


How to implement quick preview and switching functions of images through Vue?
How to implement quick preview and switching functions of images through Vue?
Vue is a JavaScript framework for building user interfaces. It can help us achieve dynamic data binding and component development. During the development process, we often encounter the need to provide users with image preview and switching functions. This article will introduce how to use Vue to implement this function, and use code examples to help readers better understand and apply this technology.
First of all, we need to introduce appropriate plug-ins into the Vue project to help us realize the preview and switching functions of images. Here we will use the vue-awesome-swiper plug-in, which is a Vue-based carousel plug-in with rich configuration options and flexible API interface.
First, we need to install the vue-awesome-swiper plug-in. Execute the following command in the command line:
npm install vue-awesome-swiper --save
After the installation is complete, register the plug-in in the entry file of Vue (usually main.js):
import Vue from 'vue' import VueAwesomeSwiper from 'vue-awesome-swiper' Vue.use(VueAwesomeSwiper)
Next, when you need to use the image preview and toggle function components.
The HTML structure is as follows:
<template> <div class="gallery"> <div class="swiper-container" ref="swiper"> <div class="swiper-wrapper"> <div class="swiper-slide" v-for="(image, index) in images" :key="index"> <img src="/static/imghw/default1.png" data-src="image" class="lazy" : @click="showGallery(index)" alt="image"> </div> </div> </div> <div class="swiper-pagination" ref="pagination"></div> </div> </template>
In the JavaScript part, we need to set the image data and configure the vue-awesome-swiper plug-in:
<script> export default { data() { return { images: [ 'image1.jpg', 'image2.jpg', 'image3.jpg', 'image4.jpg' ], swiperOption: { autoplay: { delay: 3000, disableOnInteraction: false }, pagination: { el: 'swiper-pagination' } } } }, mounted() { this.$nextTick(() => { this.initSwiper() }) }, methods: { initSwiper() { this.swiper = new Swiper(this.$refs.swiper, this.swiperOption) }, showGallery(index) { this.swiper.slideTo(index, 0) // 切换到指定索引的图片 this.$refs.gallery.style.display = 'block' // 显示图片预览 } } } </script>
In the above code, we first An array images is defined to store the path of the image. Then, we use the configuration options of the vue-awesome-swiper plugin to initialize the carousel component. When the user clicks on a picture, call the showGallery method to switch to the corresponding picture and display a preview of the picture.
Finally, add the image preview style in the CSS part:
<style scoped> .gallery { position: fixed; top: 0; left: 0; width: 100%; height: 100%; background-color: rgba(0, 0, 0, 0.8); display: none; z-index: 9999; } .gallery img { max-height: 80%; max-width: 80%; margin: auto; display: block; } </style>
Through the above code, we have successfully implemented the quick preview and switching function of images through Vue. Readers can configure and optimize according to their own needs to meet specific project needs. I hope this article can help readers understand and master the use of Vue.
The above is the detailed content of How to implement quick preview and switching functions of images through Vue?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


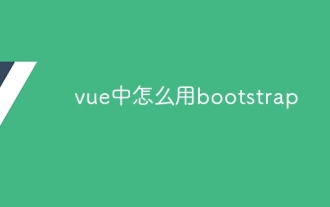
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
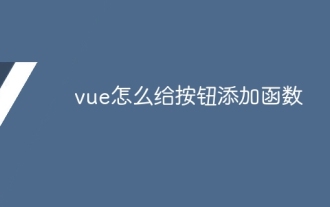
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
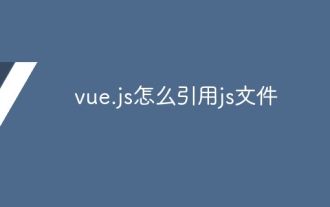
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
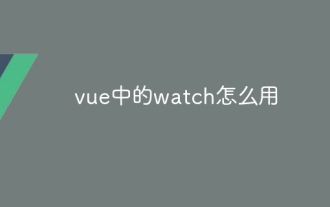
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
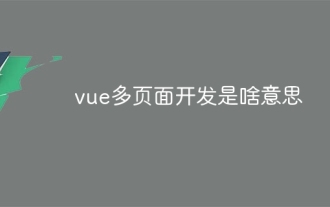
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
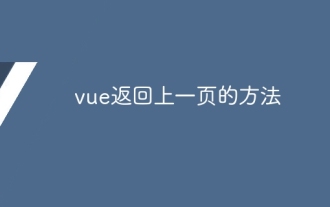
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
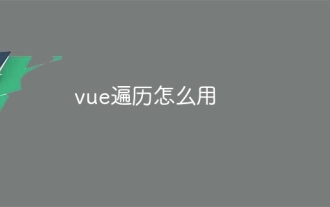
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
