Display all Sundays in a given year using Pandas in Python
Pandas is a powerful Python library for data processing and analysis. A key feature of Pandas is its ability to efficiently handle date and time data. In this article, we will show how to use Pandas to display all Sundays in a given year.
In this article, we will explore how to use Pandas, a popular data manipulation library in Python, to display all Sundays in a given year. We'll walk through the process of extracting the Sundays of the year and displaying them in a readable format.
prerequisites
Before you begin, make sure Pandas is installed on your computer. You can install it by running the following command in the terminal -
pip install pandas Getting Started
Using Pandas in Python
First, we will start by importing the Pandas library and create a Pandas DataFrame to store the day of the year. We will use the date_range function to generate a date range of one year. Below is the code to generate the date range in 2023 −
import pandas as pd year = 2023 start_date = pd.to_datetime(f'{year}-01-01') end_date = pd.to_datetime(f'{year}-12-31') dates = pd.date_range(start_date, end_date)
We use the pd.to_datetime function to create a start_date and an end_date object. The dates variable was created using the pd.date_range function, which generates a range of dates from start_date to end_date.
Extract Sunday
To extract Sunday from a date range, we will use the dt accessor provided by Pandas. The dt accessor provides various methods to manipulate date and time values of Pandas DataFrame. We will use the day_name method of the dt accessor to get the day of the week name for each date in the dates DataFrame. Here is the code to extract Sunday:
sundays = dates[dates.dt.day_name() == 'Sunday']
dates.dt.day_name() method returns the day of the week name for each date in the dates DataFrame. Then, we filter the dates DataFrame to only keep rows for Sunday.
Show Sunday
To display Sunday in a readable format, we will use the strftime method of the dt accessor. The strftime method is used to format date and time values of Pandas DataFrame. Here is the code to display Sunday:
for sunday in sundays: print(sunday.strftime('%Y-%m-%d'))
strftime('%Y-%m-%d') method formats the date into YYYY-MM-DD format. We then loop through the sundays DataFrame and print each Sunday in the desired format.
The Chinese translation ofFinal Code
is:Final Code
This is the complete code to display all Sundays in 2023 −
import pandas as pd year = 2023 start_date = pd.to_datetime(f'{year}-01-01') end_date = pd.to_datetime(f'{year}-12-31') dates = pd.date_range(start_date, end_date) sundays = dates[dates.dt.day_name() == 'Sunday'] for sunday in sundays: print(sunday.strftime('%Y-%m-%d'))
Output
DatetimeIndex(['2023-01-01', '2023-01-08', '2023-01-15', '2023-01-22', '2023-01-29', '2023-02-05', '2023-02-12', '2023-02-19', '2023-02-26', '2023-03-05', '2023-03-12', '2023-03-19', '2023-03-26', '2023-04-02', '2023-04-09', '2023-04-16', '2023-04-23', '2023-04-30', '2023-05-07', '2023-05-14', '2023-05-21', '2023-05-28', '2023-06-04', '2023-06-11', '2023-06-18', '2023-06-25', '2023-07-02', '2023-07-09', '2023-07-16', '2023-07-23', '2023-07-30', '2023-08-06', '2023-08-13', '2023-08-20', '2023-08-27', '2023-09-03', '2023-09-10', '2023-09-17', '2023-09-24', '2023-10-01', '2023-10-08', '2023-10-15', '2023-10-22', '2023-10-29', '2023-11-05', '2023-11-12', '2023-11-19', '2023-11-26', '2023-12-03', '2023-12-10', '2023-12-17', '2023-12-24', '2023-12-31'], dtype='datetime64[ns]', freq=None)>
Use Pandas to display all Sundays in a given year
To display all Sundays in a given year, we first need to create a Pandas DataFrame with a date range spanning the entire year. We can then filter this DataFrame to only include Sundays.
This is the Python code to accomplish this task. it's here. Let’s break down the code step by step −
We use the import statement to import the Pandas library.
We use the pd.date_range() function to create a date range that spans the entire year. We specify the start and end dates using the start and end parameters respectively. We replace '2022' with the desired year.
We filter the date range to include only Sundays by using the .weekday property of the date range, which returns the day of the week as an integer (Monday = 0, Tuesday = 1, etc. ). Sunday is represented by the integer 6.
We store the filtered date range in a variable named sundays.
Finally, we print the list of Sundays by calling the print() function on the sundays variable.
import pandas as pd # Replace '2022' with the desired year date_range = pd.date_range(start='1/1/2022', end='12/31/2022') # Filter the date range to only include Sundays sundays = date_range[date_range.weekday == 6] # Print the list of Sundays print(sundays)
Output
When you run the above code, you should see a list of all Sundays in a given year −
DatetimeIndex(['2022-01-02', '2022-01-09', '2022-01-16', '2022-01-23', '2022-01-30', '2022-02-06', '2022-02-13', '2022-02-20', '2022-02-27', '2022-03-06', '2022-03-13', '2022-03-20', '2022-03-27', '2022-04-03', '2022-04-10', '2022-04-17', '2022-04-24', '2022-05-01', '2022-05-08', '2022-05-15', '2022-05-22', '2022-05-29', '2022-06-05', '2022-06-12', '2022-06-19', '2022-06-26', '2022-07-03', '2022-07-10', '2022-07-17', '2022-07-24', '2022-07-31', '2022-08-07', '2022-08-14', '2022-08-21', '2022-08-28', '2022-09-04', '2022-09-11', '2022-09-18', '2022-09-25', '2022-10-02', '2022-10-09', '2022-10-16', '2022-10-23', '2022-10-30', '2022-11-06', '2022-11-13', '2022-11-20', '2022-11-27', '2022-12-04', '2022-12-11', '2022-12-18', '2022-12-25'], dtype='datetime64[ns]', freq=None)
in conclusion
In this article, we explored how to extract and display all Sundays in a given year using Pandas. We used the date_range, dt, and strftime methods of the Pandas library to generate date ranges, extract Sundays, and display them in a readable format. Pandas provides a powerful and flexible way to manipulate date and time values in Python, making it a useful tool for data analysis and visualization.
The above is the detailed content of Display all Sundays in a given year using Pandas in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
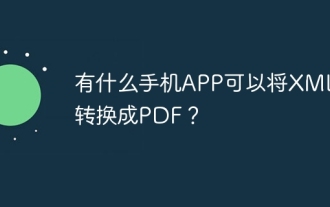
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
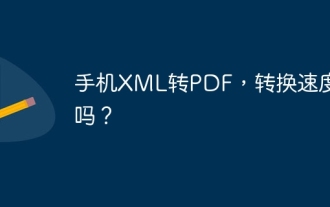
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
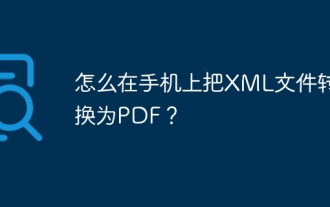
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
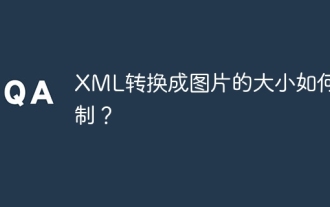
To generate images through XML, you need to use graph libraries (such as Pillow and JFreeChart) as bridges to generate images based on metadata (size, color) in XML. The key to controlling the size of the image is to adjust the values of the <width> and <height> tags in XML. However, in practical applications, the complexity of XML structure, the fineness of graph drawing, the speed of image generation and memory consumption, and the selection of image formats all have an impact on the generated image size. Therefore, it is necessary to have a deep understanding of XML structure, proficient in the graphics library, and consider factors such as optimization algorithms and image format selection.
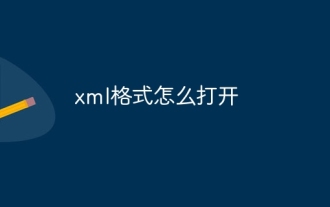
Use most text editors to open XML files; if you need a more intuitive tree display, you can use an XML editor, such as Oxygen XML Editor or XMLSpy; if you process XML data in a program, you need to use a programming language (such as Python) and XML libraries (such as xml.etree.ElementTree) to parse.
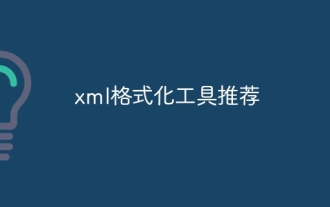
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
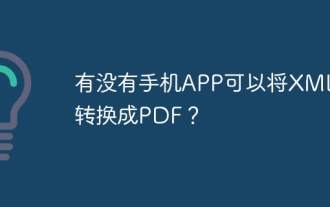
There is no APP that can convert all XML files into PDFs because the XML structure is flexible and diverse. The core of XML to PDF is to convert the data structure into a page layout, which requires parsing XML and generating PDF. Common methods include parsing XML using Python libraries such as ElementTree and generating PDFs using ReportLab library. For complex XML, it may be necessary to use XSLT transformation structures. When optimizing performance, consider using multithreaded or multiprocesses and select the appropriate library.
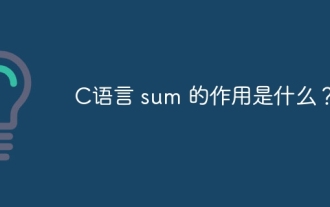
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
