How to deal with code optimization issues in C++ development
How to deal with code optimization issues in C development
In the C development process, code optimization is one of the key factors to improve program performance. Code optimization can make programs execute faster, occupy less memory, and reduce resource consumption. Therefore, knowing how to deal with code optimization issues in C development is an essential skill that every developer should master.
The following are some practical strategies for dealing with code optimization issues in C development:
- Algorithm optimization: Choosing the optimal algorithm is very important to improve program performance. When selecting an algorithm, the time complexity and space complexity of the algorithm should be considered. If there are multiple algorithms that can solve the same problem, the algorithm with lower time complexity should be chosen.
- Compiler optimization: Different compilers optimize code to different degrees. Compiling code with optimization options can improve program execution efficiency. For example, use the -O2 or -O3 option to enable the compiler's optimizations.
- Reduce the number of function calls: Function calls have overhead, so reducing the number of function calls can improve program performance. This can be achieved by embedding some functionally similar code directly into the main function rather than through function calls.
- Use inline functions: Inline functions can reduce the overhead of function calls. Declaring a function as inline allows the compiler to insert the function's code directly into the location where the function is called at compile time, thus avoiding the overhead of a function call.
- Avoid frequent memory allocation and release: Frequent memory allocation and release will lead to the generation of memory fragmentation, thereby reducing program performance. You can improve program performance by using techniques such as object pooling, caching, and reusing objects to reduce the number of memory allocations and releases.
- Use appropriate data structures: Choosing appropriate data structures is very important to improve the performance of your program. For example, using a hash table to perform search operations can improve search efficiency; using arrays to store data can reduce memory overhead; using linked lists to manage dynamic data can improve the efficiency of insertion and deletion.
- Reduce memory usage: Reducing memory usage can improve program performance. Memory usage can be reduced by techniques such as reducing the size of arrays and objects and using bitwise operations instead of multiplication and division.
- Multi-threaded programming: Using multi-threads can improve the concurrency of the program, thereby improving the execution efficiency of the program. In multi-threaded programming, you need to pay attention to thread synchronization and mutual exclusion to avoid data competition and deadlock problems.
- Use efficient IO operations: When performing file reading and writing and network communication, using efficient IO operations can improve program performance. You can use buffers, asynchronous IO and other technologies to improve the efficiency of IO operations.
- Use tools for code analysis: Using tools for code analysis can identify performance problems in the program and make targeted optimizations. For example, you can use the profiler tool to find out the hot code in the program and optimize it accordingly.
In short, in C development, code optimization is one of the key factors to improve program performance. By selecting the optimal algorithm, using appropriate data structures, reducing the number of function calls, optimizing memory usage and other techniques, the execution efficiency of the program can be improved. At the same time, using appropriate tools for code analysis and following programming best practices are also important means of optimizing code. Only through continuous learning and practice can you master the skills of dealing with code optimization issues in C development.
The above is the detailed content of How to deal with code optimization issues in C++ development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


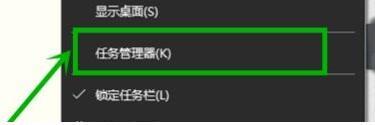
1. First, we right-click the blank space of the taskbar and select the [Task Manager] option, or right-click the start logo, and then select the [Task Manager] option. 2. In the opened Task Manager interface, we click the [Services] tab on the far right. 3. In the opened [Service] tab, click the [Open Service] option below. 4. In the [Services] window that opens, right-click the [InternetConnectionSharing(ICS)] service, and then select the [Properties] option. 5. In the properties window that opens, change [Open with] to [Disabled], click [Apply] and then click [OK]. 6. Click the start logo, then click the shutdown button, select [Restart], and complete the computer restart.
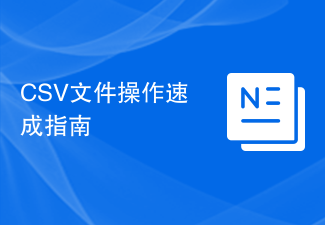
Quickly learn how to open and process CSV format files. With the continuous development of data analysis and processing, CSV format has become one of the widely used file formats. A CSV file is a simple and easy-to-read text file with different data fields separated by commas. Whether in academic research, business analysis or data processing, we often encounter situations where we need to open and process CSV files. The following guide will show you how to quickly learn to open and process CSV format files. Step 1: Understand the CSV file format First,
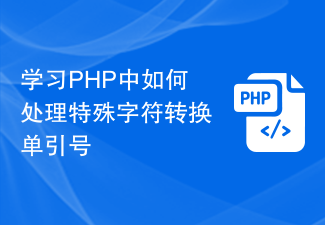
In the process of PHP development, dealing with special characters is a common problem, especially in string processing, special characters are often escaped. Among them, converting special characters into single quotes is a relatively common requirement, because in PHP, single quotes are a common way to wrap strings. In this article, we will explain how to handle special character conversion single quotes in PHP and provide specific code examples. In PHP, special characters include but are not limited to single quotes ('), double quotes ("), backslash (), etc. In strings
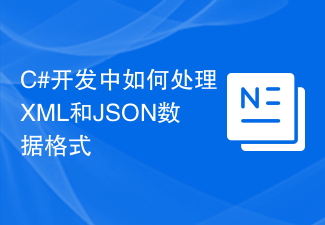
How to handle XML and JSON data formats in C# development requires specific code examples. In modern software development, XML and JSON are two widely used data formats. XML (Extensible Markup Language) is a markup language used to store and transmit data, while JSON (JavaScript Object Notation) is a lightweight data exchange format. In C# development, we often need to process and operate XML and JSON data. This article will focus on how to use C# to process these two data formats, and attach
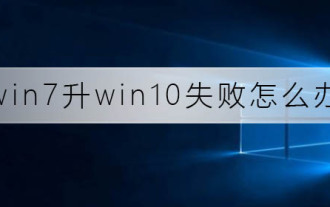
If the operating system we use is win7, some friends may fail to upgrade from win7 to win10 when upgrading. The editor thinks we can try upgrading again to see if it can solve the problem. Let’s take a look at what the editor did for details~ What to do if win7 fails to upgrade to win10. Method 1: 1. It is recommended to download a driver first to evaluate whether your computer can be upgraded to Win10. 2. Then use the driver test after upgrading. Check if there are any driver abnormalities, and then fix them with one click. Method 2: 1. Delete all files under C:\Windows\SoftwareDistribution\Download. 2.win+R run "wuauclt.e
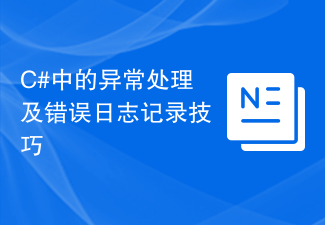
Exception handling and error logging skills in C# Introduction: In the software development process, exception handling and error logging are very important links. For C# developers, mastering exception handling skills and error logging methods can help us better track and debug code, and improve the stability and maintainability of the program. This article will introduce commonly used exception handling techniques in C# and provide specific code examples to help readers better understand and apply exception handling and error logging. 1. Basic concepts of exception handling Exceptions refer to the
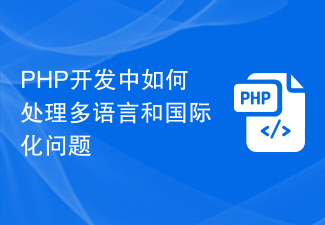
How to deal with multi-language and internationalization issues in PHP development requires specific code examples. With the development of the Internet, people's demand for multi-language and internationalization is getting higher and higher. In PHP development, how to effectively handle multi-language and internationalization issues has become an important task that developers need to solve. Handling of character encoding In PHP development, we must first ensure that character encoding is handled correctly. In multi-language environments, using UTF-8 encoding is the most common choice. You can add the following code to the head of the PHP file: header('C
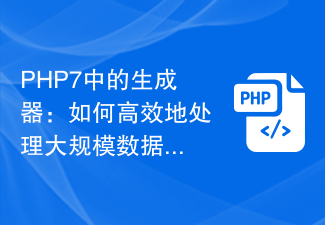
Generators in PHP7: How to handle large-scale data efficiently and save memory? Overview: PHP7 introduces generators as a powerful tool in terms of large-scale data processing and memory saving. Generators are a special type of function in the PHP language. Unlike ordinary functions, generators can pause execution and return intermediate results instead of returning all results at once. This makes the generator ideal for processing large batches of data, reducing memory usage and improving processing efficiency. This article will introduce students
