How to crop a specified area of an image using PHP
How to use PHP to crop a designated area of an image
In the process of website development, we often encounter situations where images need to be cropped. For example, the avatar uploaded by the user needs to be adjusted. size or just cut off part of it. As a commonly used server-side scripting language, PHP provides a wealth of image processing functions, which can easily help us realize the image cropping function.
The following takes the use of PHP to crop a specified area of an image as an example to introduce the implementation steps and related code examples in detail.
Step 1: Install PHP GD library
Before using PHP for image processing, you need to ensure that the GD library has been installed on the server. If it is not installed, you can install it through the following command:
sudo apt-get install php-gd
Step 2: Create a cropping function
First, we need to create a function to crop the image. The following is a simple example of a cropping function:
function cropImage($sourcePath, $targetPath, $x, $y, $width, $height) { // 获取图片的类型 $imageType = exif_imagetype($sourcePath); // 根据图片类型创建画布 switch ($imageType) { case IMAGETYPE_JPEG: $sourceImage = imagecreatefromjpeg($sourcePath); break; case IMAGETYPE_PNG: $sourceImage = imagecreatefrompng($sourcePath); break; case IMAGETYPE_GIF: $sourceImage = imagecreatefromgif($sourcePath); break; default: return false; } // 创建裁剪后的画布 $targetImage = imagecreatetruecolor($width, $height); // 裁剪图片 imagecopy($targetImage, $sourceImage, 0, 0, $x, $y, $width, $height); // 保存裁剪后的图片 imagejpeg($targetImage, $targetPath); // 释放资源 imagedestroy($sourceImage); imagedestroy($targetImage); return true; }
In this function, we first create an original canvas based on the type of image, and then create a target canvas based on the given cropping area. Then use the imagecopy
function to copy the specified area in the original canvas to the target canvas. Finally, use the imagejpeg
function to save the cropped image and release the resources.
Step 3: Test the cropping function
To facilitate testing, we can create a simple form page to allow users to enter cropping parameters. The following is a simple HTML code example:
<form action="crop.php" method="post" enctype="multipart/form-data"> <label for="image">选择要裁剪的图片:</label> <input type="file" name="image" id="image"> <br> <label for="x">裁剪起点 x 坐标:</label> <input type="text" name="x" id="x"> <br> <label for="y">裁剪起点 y 坐标:</label> <input type="text" name="y" id="y"> <br> <label for="width">裁剪宽度:</label> <input type="text" name="width" id="width"> <br> <label for="height">裁剪高度:</label> <input type="text" name="height" id="height"> <br> <input type="submit" value="裁剪"> </form>
In this form, the user can select an image file and enter the starting point coordinates, width and height of the cropping area. After submitting the form, the form data will be sent to the crop.php
page for cropping processing.
Next, we need to create a crop.php
page to receive the form data and use the crop function we created before to crop the image.
<?php if ($_SERVER['REQUEST_METHOD'] == 'POST') { $sourcePath = $_FILES['image']['tmp_name']; $targetPath = 'path/to/save/cropped/image.jpg'; $x = $_POST['x']; $y = $_POST['y']; $width = $_POST['width']; $height = $_POST['height']; if (cropImage($sourcePath, $targetPath, $x, $y, $width, $height)) { echo '图片裁剪成功!'; } else { echo '图片裁剪失败!'; } } ?>
In this crop.php
page, we first determine whether the request method is POST, and then obtain the uploaded image file path and cropping parameters. Finally, call the cropping function to crop the image and return the corresponding prompt information.
Summary:
Through the above steps, we can use PHP to crop the specified area of the image. When the user uploads the image and enters the appropriate cropping parameters, the system will automatically crop the image in the specified area and save it to the specified path.
It is worth noting that in actual applications, some security checks may be required, such as image type verification and parameter legality checks, to ensure the security and robustness of the system.
The above is the detailed content of How to crop a specified area of an image using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


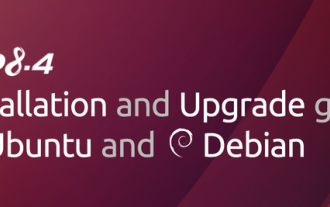
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
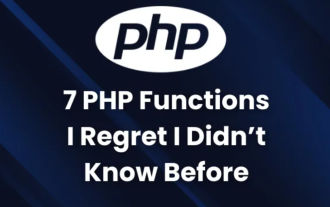
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
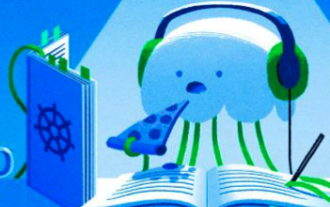
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
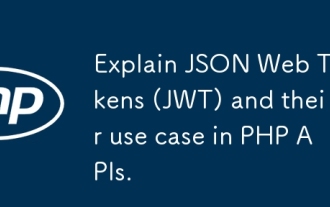
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
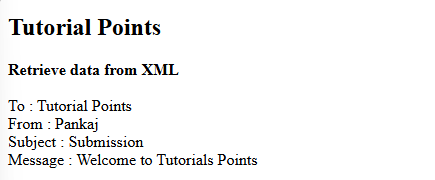
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
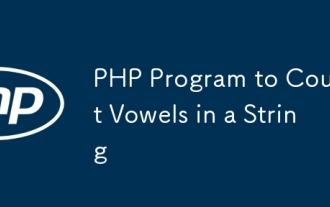
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
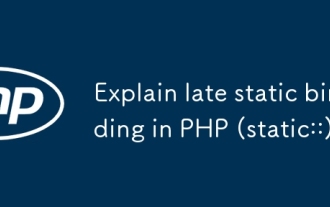
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
