Dynamic programming algorithm in C++ and its application skills
Dynamic Programming (DP) is an efficient algorithm used to solve some problems with overlapping sub-problems and optimal sub-structure properties. There are some techniques to improve efficiency when implementing dynamic programming algorithms in C language. This article will introduce the dynamic programming algorithm in C and its application techniques.
The main idea of the dynamic programming algorithm is to decompose the problem into a series of sub-problems, and when solving each sub-problem, retain a state and use this state to avoid repeated calculations. The dynamic programming algorithm can solve some computationally expensive problems because it only needs to calculate each subproblem once instead of every time.
- Three elements of dynamic programming
The dynamic programming algorithm needs to meet three elements:
(1) Optimal substructure: the optimal substructure of the problem The optimal solution contains the optimal solutions to its subproblems.
(2) No aftereffect: All states in the process are only related to the current state and have nothing to do with the previous state.
(3) Overlapping sub-problems: Multiple sub-problems overlap each other, which can avoid repeated calculations.
- Basic classification of dynamic programming
There are two basic classifications of dynamic programming: one is state-based dynamic programming, and the other is decision-based dynamic programming. State-based dynamic programming refers to saving the solutions to each sub-problem during calculation, and then calculating the solution to the larger problem based on the values of these solutions. The state is usually saved using a data structure, such as an array. Decision-based dynamic programming refers to determining the optimal solution to the larger problem based on the optimal solution of each sub-problem during calculation. This method is often used to solve optimization problems or when calculating the minimum value.
- Application skills of dynamic programming
When implementing the dynamic programming algorithm in C, there are some application skills that can improve efficiency. These techniques include:
(1) Use constants instead of array subscripts: In some dynamic programming problems, multiple accesses to the array are required. At this time, you can replace the subscript of the array with a constant, which can speed up access. For example:
for(int i=0;i<n;i++){ for(int j=0;j<m;j++){ dp[i][j] = max(dp[i-1][j],dp[i][j-1])+1; } }
You can use variable k to replace the subscript of the dp array:
for(int k=2;k<=n+m;k++){ for(int i=1;i<=n;i++){ int j = k-i; if(j<1 || j>m) continue; dp[i][j] = max(dp[i-1][j],dp[i][j-1])+1; } }
(2) Optimize the array: In some dynamic programming problems, the size of the array is very large, which may cause memory limitations . At this time, you can use a rolling array or the first dimension of a two-dimensional array to save the intermediate results. For example:
int dp[N][M]; for(int i=0;i<N;i++){ for(int j=0;j<M;j++){ dp[i][j] = max(dp[i-1][j],dp[i][j-1])+1; } }
can be optimized to:
int dp[2][M]; for(int i=0;i<N;i++){ int cur = i%2, pre = (i+1)%2; for(int j=0;j<M;j++){ dp[cur][j] = max(dp[pre][j],dp[cur][j-1])+1; } }
(3) Saving space: In some dynamic programming problems, only the most recent states need to be saved instead of the entire array. At this point, you can use a scrolling array to save only the most recent states.
(4) Avoid repeated calculations: In some dynamic programming problems, there may be repeated sub-problems. At this time, you can use memorized search or bottom-up dynamic programming to avoid repeated calculations.
- Examples of dynamic programming
The following are some examples of dynamic programming problems:
(1) Fibonacci Sequence: Fibonacci A sequence means that starting from 0 and 1, each number is equal to the sum of the previous two numbers. For example, 0, 1, 1, 2, 3, 5, 8, 13, 21.
The recursion formula is: f[n] = f[n-1] f[n-2]
Using dynamic programming algorithm, the following can be achieved:
int dp[N]; dp[0] = 0; dp[1] = 1; for(int i=2;i<=n;i++){ dp[i] = dp[i-1] + dp[i-2]; }
(2) Knapsack problem: The knapsack problem means that there are N items, each item has a weight and a value. Given the capacity C of a knapsack, find the maximum value that can be loaded without exceeding the capacity of the knapsack.
Using dynamic programming algorithm, you can achieve the following:
int dp[N][C]; for(int i=0;i<N;i++){ for(int j=0;j<C;j++){ dp[i][j] = 0; } } for(int i=0;i<N;i++){ for(int j=0;j<=C;j++){ if(j>=w[i]){ dp[i][j] = max(dp[i-1][j],dp[i-1][j-w[i]]+v[i]); } else{ dp[i][j] = dp[i-1][j]; } } }
The above is a brief introduction to dynamic programming algorithm and its application skills in C. For complex dynamic programming problems, time complexity and space complexity also need to be considered. Therefore, when implementing a dynamic programming algorithm, it is necessary to consider various factors and choose an appropriate method.
The above is the detailed content of Dynamic programming algorithm in C++ and its application skills. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


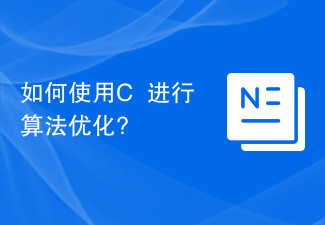
How to use C++ for algorithm optimization? Overview: In the field of computer science, algorithm optimization is a key process to improve algorithm efficiency and performance. An important aspect of writing algorithms in C++ is understanding how to optimize the algorithm to reduce time and space complexity. This article will introduce some available techniques and strategies to help developers implement efficient algorithms in C++. 1. Choose the right data structure: Choosing the right data structure is crucial to the efficiency of the algorithm. Different data structures have different time complexities for search, insertion, and deletion operations. For example
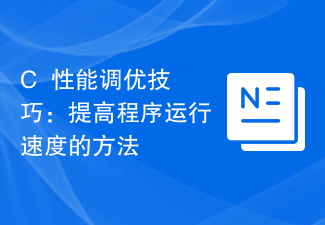
C++ performance tuning tips: Methods to improve program running speed Summary: When developing software, program performance is a crucial factor. Good performance can improve user experience and enhance the competitiveness of software. This article will introduce some C++ performance tuning techniques to help developers improve the running speed of their programs. Introduction: In the actual software development process, we often encounter situations where we need to improve the running speed of the program. Whether it is to speed up calculations, reduce latency, or improve system throughput, performance tuning is a critical link.
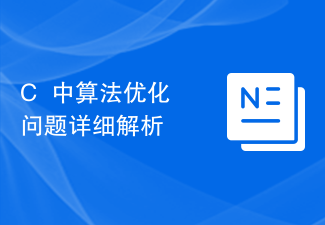
Detailed analysis of algorithm optimization issues in C++ Introduction: In the field of programming, algorithm optimization is a very important task. An efficient algorithm can effectively save time and space resources and improve program performance. As a high-level programming language, C++ provides a wealth of tools and techniques to optimize algorithms. This article will analyze the algorithm optimization issues in C++ in detail and provide specific code examples. 1. Select the appropriate data structure Choosing the appropriate data structure is the first step in optimizing the algorithm. In C++, there are a variety of data structures to choose from, such as
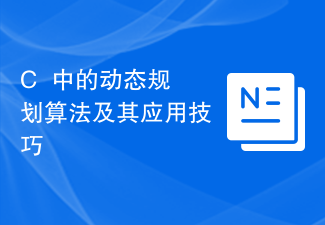
Dynamic Programming (DP) is an efficient algorithm used to solve some problems with overlapping sub-problems and optimal sub-structure properties. There are some techniques to improve efficiency when implementing dynamic programming algorithms in C++ language. This article will introduce the dynamic programming algorithm and its application techniques in C++. The main idea of the dynamic programming algorithm is to decompose the problem into a series of sub-problems, and when solving each sub-problem, retain a state and use this state to avoid repeated calculations. Dynamic programming algorithms can
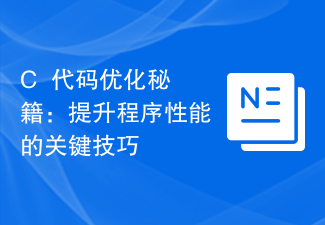
C++ is a high-level programming language and one of the preferred languages chosen by many software engineers and programmers. Although C++ provides powerful functions and flexibility, if you do not pay attention to code optimization, it may cause the program to run inefficiently. This article will share some key techniques to improve the performance of C++ programs, hoping to help readers write code more efficiently. Avoid unnecessary function calls: In C++, function calls have a certain overhead, especially for frequently called functions. Therefore, unnecessary function calls should be avoided as much as possible, you can
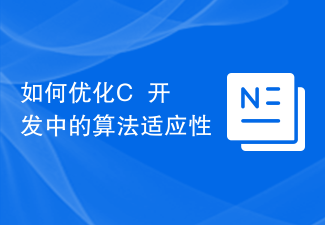
How to optimize algorithm adaptability in C++ development Summary: In C++ development, optimizing algorithm adaptability is crucial to improving program efficiency and performance. This article will introduce some methods and techniques that can help developers optimize the adaptability of algorithms and improve program execution efficiency and performance. Keywords: C++ development; algorithm adaptability; program efficiency; performance optimization Introduction In C++ development, algorithms are the core of realizing various functions and solving various problems. The adaptability of the optimization algorithm can improve the execution efficiency and performance of the program, making the program more efficient and stable.
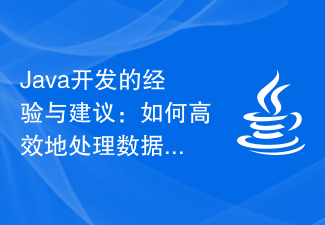
Java development is one of the most popular programming languages at present. Its power lies in its rich data structure and algorithm library. However, for developers who are just getting started or want to improve themselves, how to efficiently handle data structures and algorithms is still a challenge. This article will share with you my experience and suggestions in Java development, I hope it will be helpful to everyone. First, it is very important to understand common data structures and algorithms. Java has built-in many commonly used data structures and algorithms, such as arrays, linked lists, stacks, and queues.
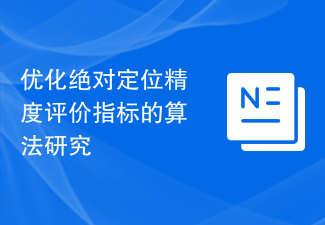
Research on Algorithm Optimization Based on Absolute Positioning Accuracy Evaluation Index Abstract: This article aims at the absolute positioning accuracy evaluation index in the positioning system and improves the accuracy and stability of the positioning system through algorithm optimization methods. First, the absolute positioning accuracy evaluation index is introduced and analyzed in detail. Then, in view of the shortcomings of the evaluation indicators, a targeted algorithm optimization method is proposed, and the effectiveness of the algorithm optimization is proved through experiments. Finally, specific code examples are given to help readers better understand the implementation process of the algorithm. Keywords: absolute positioning, accuracy
