Heuristic algorithm optimization techniques in C++
Heuristic algorithm optimization skills in C
Heuristic algorithm is a type of algorithm that solves problems through experience, rules and heuristic operations. In the field of computer science, heuristic algorithms are often used to solve problems with high computational complexity, such as NP-complete problems. Heuristic algorithms have applications in many fields, such as computing games, artificial intelligence, data mining, biology, etc.
C is an efficient, strongly typed programming language that is widely used in algorithm design and implementation. The implementation of heuristic algorithms in C needs to consider algorithm efficiency and problem-solving quality. This article will introduce heuristic algorithm optimization techniques in C.
- Algorithm design
When designing a heuristic algorithm, it is necessary to consider algorithm efficiency and the quality of problem solving. Generally speaking, heuristic algorithms often adopt techniques such as greedy strategy, local search, randomization or combination. When designing an algorithm, it is necessary to determine the basic framework of the algorithm based on the nature of the problem, and optimize the algorithm details based on the actual situation.
- Data structure selection
The data structure is the basis of the algorithm. Choosing the appropriate data structure can improve the efficiency of the algorithm. C provides many common data structures, such as arrays, vectors, linked lists, stacks, queues, heaps, hash tables, etc. Choose a data structure based on specific algorithm needs. It should be noted that the efficiency of different data structures may vary under different circumstances.
- Code implementation
You need to pay attention to the following points when implementing the heuristic algorithm in C:
(1) Variable definition and initialization: Variables should Define before use. In addition, initialization of variables is also very important.
(2) Loop structure: Loop structure is required when writing any computer program, so you need to learn to use for loops, while loops and other structures, as well as the skills of using break and continue statements in loops.
(3) Program performance optimization: There are many technologies in C that can improve program performance, such as inline functions, template technology, compiler optimization, etc.
(4) Error handling: Error handling needs to be considered when writing a program, so as to ensure the stability and robustness of the program.
- Commonly used heuristic algorithm optimization techniques
(1) Greedy algorithm: Greedy algorithm is an algorithm based on greedy selection and driven by local optimality. Greedy algorithms can solve many practical problems, such as minimum spanning tree, shortest path, knapsack problem, etc.
(2) Simulated annealing algorithm: The simulated annealing algorithm is a randomized algorithm that can be used to solve optimization problems. It uses a certain probability to accept suboptimal solutions to avoid falling into local optimal solutions.
(3) Genetic algorithm: The genetic algorithm is an algorithm based on the theory of biological evolution. It searches for the optimal solution through group evolution. Genetic algorithms can be used to solve complex optimization problems, such as the traveling salesman problem, knapsack problem, etc.
(4) Tabu search algorithm: The tabu search algorithm is a local search algorithm that finds better solutions by jumping out of the local optimal solution. The tabu search algorithm prevents the algorithm from falling into a local optimal solution by setting a tabu list.
- Conclusion
This article introduces heuristic algorithm optimization techniques in C. Heuristic algorithms are used in many fields, and it is very challenging to design and implement an efficient heuristic algorithm. In practice, it is necessary to select algorithms and data structures according to specific situations, and combine program performance optimization technology to improve algorithm efficiency.
The above is the detailed content of Heuristic algorithm optimization techniques in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


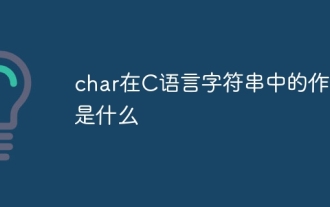
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
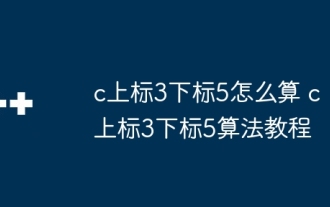
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
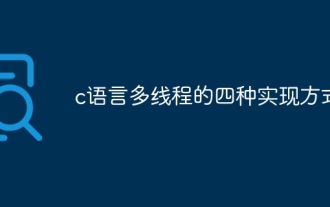
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
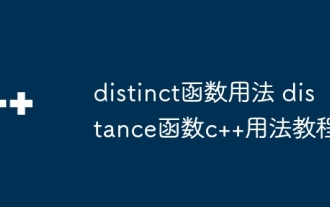
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
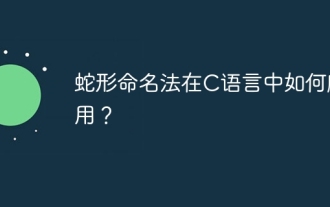
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
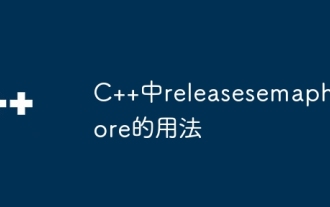
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
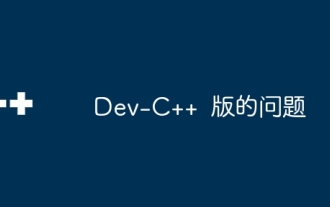
Dev-C 4.9.9.2 Compilation Errors and Solutions When compiling programs in Windows 11 system using Dev-C 4.9.9.2, the compiler record pane may display the following error message: gcc.exe:internalerror:aborted(programcollect2)pleasesubmitafullbugreport.seeforinstructions. Although the final "compilation is successful", the actual program cannot run and an error message "original code archive cannot be compiled" pops up. This is usually because the linker collects
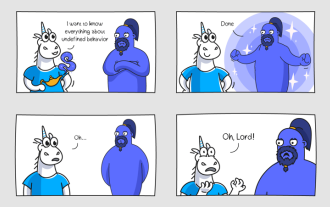
Exploring Undefined Behaviors in C Programming: A Detailed Guide This article introduces an e-book on Undefined Behaviors in C Programming, a total of 12 chapters covering some of the most difficult and lesser-known aspects of C Programming. This book is not an introductory textbook for C language, but is aimed at readers familiar with C language programming, and explores in-depth various situations and potential consequences of undefined behaviors. Author DmitrySviridkin, editor Andrey Karpov. After six months of careful preparation, this e-book finally met with readers. Printed versions will also be launched in the future. This book was originally planned to include 11 chapters, but during the creation process, the content was continuously enriched and finally expanded to 12 chapters - this itself is a classic array out-of-bounds case, and it can be said to be every C programmer
