How to solve the code modularization problem in C++ development
How to solve the code modularization problem in C development
For C developers, code modularization is a common problem. As projects increase in size and complexity, code modularization becomes even more important to improve code maintainability, reusability, and testability. This article will introduce some methods and techniques to help C developers solve code modularization problems.
- Using namespaces
Namespaces are a way in C to organize related code together. By using namespaces, you can separate different functions or modules, avoid naming conflicts, and improve code readability. For example, you can place code related to file input and output in a namespace called "io".
1 2 3 4 |
|
- Dividing classes and functions
Putting related code into classes and functions is another way to modularize your code. By organizing code with similar functionality into classes, you can improve code reusability and maintainability. For example, you can create a class called "Math" and put math-related functions in it.
1 2 3 4 5 6 7 8 |
|
- Using header files
Header files are commonly used tools in C development, which can put public function, class and data structure declarations together. By using header files, you can easily reference modular code and reduce repeated code writing. In the header file, you can define the interfaces of related classes and functions and reference them in other files through the #include statement.
1 2 3 4 5 6 7 8 9 10 11 12 |
|
1 2 3 4 5 6 |
|
- Use modular development tools
In addition to the above methods, you can also use modular development tools to solve code modularization problems. For example, you can use CMake to manage the modularity of your project. Through the CMake configuration file, different code files, library files and dependencies can be combined together to generate executable files or library files.
1 2 3 4 5 6 7 8 9 10 11 12 |
|
Summary
By using namespaces, dividing classes and functions, using header files, and using modular development tools, C developers can effectively solve code modularization problems. These methods and techniques can not only improve the maintainability, reusability and testability of the code, but also improve development efficiency and team collaboration. When developing C, we should always pay attention to code modularization to avoid code bloat and confusion, and improve development quality and efficiency.
The above is the detailed content of How to solve the code modularization problem in C++ development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




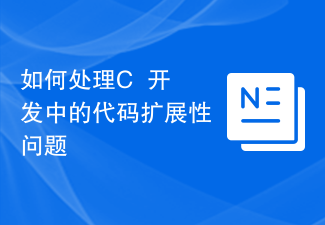
How to deal with the code scalability problem in C++ development. As software becomes increasingly complex and requirements continue to change, code scalability has become an issue that cannot be ignored in software development. Especially in C++ development, the problem of code scalability is more prominent. This article will introduce some methods and techniques for dealing with code scalability issues in C++ development. Using the Principles of Object-Oriented Programming (OOP) Object-oriented programming is a programming paradigm that encapsulates data and operations, which can improve the maintainability and scalability of your code. In C++, I
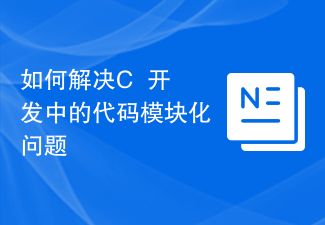
How to solve the code modularization problem in C++ development For C++ developers, code modularization is a common problem. As projects increase in size and complexity, code modularization becomes even more important to improve code maintainability, reusability, and testability. This article will introduce some methods and techniques to help C++ developers solve code modularization problems. Using Namespaces Namespaces are a way of organizing related code together in C++. By using namespaces, different functions or modules can be separated
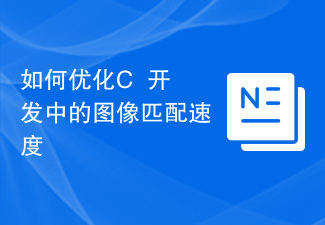
How to optimize image matching speed in C++ development Introduction: With the continuous development of image processing technology, image matching plays an important role in the fields of computer vision and image recognition. In C++ development, how to optimize image matching speed has become a key issue. This article will introduce some techniques to improve image matching speed through algorithm optimization, multi-threading technology and hardware acceleration. 1. Algorithm Optimization Feature Extraction Algorithm Selection In image matching, feature extraction is a key step. Choosing a feature extraction algorithm suitable for the target scene can greatly
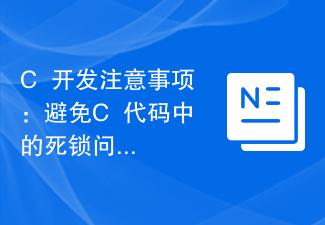
C++ Development Notes: Avoiding Deadlock Problems in C++ Code Introduction: In C++ development, deadlock (Deadlock) is a very common problem, which can lead to serious consequences such as unresponsiveness and crash of the program. Therefore, when we write C++ code, we must pay special attention to avoiding deadlock. This article will introduce some common deadlock problems and how to avoid deadlock in C++ code. 1. What is deadlock? Deadlock means that two or more processes (threads) are waiting for each other's resources, resulting in the inability to continue execution.
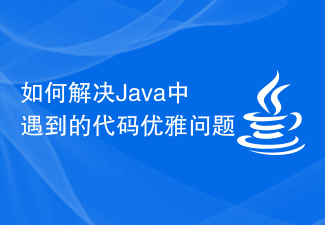
How to solve code elegance problems encountered in Java Code elegance is the goal pursued by every programmer. An elegant code can not only improve the readability and maintainability of the code, but also improve development efficiency and code quality. In Java development, we often encounter some code elegance problems. This article will explore how to solve these problems. Reasonable use of naming conventions Naming is very important when writing Java code. Reasonable naming conventions can make code more readable and better express the intent of the code. we can follow
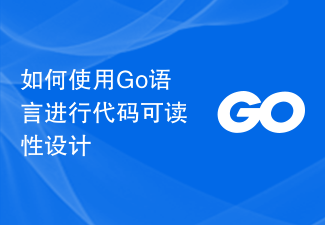
How to use Go language for code readability design In modern software development, code readability is crucial. Well-readable code not only improves the efficiency of teamwork, but also reduces the cost of maintaining the code. Go language is a concise, elegant and easy-to-read programming language that provides some features and conventions to help developers better design code with good readability. This article will introduce how to use Go language to design code readability and illustrate it through sample code. Use meaningful variable and function names in Go
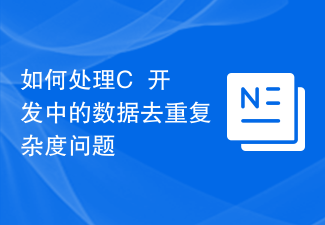
How to deal with the complexity of data deduplication in C++ development. In C++ development, we often encounter the problem of data deduplication. Data deduplication is a common task, especially when large amounts of data are involved. However, data deduplication often faces complexity problems. This article will introduce some methods to deal with the complexity of data deduplication in C++ development. First of all, it is very important to understand the complexity of data deduplication. The complexity of data deduplication usually depends on two factors: the size of the data collection and the uniqueness of the data elements.
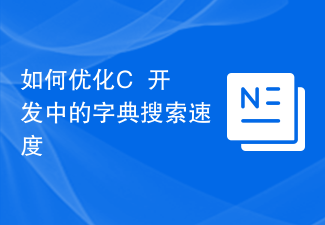
How to Optimize Dictionary Search Speed in C++ Development Summary: Using dictionaries for data search is a common task in C++ development. However, as the amount of data in the dictionary increases, the efficiency of the search may decrease. This article will introduce some methods to optimize dictionary search speed in C++ development, including the selection of data structures, optimization of algorithms, and the application of parallel processing. Introduction: In most applications, fast search of data is critical. In C++ development, we usually use dictionaries to store and retrieve data. However
