How to solve the '[Vue warn]: v-model is not supported on' error
How to solve the "[Vue warn]: v-model is not supported on" error
Vue.js is a popular JavaScript framework that is widely used in Build flexible user interfaces. During the development process using Vue.js, you sometimes encounter an error message: "[Vue warn]: v-model is not supported on". This error message usually appears when using v-model to bind data.
The reason for this error message is that the usage of v-model is incorrect or does not support binding on certain elements. Don't worry, below we will introduce some ways to solve this problem and give corresponding code examples.
- Use the correct elements
v-model can only be used on some specific form elements, such as <input>
, <textarea>
, <select>
, etc. If you try to use v-model on an element that doesn't support it, you'll get an error. Therefore, make sure your v-model is bound to the correct element. Here is a correct example of using v-model:
1 2 3 4 5 6 |
|
- Using the model attribute
Sometimes, you may need to bind on an element that does not support v-model data. At this time, you can use the model attribute of Vue.js to solve this problem. You can use v-model on non-form elements by defining a custom component and adding the model attribute on it. Here is an example of using the model attribute:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
|
In the above example, we have defined a custom component my-component
and added the model attribute in it. value
The property is bound as the value passed in by the parent component, the data is updated through the updateValue
method, and this.$emit('input', 'New Value')
Pass the updated value to the parent component.
- Using modifiers
Finally, you can also solve the problem of elements that v-model does not support by using modifiers. Vue.js provides some modifiers for binding according to different usage scenarios.
For example, in some check boxes, you can use the .lazy
modifier to let v-model update the data only when the change event is triggered. The following is an example of using modifiers:
1 2 3 4 5 6 |
|
In the above example, the .lazy
modifier causes v-model to update data only when the change event is triggered, rather than when the input event is triggered. Update immediately when triggered.
Summary:
The above is the method to solve the "[Vue warn]: v-model is not supported on" error. Please ensure that v-model is only used on appropriate form elements. If you need to bind data on non-form elements, consider using the model attribute or modifier.
I hope this article can help you solve this problem and make your Vue.js development smoother.
The above is the detailed content of How to solve the '[Vue warn]: v-model is not supported on' error. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


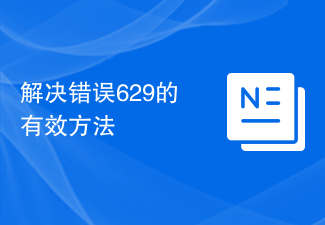
Error 629 refers to a common problem encountered when using a computer or network, indicating that a malfunction or error has occurred in the currently connected network or server. When error 629 occurs, users cannot access the Internet normally, which is very troublesome for many people. However, we don’t need to worry too much as error 629 usually has some simple solutions. First, we can try to restart the computer and network devices, such as routers or modems. Sometimes, this error may just be a temporary problem and a reboot can help restart the
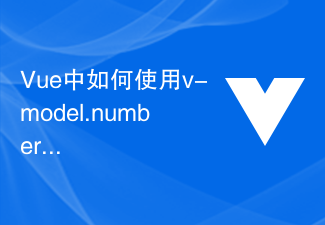
In Vue, v-model is an important instruction used to implement two-way binding. It allows us to easily synchronize user input to Vue's data attribute. But in some cases, we need to convert the data, such as converting the string type input by the user into a numeric type. In this case, we need to use the .number modifier of v-model to achieve this. Basic usage of v-model.number v-model.number is a modification of v-model
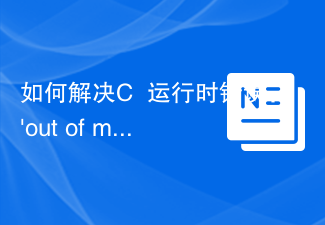
How to solve C++ runtime error: 'outofmemoryexception'? Introduction: In C++ programming, out of memory situations are often encountered, especially when processing large data sets or complex algorithms. 'outofmemoryexception' (out of memory exception) is thrown when the program cannot allocate additional memory to meet its needs. This article will describe how to solve this type of problem and give corresponding code examples. Check for memory leak issues: Memory Leak
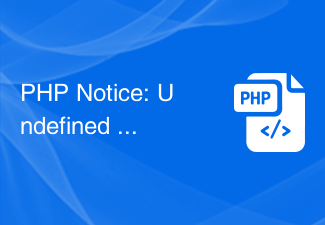
Solution to PHPNotice:Undefinedindex error When using PHP to develop applications, we often encounter the error message "PHPNotice:Undefinedindex". This error is usually caused by accessing an undefined array index. This article will introduce several methods to solve the Undefinedindex error and give corresponding code examples. Use isset() function to check if array index exists first
![How to solve '[Vue warn]: Avoid using non-primitive' error](https://img.php.cn/upload/article/000/465/014/169234247419966.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
How to solve the "[Vuewarn]:Avoidusingnon-primitive" error In Vue.js programming, you may encounter an error named "[Vuewarn]:Avoidusingnon-primitive". This error usually occurs when you use Vue.js components, especially when using non-primitive data types in props or data.
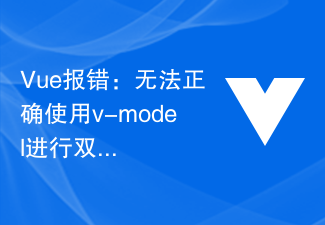
Vue error: v-model cannot be used correctly for two-way data binding. How to solve it? Introduction: Two-way data binding is a very common and powerful feature when developing with Vue. However, sometimes we may encounter a problem, that is, when we try to use v-model for two-way data binding, we encounter an error. This article describes the cause and solution of this problem, and provides a code example to demonstrate how to solve the problem. Problem Description: When we try to use v-model in Vue

Using v-model's two-way binding in Vue to optimize application data performance In Vue, we often use the v-model directive to achieve two-way binding between form elements and data. This two-way binding greatly simplifies the development process and improves user experience. However, since v-model needs to listen to the input event of the form element, this two-way binding may cause certain performance problems when the amount of data is large. This article will introduce how to optimize data performance when using v-model and provide a
![How to deal with '[Vue warn]: Property or method is not defined' error](https://img.php.cn/upload/article/000/465/014/169305366131078.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
How to deal with the "[Vuewarn]:Propertyormethodisnotdefined" error When developing applications using the Vue framework, we sometimes encounter the "[Vuewarn]:Propertyormethodisnotdefined" error. This error usually occurs when we try to access a property or method that is not defined in the Vue instance. Next, we will introduce some common situations and solutions
