How to optimize algorithm scalability in C++ development
How to optimize algorithm scalability in C development
In software development, algorithm scalability is a very important issue. For C developers, being able to design and implement algorithms that scale well is a key skill. This article will discuss some optimization techniques to help C developers improve the scalability of their algorithms.
- Abstract the core part of the algorithm
To optimize the scalability of the algorithm, we must first understand the core functions of the algorithm. By abstracting away the core modules in an algorithm, it can be decoupled from specific implementation details. You can use classes or templates to encapsulate core modules and provide interfaces for other modules to call.
- Use object-oriented design principles
Object-oriented design principles can help us build algorithms with good scalability. For example, the single responsibility principle can help us split different functions into different classes so that each class is responsible for only one responsibility. The open-closed principle can help us implement new functions by extending existing classes instead of modifying existing classes.
- Using Generic Programming
Generic programming in C is a powerful technique that can improve the scalability of algorithms. By using generic programming, we can decouple algorithms from specific data types, making the algorithm applicable to different types of data. By using template classes and functions, we can implement common algorithms while ensuring high efficiency.
- Using Design Patterns
Design patterns are proven solutions that can help us solve common software design problems. In algorithm development, some design patterns can be used to improve scalability. For example, the factory pattern can help us decouple specific implementation classes and calling classes, allowing the algorithm to dynamically select specific implementations. The strategy pattern can help us encapsulate the implementation of various algorithms and dynamically switch algorithms at runtime.
- Use appropriate data structures
Choosing appropriate data structures is very important for the scalability of the algorithm. Different data structures have different characteristics and can be applied to different problems. For example, if you need to frequently insert and delete elements, you can choose a linked list as the data structure to store the data. If you need fast search operations, you can choose a hash table or binary search tree.
- Performance optimization
Performance optimization is also part of the algorithm scalability. If an algorithm has poor performance, it may not be used even if it scales well. Therefore, you should always pay attention to the performance of the algorithm during development and optimize it accordingly. You can use some performance analysis tools to locate performance bottlenecks and perform targeted optimization.
- Write clear documentation and comments
Good documentation and comments can help other developers quickly understand and use your algorithm. When writing code, you should pay attention to writing clear comments and documentation, including how to use the algorithm, precautions, and parameter descriptions. This makes it easier for other developers to understand and extend your algorithm when they use it.
Summary:
Optimizing algorithm scalability in C development is a process that requires skill and experience. Through abstraction, object-oriented design, generic programming, design patterns, appropriate data structures, performance optimization, and clear documentation and comments, we can improve the scalability of our algorithms and make our code easier to maintain and extend. I hope the content of this article can help C developers optimize the scalability of algorithms.
The above is the detailed content of How to optimize algorithm scalability in C++ development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


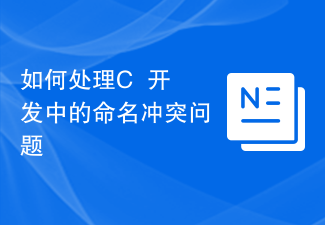
How to deal with naming conflicts in C++ development. Naming conflicts are a common problem during C++ development. When multiple variables, functions, or classes have the same name, the compiler cannot determine which one is being referenced, leading to compilation errors. To solve this problem, C++ provides several methods to handle naming conflicts. Using Namespaces Namespaces are an effective way to handle naming conflicts in C++. Name conflicts can be avoided by placing related variables, functions, or classes in the same namespace. For example, you can create
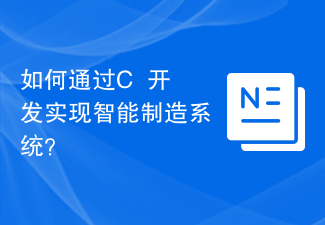
How to implement intelligent manufacturing system through C++ development? With the development of information technology and the needs of the manufacturing industry, intelligent manufacturing systems have become an important development direction of the manufacturing industry. As an efficient and powerful programming language, C++ can provide strong support for the development of intelligent manufacturing systems. This article will introduce how to implement intelligent manufacturing systems through C++ development and give corresponding code examples. 1. Basic components of an intelligent manufacturing system An intelligent manufacturing system is a highly automated and intelligent production system. It mainly consists of the following components:
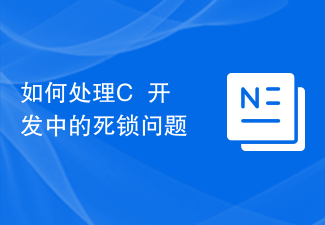
How to deal with deadlock problems in C++ development Deadlock is one of the common problems in multi-threaded programming, especially when developing in C++. Deadlock problems may occur when multiple threads wait for each other's resources. If not handled in time, deadlock will not only cause the program to freeze, but also affect the performance and stability of the system. Therefore, it is very important to learn how to deal with deadlock problems in C++ development. 1. Understand the causes of deadlocks. To solve the deadlock problem, you first need to understand the causes of deadlocks. Deadlock usually occurs when
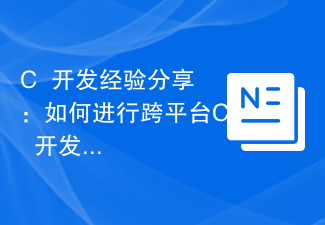
C++ is a powerful programming language that is widely used in software development in various fields. However, due to the differences between different operating systems, C++ developers often face a problem: how to perform cross-platform C++ development? This article will share some C++ development experience to help you achieve success in cross-platform development. Understand the target platform features First, you need to understand the features and limitations of the target platform. Different operating systems have different APIs, file systems, and network communications. Therefore, before carrying out cross-platform development, you must first
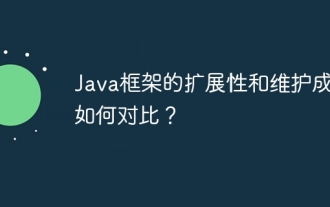
When choosing a Java framework, Spring Framework is known for its high scalability, but as complexity increases, maintenance costs also increase. In contrast, Dropwizard is generally less expensive to maintain but less scalable. Developers should evaluate frameworks based on specific needs.
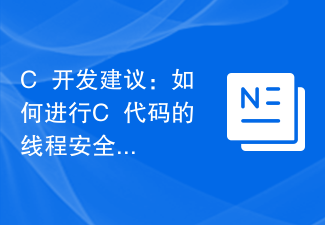
C++ is a very powerful programming language that is widely used in development in various fields. However, when using C++ to develop multi-threaded applications, developers need to pay special attention to thread safety issues. If an application has thread safety issues, it may lead to application crashes, data loss, and other issues. Therefore, when designing C++ code, you should pay attention to thread safety issues. Here are a few suggestions for thread-safe design of C++ code. Avoid using global variables Using global variables may lead to thread safety issues. If multiple lines
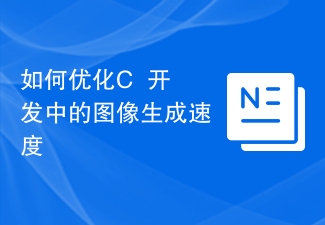
Overview of how to optimize image generation speed in C++ development: In today's computer applications, image generation has become an indispensable part. As an efficient, statically typed programming language, C++ is widely used in the development of image generation. However, as the complexity of image generation tasks continues to increase, performance requirements are becoming higher and higher. Therefore, how to optimize the image generation speed in C++ development has become an important topic. This article will introduce some commonly used optimization methods and techniques to help developers achieve efficient graphs in C++.
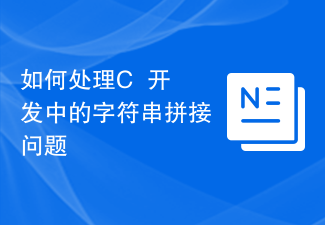
How to deal with string concatenation issues in C++ development In C++ development, string concatenation is a common task. Whether you are concatenating multiple strings together or concatenating strings with other data types, handling string concatenation correctly is critical to your program's performance and readability. This article will introduce some common methods and techniques to help readers effectively deal with string splicing problems in C++ development. 1. Use operator overloading for string splicing. String splicing in C++ can be achieved by overloading the addition operator.
