


How to use PHP to achieve lossless compression and optimization of images
How to use PHP to achieve lossless compression and optimization of images
Introduction:
In web development, images are an indispensable part. However, image files that are too large will cause the web page to load slowly and affect the user experience. In order to solve this problem, we can use PHP to achieve lossless compression and optimization of images. This article will introduce how to use PHP to implement this function and provide corresponding code examples.
-
Installing and configuring the GD library
The GD library is a library for processing graphics images in PHP. Before starting, we need to make sure that the GD library is installed on the server. You can check whether the GD library is installed by running the following command:<?php if(function_exists('gd_info')) { echo 'GD库已安装'; } else { echo 'GD库未安装'; }
Copy after loginIf the output result is "GD library has been installed", it means that the GD library has been installed. If the output result is "GD library not installed", you need to install the GD library.
Compress images
The following code example shows how to use the GD library to compress images. We can limit the size of the image by specifying the maximum width and height, and set the compression quality to reduce the image file size.<?php function compressImage($source, $destination, $maxWidth, $maxHeight, $quality) { $info = getimagesize($source); $width = $info[0]; $height = $info[1]; // 计算缩放比例 $scale = min($maxWidth/$width, $maxHeight/$height); // 计算缩放后的宽度和高度 $newWidth = $width * $scale; $newHeight = $height * $scale; // 创建缩略图 $thumb = imagecreatetruecolor($newWidth, $newHeight); // 根据原图类型进行相应的处理 if ($info['mime'] == 'image/jpeg') { $sourceImage = imagecreatefromjpeg($source); } elseif ($info['mime'] == 'image/png') { $sourceImage = imagecreatefrompng($source); } elseif ($info['mime'] == 'image/gif') { $sourceImage = imagecreatefromgif($source); } else { return false; } // 将原图复制到缩略图中 imagecopyresampled($thumb, $sourceImage, 0, 0, 0, 0, $newWidth, $newHeight, $width, $height); // 保存缩略图 if ($info['mime'] == 'image/jpeg') { imagejpeg($thumb, $destination, $quality); } elseif ($info['mime'] == 'image/png') { imagepng($thumb, $destination, 9); } elseif ($info['mime'] == 'image/gif') { imagegif($thumb, $destination); } return true; } // 使用示例 $sourceImage = 'source.jpg'; $destinationImage = 'compressed.jpg'; $maxWidth = 800; $maxHeight = 600; $quality = 75; compressImage($sourceImage, $destinationImage, $maxWidth, $maxHeight, $quality);
Copy after loginIn the above code example, the
compressImage
function accepts the source file path, target file path, maximum width, maximum height and compression quality of the image as parameters. It first obtains the size information of the original image, then calculates the scaling ratio based on the maximum width and height, and calculates the scaled width and height. After that, the function creates a thumbnail image and performs corresponding processing according to the type of the original image. Finally, the thumbnail is saved to the target file with the specified compression quality.- Optimize images
In addition to compressing images, other methods can be used to further optimize images. The following are some common optimization methods: Use transparent images: If there are transparent parts in the picture, you can save it in transparent PNG format instead of JPEG format.
<?php $sourceImage = 'source.png'; $destinationImage = 'transparent.png'; compressImage($sourceImage, $destinationImage, $maxWidth, $maxHeight, $quality);
Copy after loginDelete picture metadata: Some picture files contain a lot of metadata, such as shooting time, device information, etc. We can use the following code to remove metadata from the image:
<?php function deleteMetadata($source, $destination) { $info = getimagesize($source); // 根据原图类型进行相应的处理 if ($info['mime'] == 'image/jpeg') { $sourceImage = imagecreatefromjpeg($source); imagejpeg($sourceImage, $destination); } elseif ($info['mime'] == 'image/png') { $sourceImage = imagecreatefrompng($source); imagepng($sourceImage, $destination, 9); } elseif ($info['mime'] == 'image/gif') { $sourceImage = imagecreatefromgif($source); imagegif($sourceImage, $destination); } return true; } // 使用示例 $sourceImage = 'source.jpg'; $destinationImage = 'optimized.jpg'; deleteMetadata($sourceImage, $destinationImage);
Copy after loginUse WebP format: WebP is an image format that supports lossless and lossy compression, usually better than JPEG and PNG formats Picture files are smaller. You can use the following code to save images in WebP format:
<?php function saveWebp($source, $destination) { $info = getimagesize($source); // 根据原图类型进行相应的处理 if ($info['mime'] == 'image/jpeg') { $sourceImage = imagecreatefromjpeg($source); imagewebp($sourceImage, $destination); } elseif ($info['mime'] == 'image/png') { $sourceImage = imagecreatefrompng($source); imagewebp($sourceImage, $destination); } elseif ($info['mime'] == 'image/gif') { $sourceImage = imagecreatefromgif($source); imagewebp($sourceImage, $destination); } return true; } // 使用示例 $sourceImage = 'source.jpg'; $destinationImage = 'optimized.webp'; saveWebp($sourceImage, $destinationImage);
Copy after loginSummary:
By using PHP and GD libraries, we can easily achieve lossless compression and optimization of images. In addition to compressing images, you can also use other optimization methods to reduce the size of image files and improve web page loading speed, thereby improving user experience. The above code examples show you how to implement these functions. Please choose the appropriate method and code to use according to your needs.
The above is the detailed content of How to use PHP to achieve lossless compression and optimization of images. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
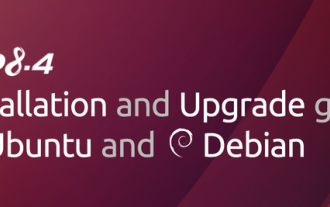
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
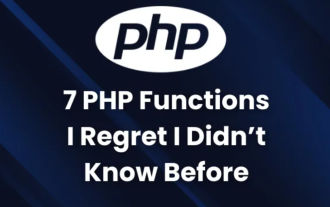
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
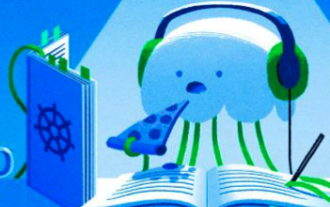
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
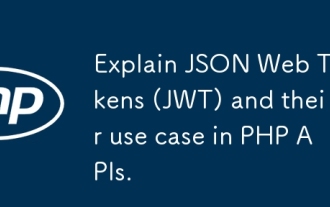
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
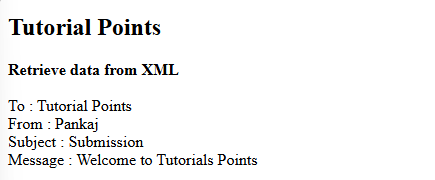
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
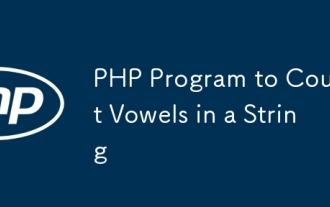
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
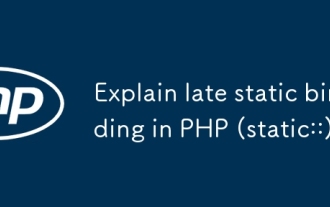
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
