Golang's method of image segmentation and content recognition
Golang’s method of achieving image segmentation and content recognition
With the advancement of artificial intelligence and computer vision technology, image segmentation and content recognition play a role in various fields plays an increasingly important role. This article will introduce how to use Golang to achieve image segmentation and content recognition, and come with code examples.
Before we start, we need to install several necessary Go packages. First, we need to install "github.com/otiai10/gosseract/v2", which is a Golang library for text recognition. Secondly, we also need to install "gonum.org/v1/gonum/mat", which is a Golang library for matrix operations. You can use the following command to install:
1 2 |
|
Next, we will use the following steps to achieve image segmentation and content recognition.
Step 1: Read the image and perform grayscale processing
First, we need to read the image from the file and convert it into a grayscale image. The code example is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
|
In this code, we first open and read an image named "image.jpg". Then, we decode the picture into an image object through the "jpeg.Decode" function. Next, we created a new grayscale image object "gray" and used a double loop to convert the original image to grayscale.
Step 2: Segment the image
After obtaining the grayscale image, we can use some image processing algorithms to segment the image. Here we use the OTSU algorithm for threshold segmentation. The code example is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
|
In this code, we define a function named "otsu" to calculate the threshold of the OTSU algorithm. We then use this function in the "main" function to get the threshold. Next, we create a new binary image "binary" and threshold segment the grayscale image using a double loop.
Step 3: Content identification
After segmenting the image, we can use the "gosseract" library to identify the content of each area. The code example is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
|
In this code, we use the "NewClient" and "Close" functions in the "gosseract" library to create and close the recognition client. We then use a double loop to iterate over the segmented binary images. For non-white areas, we get the coordinate range of the area and convert it into a sub-image. Next, we calculate the proportion of black pixels in the sub-image to remove noise. Finally, we convert the subimage to text via the "ImageToText" function and save the result in the "texts" array.
Through the above steps, we have completed the method of using Golang to achieve image segmentation and content recognition. You can modify and optimize the code according to your own needs to adapt to different scenarios and needs. I hope this article can provide some help for you to understand and apply image segmentation and content recognition technology.
The above is the detailed content of Golang's method of image segmentation and content recognition. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
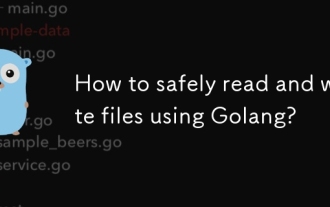
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
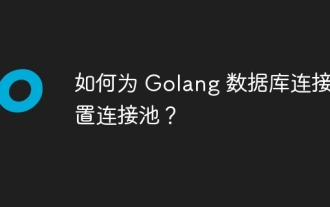
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
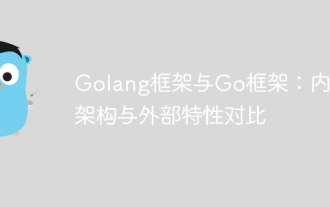
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
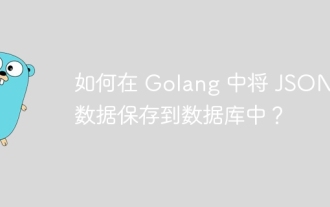
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
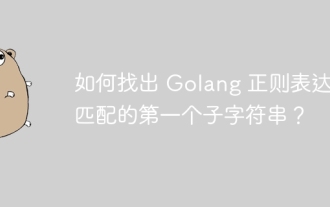
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
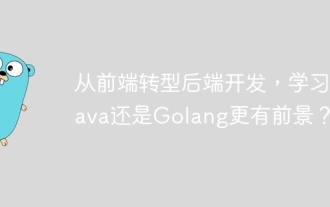
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
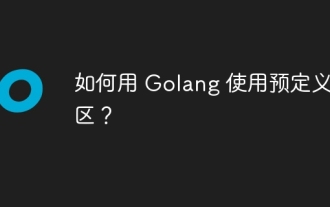
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
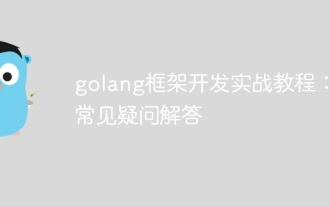
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
