


How to implement drag-and-drop and sorting functions of images through Vue?
How to implement the drag and drop and sorting functions of images through Vue?
Vue, as a popular JavaScript framework, provides powerful functions for handling user interfaces. In this article, we will learn how to use Vue to implement image dragging and sorting functions.
First, we need to install Vue and create a Vue instance. We can install Vue through the following command:
npm install vue
Next, create an HTML file, introduce Vue dependencies, and create a Vue instance:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Drag and Sort Images</title> <script src="https://cdn.jsdelivr.net/npm/vue"></script> </head> <body> <div id="app"> <div class="image-container"> <div class="image-card" v-for="(image, index) in images" :key="index" :style="{left: image.x + 'px', top: image.y + 'px'}" @mousedown="startDrag(index)" @mouseup="stopDrag(index)" @mousemove="drag(index)"> <img src="/static/imghw/default1.png" data-src="image.src" class="lazy" : alt="Image"> </div> </div> </div> <script> new Vue({ el: '#app', data: { images: [ { src: 'image1.jpg', x: 0, y: 0 }, { src: 'image2.jpg', x: 0, y: 0 }, { src: 'image3.jpg', x: 0, y: 0 }, { src: 'image4.jpg', x: 0, y: 0 } ], dragging: false, draggedIndex: -1, initialX: 0, initialY: 0 }, methods: { startDrag(index) { this.dragging = true; this.draggedIndex = index; this.initialX = event.clientX; this.initialY = event.clientY; }, stopDrag(index) { if (this.dragging && this.draggedIndex !== -1) { this.images.splice(index, 0, this.images.splice(this.draggedIndex, 1)[0]); } this.dragging = false; this.draggedIndex = -1; }, drag(index) { if (this.dragging && this.draggedIndex !== -1) { const dx = event.clientX - this.initialX; const dy = event.clientY - this.initialY; this.images[index].x += dx; this.images[index].y += dy; this.initialX = event.clientX; this.initialY = event.clientY; } } } }); </script> </body> </html>
The above code will create a drag and drop Image container with sorting functionality. We use the v-for
directive to iterate through the images
array, and the v-bind
directive to bind the position of each image to the CSS style.
In the data
attribute of the Vue instance, we define an images
array, each element contains src
, x
and y
attributes. The dragging
, draggedIndex
, initialX
, and initialY
properties are used to track the status and initial position of dragging.
startDrag
method sets the drag state when the mouse is pressed and records the initial position.
stopDrag
method stops dragging when the mouse is released, and sorts the array according to the dragged position.
drag
method updates the position of the image when the mouse moves.
The above code implements basic drag-and-drop and sorting functions, but it can be further improved.
We can add restrictions so that the image can only be moved within the container. To this end, we can modify the drag
method as follows:
drag(index) { if (this.dragging && this.draggedIndex !== -1) { const dx = event.clientX - this.initialX; const dy = event.clientY - this.initialY; const container = document.querySelector('.image-container'); const containerRect = container.getBoundingClientRect(); const imageRect = event.target.getBoundingClientRect(); if ( imageRect.left + dx >= containerRect.left && imageRect.right + dx <= containerRect.right && imageRect.top + dy >= containerRect.top && imageRect.bottom + dy <= containerRect.bottom ) { this.images[index].x += dx; this.images[index].y += dy; this.initialX = event.clientX; this.initialY = event.clientY; } } }
In this way, the image will only be dragged and moved within the container range.
Through this simple code example, we learned how to use Vue to implement the drag-and-drop and sorting functions of images. In actual projects, you can improve and expand this function according to your own needs.
The above is the detailed content of How to implement drag-and-drop and sorting functions of images through Vue?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


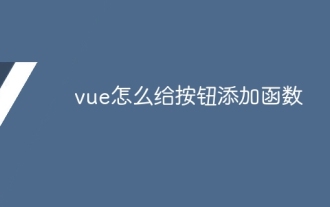
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
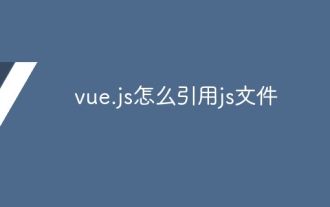
There are three ways to refer to JS files in Vue.js: directly specify the path using the <script> tag;; dynamic import using the mounted() lifecycle hook; and importing through the Vuex state management library.
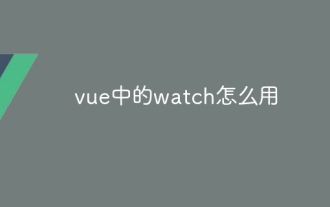
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
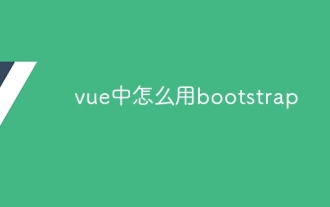
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.
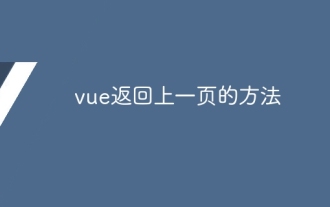
Vue.js has four methods to return to the previous page: $router.go(-1)$router.back() uses <router-link to="/" component window.history.back(), and the method selection depends on the scene.
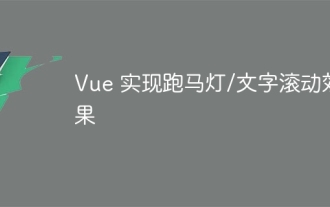
Implement marquee/text scrolling effects in Vue, using CSS animations or third-party libraries. This article introduces how to use CSS animation: create scroll text and wrap text with <div>. Define CSS animations and set overflow: hidden, width, and animation. Define keyframes, set transform: translateX() at the beginning and end of the animation. Adjust animation properties such as duration, scroll speed, and direction.

You can query the Vue version by using Vue Devtools to view the Vue tab in the browser's console. Use npm to run the "npm list -g vue" command. Find the Vue item in the "dependencies" object of the package.json file. For Vue CLI projects, run the "vue --version" command. Check the version information in the <script> tag in the HTML file that refers to the Vue file.
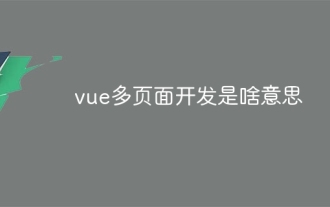
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
