Solve PHP error: access to private properties
Solution to PHP error: access to private properties
In the process of PHP programming, we often encounter errors when accessing private properties. Private properties refer to properties defined in a class that can only be accessed within this class. If you try to access private properties outside the class, an error will be reported. In this article, we'll explain how to fix this common problem.
First, let us look at a simple sample code:
class Person { private $name = "John"; public function getName() { return $this->name; } } $person = new Person(); echo $person->name; // 访问私有属性,报错
The above code defines a class named Person, which contains a private property $name and a public method getName. Returns the value of $name. Outside the class, we create a Person object and try to access the private property $name directly. However, such an operation will result in an error.
In order to solve this problem, we can provide a public method inside the class to indirectly access private properties. The modified code is as follows:
class Person { private $name = "John"; public function getName() { return $this->name; } public function setName($newName) { $this->name = $newName; } } $person = new Person(); echo $person->getName(); // 输出 "John" $person->setName("Tom"); echo $person->getName(); // 输出 "Tom"
In the modified code, we added a public method setName, which accepts a parameter $newName for setting the value of the private property $name. Through this method, we can indirectly access and modify private properties outside the class.
In addition to providing methods to get and set private properties, we can also use the magic methods __get and __set to handle access to private properties. The sample code is as follows:
class Person { private $name = "John"; public function __get($property) { if (property_exists($this, $property)) { return $this->$property; } } public function __set($property, $value) { if (property_exists($this, $property)) { $this->$property = $value; } } } $person = new Person(); echo $person->name; // 输出 "John" $person->name = "Tom"; echo $person->name; // 输出 "Tom"
In this example, we use the __get and __set methods to get and set private properties. When accessing a private property, the __get method is called, which first checks whether the property exists and returns its value if it exists. Similarly, when setting a private property, the __set method is called, which also checks whether the property exists and assigns the new value to the private property.
Through the above method, we can solve the problem of accessing private properties. However, we should use these methods with caution, as too many direct accesses or modifications to private properties can break encapsulation, making the code less maintainable.
To summarize, to solve the problem of accessing private properties in PHP errors, we can provide public methods to get and set the value of private properties, or use the magic methods __get and __set to handle access to private properties. Condition. When writing code, we should use these methods reasonably to ensure the encapsulation and maintainability of the code.
The above is the detailed content of Solve PHP error: access to private properties. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


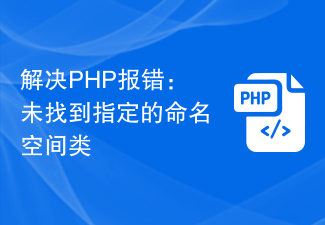
Solve PHP error: The specified namespace class was not found. When developing using PHP, we often encounter various error messages. One of the common errors is "The specified namespace class was not found". This error is usually caused by the imported class file not being properly namespace referenced. This article explains how to solve this problem and provides some code examples. First, let’s take a look at an example of a common error message: Fatalerror:UncaughtError:C
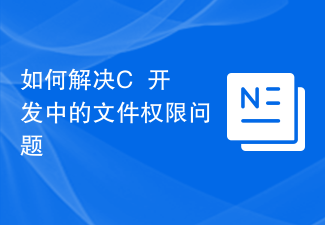
How to solve file permission issues in C++ development During the C++ development process, file permission issues are a common challenge. In many cases, we need to access and operate files with different permissions, such as reading, writing, executing and deleting files. This article will introduce some methods to solve file permission problems in C++ development. 1. Understand file permissions Before solving file permissions problems, we first need to understand the basic concepts of file permissions. File permissions refer to the file's owner, owning group, and other users' access rights to the file. In Li
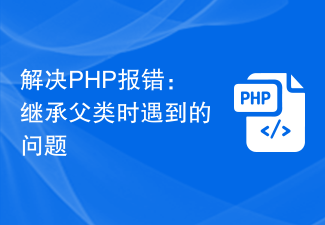
Solving PHP errors: Problems encountered when inheriting parent classes In PHP, inheritance is an important feature of object-oriented programming. Through inheritance, we can reuse existing code and extend and improve it without modifying the original code. Although inheritance is widely used in development, sometimes you may encounter some error problems when inheriting from a parent class. This article will focus on solving common problems encountered when inheriting from a parent class and provide corresponding code examples. Question 1: The parent class is not found. During the process of inheriting the parent class, if the system does not
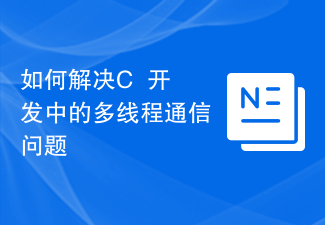
How to solve the multi-threaded communication problem in C++ development. Multi-threaded programming is a common programming method in modern software development. It allows the program to perform multiple tasks at the same time during execution, improving the concurrency and responsiveness of the program. However, multi-threaded programming will also bring some problems, one of the important problems is the communication between multi-threads. In C++ development, multi-threaded communication refers to the transmission and sharing of data or messages between different threads. Correct and efficient multi-thread communication is crucial to ensure program correctness and performance. This article
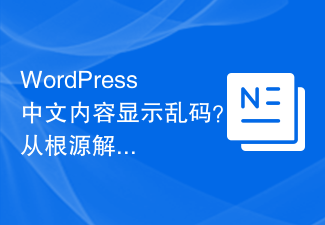
WordPress is a powerful open source content management system that is widely used in website construction and blog publishing. However, in the process of using WordPress, sometimes you encounter the problem of Chinese content displaying garbled characters, which brings troubles to user experience and SEO optimization. Starting from the root cause, this article introduces the possible reasons why WordPress Chinese content displays garbled characters, and provides specific code examples to solve this problem. 1. Cause analysis Database character set setting problem: WordPress uses a database to store the website
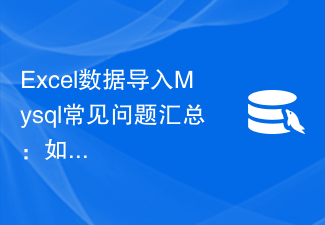
Summary of frequently asked questions about importing Excel data into Mysql: How to solve the problem of field type mismatch? Importing data is a very common operation in database management, and Excel, as a common data processing tool, is usually used for data collection and organization. However, when importing Excel data into a Mysql database, you may encounter field type mismatch problems. This article will discuss this issue and provide some solutions. First, let’s understand the origin of the problem of field type mismatch.
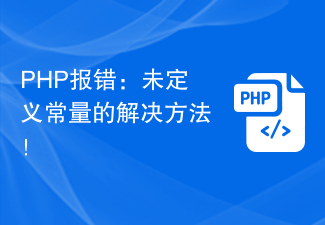
PHP error: Undefined constant solution! In PHP programming, we often encounter constant undefined errors. This error usually occurs when undefined constants are used in the code. This article will introduce the concept of constants and how to solve the problem of undefined constants. First, let's understand what constants are. In PHP, a constant is a value that once defined cannot be changed again. Constants are defined using the define() function. Here's a simple example: <?phpdefine("
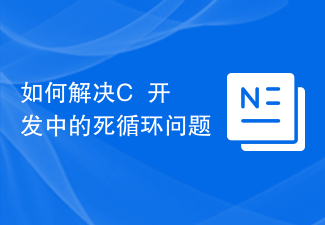
How to solve the infinite loop problem in C++ development. In C++ development, the infinite loop is a very common but very difficult problem. When a program falls into an infinite loop, it will cause the program to fail to execute normally, and may even cause the system to crash. Therefore, solving infinite loop problems is one of the essential skills in C++ development. This article will introduce some common methods to solve the infinite loop problem. Checking Loop Conditions One of the most common causes of endless loops is incorrect loop conditions. When the loop condition is always true, the loop will continue to execute, resulting in an infinite loop.
