Multithreading optimization techniques in C++
With the development of computer technology and the improvement of hardware performance, multi-threading technology has become an essential skill for modern programming. C is a classic programming language that also provides many powerful multi-threading technologies. This article will introduce some multi-threading optimization techniques in C to help readers better apply multi-threading technology.
1. Use std::thread
C 11 introduced std::thread, integrating multi-threading technology directly into the standard library. Creating a new thread using std::thread is very simple, just pass a function pointer. For example:
#include <thread> #include <iostream> void hello() { std::cout << "Hello World!"; } int main() { std::thread t(hello); t.join(); return 0; }
The above code creates a new thread t, executes the hello function, and waits for thread t to complete. Note that thread creation and destruction requires a certain amount of overhead, so std::thread needs to be used rationally.
2. Use std::async
std::async is another convenient multi-threading technology, which can execute a function asynchronously and return a std::future object. Use std::async to more conveniently manage the execution of asynchronous tasks and obtain results. For example:
#include <future> #include <iostream> int add(int a, int b) { return a + b; } int main() { auto async_result = std::async(add, 1, 2); std::cout << async_result.get(); return 0; }
The above code calls the add function to calculate 1 2 asynchronously, and uses the std::future object to manage the acquisition of the calculation results. It should be noted that std::async uses the std::launch::async strategy by default and will execute functions in a new thread. If you wish to use the std::launch::deferred strategy, you need to specify it manually. However, using the std::launch::deferred strategy will cause the function to be executed only when std::future::get() is called, so the choice needs to be made on a case-by-case basis.
3. Use std::condition_variable
In multi-threaded programming, communication and synchronization need to be carried out between threads, and std::condition_variable can achieve this purpose very well. Using std::condition_variable allows one thread to wait for a certain condition of another thread to be true, thereby achieving synchronization between threads. For example:
#include <condition_variable> #include <mutex> #include <thread> #include <iostream> std::mutex mutex; std::condition_variable cv; bool ready = false; void producer() { std::unique_lock<std::mutex> lock(mutex); // wait for the condition to become true cv.wait(lock, [] { return ready; }); std::cout << "Producer done." << std::endl; } void consumer() { std::this_thread::sleep_for(std::chrono::seconds(1)); ready = true; std::cout << "Consumer done." << std::endl; cv.notify_one(); } int main() { std::thread t1(producer); std::thread t2(consumer); t1.join(); t2.join(); return 0; }
The above code creates two threads t1 and t2, where t1 is waiting until a condition variable ready becomes true, and t2 sets the condition variable to after waiting for 1 second. true, and notify t1. It should be noted that std::condition_variable must be used in conjunction with std::mutex to prevent multiple threads from accessing condition variables at the same time.
4. Use the thread pool
In the case of a large number of short-term tasks that need to be created and run, the thread pool is often used to improve the performance of the program. The thread pool maintains a certain number of threads and manages the allocation and execution of tasks. Using a thread pool can avoid the additional overhead of frequently creating and destroying threads, while taking full advantage of multi-core CPUs. For example:
#include <iostream> #include <thread> #include <mutex> #include <condition_variable> #include <vector> #include <queue> #include <functional> class ThreadPool { public: ThreadPool(std::size_t numThreads = std::thread::hardware_concurrency()) { for (std::size_t i = 0; i < numThreads; ++i) { pool.emplace_back([this] { while (!stop) { std::function<void()> task; { std::unique_lock<std::mutex> lock{ mutex }; condition.wait(lock, [this] { return stop || !tasks.empty(); }); if (stop && tasks.empty()) return; task = std::move(tasks.front()); tasks.pop(); } task(); } }); } } ~ThreadPool() { { std::unique_lock<std::mutex> lock{ mutex }; stop = true; } condition.notify_all(); for (auto& worker : pool) { worker.join(); } } template <typename F, typename... Args> auto enqueue(F&& f, Args&&... args) -> std::future<typename std::result_of<F(Args...)>::type> { using return_type = typename std::result_of<F(Args...)>::type; auto task = std::make_shared<std::packaged_task<return_type()>>( std::bind(std::forward<F>(f), std::forward<Args>(args)...)); std::future<return_type> future = task->get_future(); { std::unique_lock<std::mutex> lock{ mutex }; if (stop) throw std::runtime_error("enqueue on stopped ThreadPool"); tasks.emplace([task](){ (*task)(); }); } condition.notify_one(); return future; } private: std::vector<std::thread> pool; std::queue<std::function<void()>> tasks; std::mutex mutex; std::condition_variable condition; bool stop = false; }; void hello() { std::cout << "Hello World!" << std::endl; } int add(int a, int b) { return a + b; } int main() { { ThreadPool pool; auto f1 = pool.enqueue(hello); auto f2 = pool.enqueue(add, 1, 2); std::cout << f2.get() << std::endl; } return 0; }
The above code defines a ThreadPool class, which contains multiple threads and a task queue. The thread pool continues to take tasks from the task queue and execute them until the queue is empty or the thread pool stops. Use the ThreadPool::enqueue method to add the task to the task queue and return a std::future object to manage the results of task execution.
In general, C provides a variety of multi-threading technologies to help developers take advantage of the performance of multi-core CPUs and manage threads and tasks more flexibly. Developers should use these techniques appropriately to optimize program performance.
The above is the detailed content of Multithreading optimization techniques in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


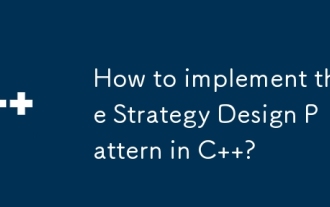
The steps to implement the strategy pattern in C++ are as follows: define the strategy interface and declare the methods that need to be executed. Create specific strategy classes, implement the interface respectively and provide different algorithms. Use a context class to hold a reference to a concrete strategy class and perform operations through it.
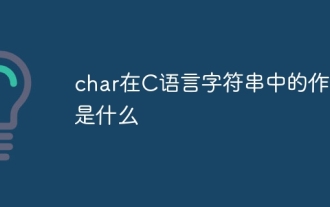
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
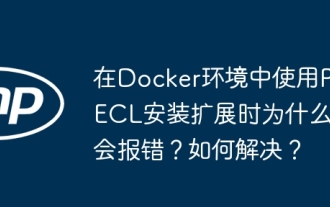
Causes and solutions for errors when using PECL to install extensions in Docker environment When using Docker environment, we often encounter some headaches...
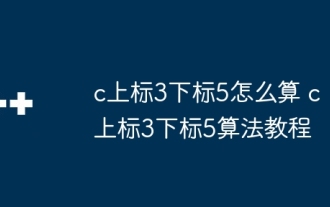
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
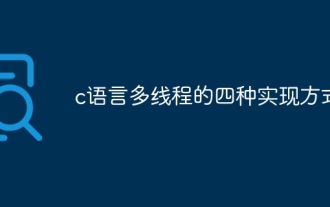
Multithreading in the language can greatly improve program efficiency. There are four main ways to implement multithreading in C language: Create independent processes: Create multiple independently running processes, each process has its own memory space. Pseudo-multithreading: Create multiple execution streams in a process that share the same memory space and execute alternately. Multi-threaded library: Use multi-threaded libraries such as pthreads to create and manage threads, providing rich thread operation functions. Coroutine: A lightweight multi-threaded implementation that divides tasks into small subtasks and executes them in turn.
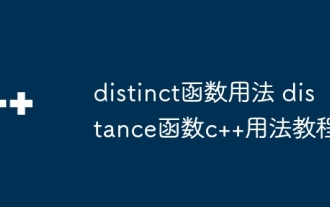
std::unique removes adjacent duplicate elements in the container and moves them to the end, returning an iterator pointing to the first duplicate element. std::distance calculates the distance between two iterators, that is, the number of elements they point to. These two functions are useful for optimizing code and improving efficiency, but there are also some pitfalls to be paid attention to, such as: std::unique only deals with adjacent duplicate elements. std::distance is less efficient when dealing with non-random access iterators. By mastering these features and best practices, you can fully utilize the power of these two functions.
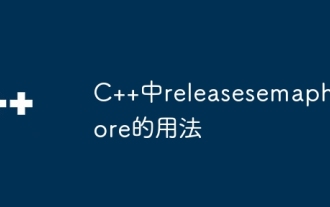
The release_semaphore function in C is used to release the obtained semaphore so that other threads or processes can access shared resources. It increases the semaphore count by 1, allowing the blocking thread to continue execution.
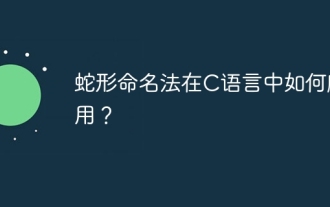
In C language, snake nomenclature is a coding style convention, which uses underscores to connect multiple words to form variable names or function names to enhance readability. Although it won't affect compilation and operation, lengthy naming, IDE support issues, and historical baggage need to be considered.
