In this example, we first open the image file using the Open
function and decode the image using the Decode
function. We then use the ExtractColors
function to perform color analysis on the image and specify the number of colors to extract. Finally, we use the log.Printf
function to print the results.
Conclusion
This article introduces how to use Golang to extract feature points and color analysis of images, and provides corresponding code examples. By learning and using these techniques, we can better understand and process image data and achieve better results in various fields of image processing. I hope this article can be helpful to readers in their study and practice of image processing.
The above is the detailed content of Golang image processing: How to extract feature points and color analysis of images. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


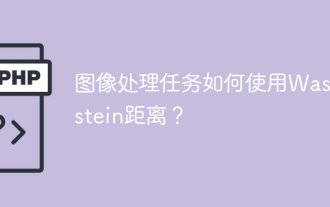
Wasserstein distance, also known as EarthMover's Distance (EMD), is a metric used to measure the difference between two probability distributions. Compared with traditional KL divergence or JS divergence, Wasserstein distance takes into account the structural information between distributions and therefore exhibits better performance in many image processing tasks. By calculating the minimum transportation cost between two distributions, Wasserstein distance is able to measure the minimum amount of work required to transform one distribution into another. This metric is able to capture the geometric differences between distributions, thereby playing an important role in tasks such as image generation and style transfer. Therefore, the Wasserstein distance becomes the concept
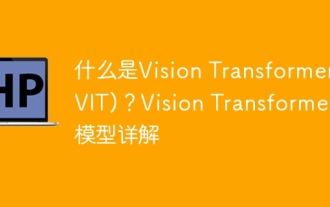
VisionTransformer (VIT) is a Transformer-based image classification model proposed by Google. Different from traditional CNN models, VIT represents images as sequences and learns the image structure by predicting the class label of the image. To achieve this, VIT divides the input image into multiple patches and concatenates the pixels in each patch through channels and then performs linear projection to achieve the desired input dimensions. Finally, each patch is flattened into a single vector, forming the input sequence. Through Transformer's self-attention mechanism, VIT is able to capture the relationship between different patches and perform effective feature extraction and classification prediction. This serialized image representation is
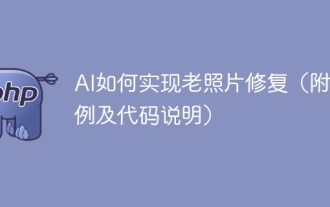
Old photo restoration is a method of using artificial intelligence technology to repair, enhance and improve old photos. Using computer vision and machine learning algorithms, the technology can automatically identify and repair damage and flaws in old photos, making them look clearer, more natural and more realistic. The technical principles of old photo restoration mainly include the following aspects: 1. Image denoising and enhancement. When restoring old photos, they need to be denoised and enhanced first. Image processing algorithms and filters, such as mean filtering, Gaussian filtering, bilateral filtering, etc., can be used to solve noise and color spots problems, thereby improving the quality of photos. 2. Image restoration and repair In old photos, there may be some defects and damage, such as scratches, cracks, fading, etc. These problems can be solved by image restoration and repair algorithms
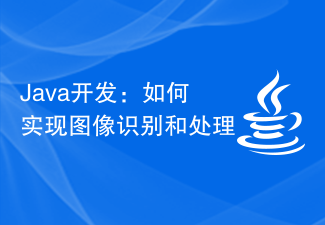
Java Development: A Practical Guide to Image Recognition and Processing Abstract: With the rapid development of computer vision and artificial intelligence, image recognition and processing play an important role in various fields. This article will introduce how to use Java language to implement image recognition and processing, and provide specific code examples. 1. Basic principles of image recognition Image recognition refers to the use of computer technology to analyze and understand images to identify objects, features or content in the image. Before performing image recognition, we need to understand some basic image processing techniques, as shown in the figure
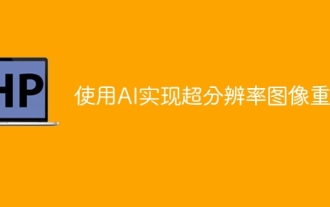
Super-resolution image reconstruction is the process of generating high-resolution images from low-resolution images using deep learning techniques, such as convolutional neural networks (CNN) and generative adversarial networks (GAN). The goal of this method is to improve the quality and detail of images by converting low-resolution images into high-resolution images. This technology has wide applications in many fields, such as medical imaging, surveillance cameras, satellite images, etc. Through super-resolution image reconstruction, we can obtain clearer and more detailed images, which helps to more accurately analyze and identify targets and features in images. Reconstruction methods Super-resolution image reconstruction methods can generally be divided into two categories: interpolation-based methods and deep learning-based methods. 1) Interpolation-based method Super-resolution image reconstruction based on interpolation

PHP study notes: Face recognition and image processing Preface: With the development of artificial intelligence technology, face recognition and image processing have become hot topics. In practical applications, face recognition and image processing are mostly used in security monitoring, face unlocking, card comparison, etc. As a commonly used server-side scripting language, PHP can also be used to implement functions related to face recognition and image processing. This article will take you through face recognition and image processing in PHP, with specific code examples. 1. Face recognition in PHP Face recognition is a
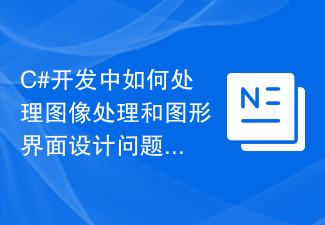
How to deal with image processing and graphical interface design issues in C# development requires specific code examples. Introduction: In modern software development, image processing and graphical interface design are common requirements. As a general-purpose high-level programming language, C# has powerful image processing and graphical interface design capabilities. This article will be based on C#, discuss how to deal with image processing and graphical interface design issues, and give detailed code examples. 1. Image processing issues: Image reading and display: In C#, image reading and display are basic operations. Can be used.N

The Scale Invariant Feature Transform (SIFT) algorithm is a feature extraction algorithm used in the fields of image processing and computer vision. This algorithm was proposed in 1999 to improve object recognition and matching performance in computer vision systems. The SIFT algorithm is robust and accurate and is widely used in image recognition, three-dimensional reconstruction, target detection, video tracking and other fields. It achieves scale invariance by detecting key points in multiple scale spaces and extracting local feature descriptors around the key points. The main steps of the SIFT algorithm include scale space construction, key point detection, key point positioning, direction assignment and feature descriptor generation. Through these steps, the SIFT algorithm can extract robust and unique features, thereby achieving efficient image processing.
