How to deal with data normalization issues in C++ development
How to deal with data normalization issues in C development
In C development, we often need to process various types of data, which often have different Value range and distribution characteristics. To use this data more efficiently, we often need to normalize it. Data normalization is a data processing technique that maps data of different scales to the same scale range. In this article, we will explore how to deal with data normalization issues in C development.
The purpose of data normalization is to eliminate the dimensional influence between data and map the data to the same range. Common data normalization methods include max-min normalization and standardized normalization.
Maximum-minimum normalization is to linearly map the data to the [0, 1] interval. Suppose we have a data set D={x1, x2, x3, ..., xn}, where xi is the value of the i-th sample. The formula of maximum-minimum normalization is as follows:
x' = (x - min(D)) / (max(D) - min(D))
where x' is the normalized Unified data. Max-min normalization is suitable when there is a priori knowledge of the distribution range of the data.
Standardization Normalization maps data to a distribution with mean 0 and variance 1. The formula for standardized normalization is as follows:
x' = (x - μ) / σ
where x' is the normalized data, μ is the mean of the data, and σ is the data standard deviation. Normalization is suitable when there is no a priori knowledge of the distribution range of the data.
In C, we can use various libraries to implement data normalization. For example, in the OpenCV library, you can use the normalize function to achieve max-min normalization. The sample code is as follows:
#include <opencv2/opencv.hpp> int main() { cv::Mat data; // 假设data是一个n×m的矩阵,每一行代表一个样本 cv::Mat normalizedData; cv::normalize(data, normalizedData, 0, 1, cv::NORM_MINMAX); // 对normalizedData进行后续处理 // ... return 0; }
In the above code, the normalize function normalizes each element in the data matrix to the [0, 1] interval and stores the result in normalizedData.
In addition, you can also use the numerical calculation library Eigen to achieve data normalization. The sample code is as follows:
#include <Eigen/Core> #include <Eigen/Dense> int main() { Eigen::MatrixXd data; // 假设data是一个n×m的矩阵,每一行代表一个样本 Eigen::MatrixXd normalizedData; // 计算每一列的均值和标准差 Eigen::VectorXd mean = data.colwise().mean(); Eigen::VectorXd std = ((data.rowwise() - mean.transpose()).array().square().colwise().sum() / (data.rows() - 1)).sqrt(); // 对data矩阵进行标准化 normalizedData = (data.rowwise() - mean.transpose()).array().rowwise() / std.transpose().array(); // 对normalizedData进行后续处理 // ... return 0; }
In the above code, we first calculate the mean and standard deviation of each column of the data matrix, and then use these statistics to standardize the data.
It should be noted that in actual applications, we usually only normalize the training data, and then use the same normalization parameters to process the test data to ensure the consistency of the model.
To sum up, data normalization is an important task in C development. With appropriate normalization methods and library functions, we can better handle data of different scales and improve the performance and accuracy of the model. I hope this article can provide some help to readers on data normalization issues in C development.
The above is the detailed content of How to deal with data normalization issues in C++ development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


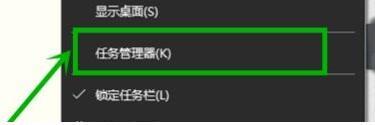
1. First, we right-click the blank space of the taskbar and select the [Task Manager] option, or right-click the start logo, and then select the [Task Manager] option. 2. In the opened Task Manager interface, we click the [Services] tab on the far right. 3. In the opened [Service] tab, click the [Open Service] option below. 4. In the [Services] window that opens, right-click the [InternetConnectionSharing(ICS)] service, and then select the [Properties] option. 5. In the properties window that opens, change [Open with] to [Disabled], click [Apply] and then click [OK]. 6. Click the start logo, then click the shutdown button, select [Restart], and complete the computer restart.
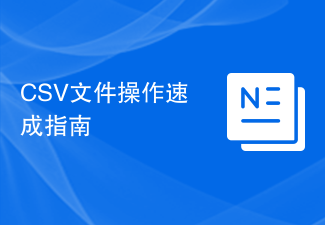
Quickly learn how to open and process CSV format files. With the continuous development of data analysis and processing, CSV format has become one of the widely used file formats. A CSV file is a simple and easy-to-read text file with different data fields separated by commas. Whether in academic research, business analysis or data processing, we often encounter situations where we need to open and process CSV files. The following guide will show you how to quickly learn to open and process CSV format files. Step 1: Understand the CSV file format First,
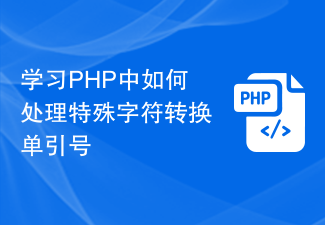
In the process of PHP development, dealing with special characters is a common problem, especially in string processing, special characters are often escaped. Among them, converting special characters into single quotes is a relatively common requirement, because in PHP, single quotes are a common way to wrap strings. In this article, we will explain how to handle special character conversion single quotes in PHP and provide specific code examples. In PHP, special characters include but are not limited to single quotes ('), double quotes ("), backslash (), etc. In strings
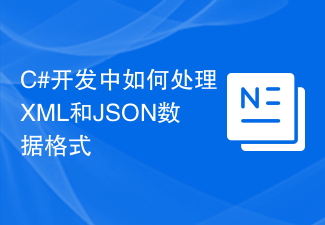
How to handle XML and JSON data formats in C# development requires specific code examples. In modern software development, XML and JSON are two widely used data formats. XML (Extensible Markup Language) is a markup language used to store and transmit data, while JSON (JavaScript Object Notation) is a lightweight data exchange format. In C# development, we often need to process and operate XML and JSON data. This article will focus on how to use C# to process these two data formats, and attach
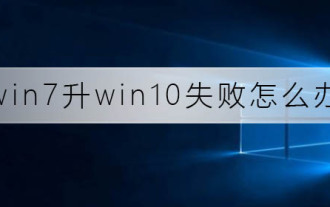
If the operating system we use is win7, some friends may fail to upgrade from win7 to win10 when upgrading. The editor thinks we can try upgrading again to see if it can solve the problem. Let’s take a look at what the editor did for details~ What to do if win7 fails to upgrade to win10. Method 1: 1. It is recommended to download a driver first to evaluate whether your computer can be upgraded to Win10. 2. Then use the driver test after upgrading. Check if there are any driver abnormalities, and then fix them with one click. Method 2: 1. Delete all files under C:\Windows\SoftwareDistribution\Download. 2.win+R run "wuauclt.e
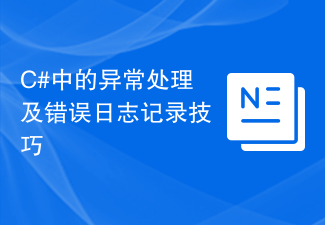
Exception handling and error logging skills in C# Introduction: In the software development process, exception handling and error logging are very important links. For C# developers, mastering exception handling skills and error logging methods can help us better track and debug code, and improve the stability and maintainability of the program. This article will introduce commonly used exception handling techniques in C# and provide specific code examples to help readers better understand and apply exception handling and error logging. 1. Basic concepts of exception handling Exceptions refer to the
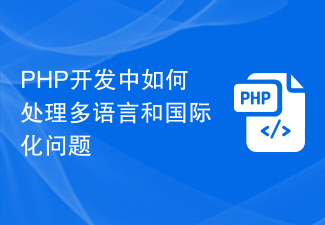
How to deal with multi-language and internationalization issues in PHP development requires specific code examples. With the development of the Internet, people's demand for multi-language and internationalization is getting higher and higher. In PHP development, how to effectively handle multi-language and internationalization issues has become an important task that developers need to solve. Handling of character encoding In PHP development, we must first ensure that character encoding is handled correctly. In multi-language environments, using UTF-8 encoding is the most common choice. You can add the following code to the head of the PHP file: header('C
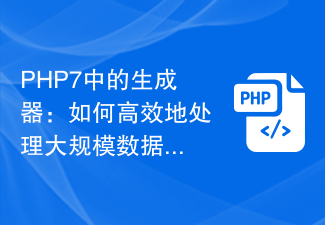
Generators in PHP7: How to handle large-scale data efficiently and save memory? Overview: PHP7 introduces generators as a powerful tool in terms of large-scale data processing and memory saving. Generators are a special type of function in the PHP language. Unlike ordinary functions, generators can pause execution and return intermediate results instead of returning all results at once. This makes the generator ideal for processing large batches of data, reducing memory usage and improving processing efficiency. This article will introduce students
