Solution to solve Java database update exception (DatabaseUpdateException)
Solution to Java database update exception (DatabaseUpdateException)
When using Java programming language for database operations, we often encounter database update exception (DatabaseUpdateException) . This kind of exception is usually caused by improper data operation, database connection exception or SQL statement error. This article will introduce some common solutions to handle these exceptions, with corresponding code examples.
1. Check the database connection
Before performing database operations, first ensure that the database connection is normal. If there is a problem with the database connection, an exception will be thrown when executing the SQL statement. You can check whether the database connection is normal by writing a simple method:
public class DatabaseUtil { // ... public static Connection getConnection() { Connection conn = null; try { // 获取数据库连接代码 } catch (SQLException e) { e.printStackTrace(); } return conn; } public static boolean isConnectionValid(Connection conn) { try { return conn.isValid(5000); // 设置超时时间为5秒 } catch (SQLException e) { e.printStackTrace(); } return false; } }
Before performing database operations, you can call the isConnectionValid
method to check whether the database connection is valid.
2. Check the SQL statement
Before executing the SQL statement, be sure to carefully check the correctness of the SQL statement. Common SQL statement errors include syntax errors, table name errors, field name errors, etc. You can check the correctness of the SQL statement by printing it or using database debugging tools.
public class DatabaseUtil { // ... public static void insertData(String name, int age) throws SQLException { Connection conn = null; Statement stmt = null; try { conn = getConnection(); stmt = conn.createStatement(); String sql = "INSERT INTO user (name, age) VALUES ('" + name + "', " + age + ")"; stmt.executeUpdate(sql); } catch (SQLException e) { e.printStackTrace(); throw e; } finally { // 释放资源的代码 } } }
When performing the data insertion operation, you can first print the SQL statement to see if it meets expectations.
3. Handling exceptions
If an exception is thrown during the database update operation, corresponding exception handling should be performed. You can choose to capture and record exceptions, roll back transactions, or close database connections based on specific circumstances.
public class DatabaseUtil { // ... public static void updateData(int id, String name) { Connection conn = null; Statement stmt = null; try { conn = getConnection(); stmt = conn.createStatement(); String sql = "UPDATE user SET name='" + name + "' WHERE id=" + id; stmt.executeUpdate(sql); } catch (SQLException e) { e.printStackTrace(); // 异常处理代码 } finally { // 释放资源的代码 } } }
When updating data, if an exception occurs, corresponding processing can be carried out according to the actual situation, such as logging or rolling back the transaction.
4. Use transactions
When performing database operations, you can use transactions to ensure the atomicity of a set of operations. Transactions can guarantee that multiple database operations either all succeed or all fail. You can use the corresponding transaction management class to complete transaction control.
public class DatabaseUtil { // ... public static void updateDataWithTransaction(int id, String name) throws SQLException { Connection conn = null; Statement stmt = null; try { conn = getConnection(); conn.setAutoCommit(false); stmt = conn.createStatement(); String sql = "UPDATE user SET name='" + name + "' WHERE id=" + id; stmt.executeUpdate(sql); // 其他数据库操作代码 conn.commit(); } catch (SQLException e) { e.printStackTrace(); conn.rollback(); throw e; } finally { // 释放资源的代码 conn.setAutoCommit(true); } } }
When using transactions, you need to call the setAutoCommit(false)
method in the appropriate place to set the automatic commit of the transaction to false
, and call it after the transaction execution is completed commit()
method commits the transaction. If an exception occurs, you can call the rollback()
method to roll back the transaction and continue to throw the exception upward.
In summary, solving Java database update exceptions requires us to carefully check the database connection, SQL statements and perform appropriate exception handling. Reasonable use of transactions can ensure the atomicity of database operations. Through the above solutions and code examples, we hope to help readers better handle database update exceptions.
The above is the detailed content of Solution to solve Java database update exception (DatabaseUpdateException). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
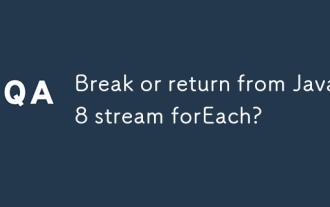
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
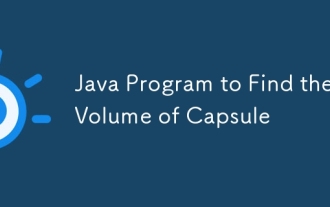
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
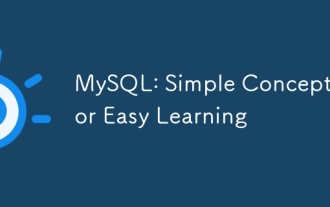
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
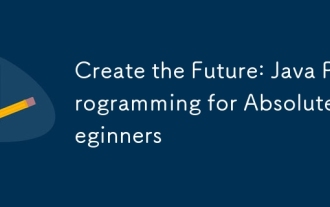
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
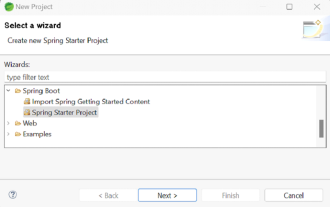
Spring Boot simplifies the creation of robust, scalable, and production-ready Java applications, revolutionizing Java development. Its "convention over configuration" approach, inherent to the Spring ecosystem, minimizes manual setup, allo
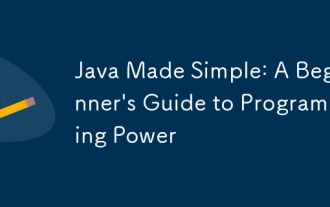
Java Made Simple: A Beginner's Guide to Programming Power Introduction Java is a powerful programming language used in everything from mobile applications to enterprise-level systems. For beginners, Java's syntax is simple and easy to understand, making it an ideal choice for learning programming. Basic Syntax Java uses a class-based object-oriented programming paradigm. Classes are templates that organize related data and behavior together. Here is a simple Java class example: publicclassPerson{privateStringname;privateintage;
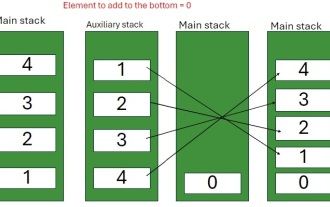
A stack is a data structure that follows the LIFO (Last In, First Out) principle. In other words, The last element we add to a stack is the first one to be removed. When we add (or push) elements to a stack, they are placed on top; i.e. above all the
