Detailed explanation of loop statements in C++
C is an efficient programming language with powerful features and a wide range of applications. Loop statements are one of the most important parts of C. C provides several loop statements to make it easier for programmers to iterate data. This article will introduce the loop statement in C in detail.
1. for loop
The for loop is an iterative statement that allows programmers to easily perform a specified operation repeatedly. The basic syntax of a for loop is as follows:
for(initialization; condition; increment) { // code to be executed }
Among them, initialization is the initialization expression, which is executed once before the start of the loop; condition is the loop condition expression, if it is true, the loop continues to be executed; increment is the increment A quantitative expression that is executed once at the end of each loop.
For example, the following code snippet will print numbers from 0 to 9:
for(int i=0; i<10; i++) { cout<<i<<endl; }
At this time, the program will output the numbers 0 to 9 on the console.
2. While loop
The while loop is a common iteration structure that is used to repeatedly execute one or more statements until the condition is no longer true. The basic syntax of the while loop is as follows:
while(condition) { // code to be executed }
The condition is calculated. If the result is true, the loop body will be executed; otherwise, the program will jump out of the loop and continue executing the program after the loop.
The following code segment prints the numbers 1~5:
int i = 1; while(i <= 5) { cout << i << endl; i++; }
At this time, the program will output the numbers 1~5 on the console.
3. do-while loop
The do-while loop is similar to the while loop, but the do-while loop will test the loop condition at least once after executing the loop body. The basic syntax is as follows:
do { // code to be executed } while(condition);
At the beginning of the loop, first execute the statements in the loop body, and then calculate the condition. If the result is true, execute the code in the loop body. When the condition is false, break out of the loop.
The output result of the following code is consistent with the previous while loop:
int i = 1; do { cout << i << endl; i++; } while (i <= 5);
4. Nested loops
Nested loops refer to nested loops within a loop. Another cycle. Use nested loops to handle complex logic operations.
The following code snippet demonstrates how to use nested loops to output the multiplication table:
for (int i = 1; i <= 9; i++) { for (int j = 1; j <= i; j++) { cout << j << "*" << i << "=" << i * j << " "; } cout << endl; }
The above code will output the following multiplication table:
1*1=1 1*2=2 2*2=4 1*3=3 2*3=6 3*3=9 1*4=4 2*4=8 3*4=12 4*4=16 1*5=5 2*5=10 3*5=15 4*5=20 5*5=25 1*6=6 2*6=12 3*6=18 4*6=24 5*6=30 6*6=36 1*7=7 2*7=14 3*7=21 4*7=28 5*7=35 6*7=42 7*7=49 1*8=8 2*8=16 3*8=24 4*8=32 5*8=40 6*8=48 7*8=56 8*8=64 1*9=9 2*9=18 3*9=27 4*9=36 5*9=45 6*9=54 7*9=63 8*9=72 9*9=81
Summary:
This article mainly introduces three common loop statements: for loop, while loop and do-while loop, as well as the use of nested loops. Programmers can choose different loop statements to complete specific tasks according to the actual situation, improving the efficiency and readability of the program.
The above is the detailed content of Detailed explanation of loop statements in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


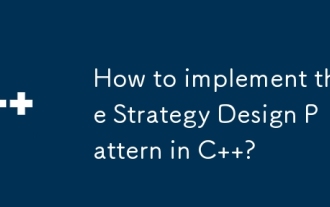
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
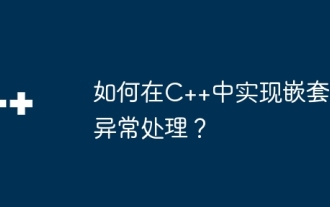
Nested exception handling is implemented in C++ through nested try-catch blocks, allowing new exceptions to be raised within the exception handler. The nested try-catch steps are as follows: 1. The outer try-catch block handles all exceptions, including those thrown by the inner exception handler. 2. The inner try-catch block handles specific types of exceptions, and if an out-of-scope exception occurs, control is given to the external exception handler.
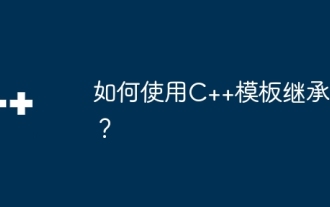
C++ template inheritance allows template-derived classes to reuse the code and functionality of the base class template, which is suitable for creating classes with the same core logic but different specific behaviors. The template inheritance syntax is: templateclassDerived:publicBase{}. Example: templateclassBase{};templateclassDerived:publicBase{};. Practical case: Created the derived class Derived, inherited the counting function of the base class Base, and added the printCount method to print the current count.
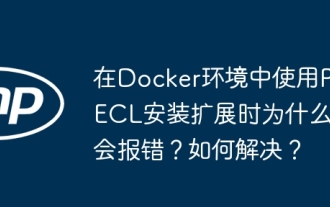
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
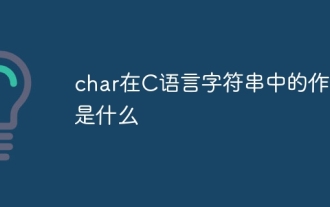
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
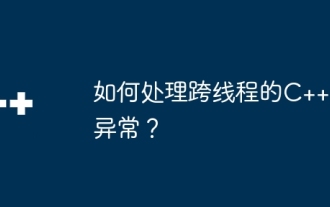
In multi-threaded C++, exception handling is implemented through the std::promise and std::future mechanisms: use the promise object to record the exception in the thread that throws the exception. Use a future object to check for exceptions in the thread that receives the exception. Practical cases show how to use promises and futures to catch and handle exceptions in different threads.
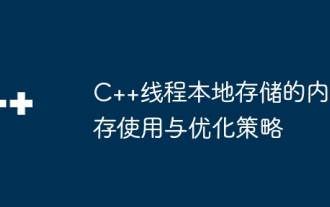
TLS provides each thread with a private copy of the data, stored in the thread stack space, and memory usage varies depending on the number of threads and the amount of data. Optimization strategies include dynamically allocating memory using thread-specific keys, using smart pointers to prevent leaks, and partitioning data to save space. For example, an application can dynamically allocate TLS storage to store error messages only for sessions with error messages.
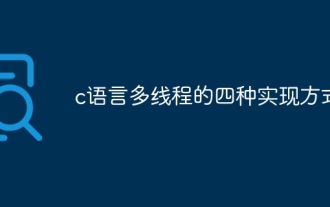
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
