Using text files for input and output in C++
In C, the processing of text files is very important, because most programs need to perform input and output with external files, such as reading data files stored on disk or converting Data within the program is stored in files. Therefore, this article will introduce how to use text files for input and output in C.
1. Opening and closing text files
In C, you need to use the file stream class object (fstream) to read and write files. Before using a file, you need to create a file stream object and associate it with the file. The constructor of a file stream object can accept the file name and opening method as parameters. There are three common file opening methods in C:
- ios::in: Open the file in read-only mode.
- ios::out: Open the file in write-only mode.
- ios::app: Open the file in append mode.
The following is a simple example to demonstrate how to open a file:
#include <fstream> using namespace std; int main() { // 创建文件流对象并关联文件 fstream file("example.txt", ios::in | ios::out); // 判断文件是否打开成功 if (!file) { cout << "无法打开文件" << endl; return -1; } // 文件操作 // 关闭文件 file.close(); return 0; }
In the above code, a file stream object named file is created through the constructor of the fstream class. And associate it with the file example.txt. When a file is opened, a method is used that is both readable and writable. In other words, when you need to read a file, you need to use the ios::in option; when you need to write a file, you need to use the ios::out option; when you need to add content at the end of the file, you need to use ios ::appoptions. For convenience, both readable and writable methods are used here.
After the file operation is completed, the file needs to be closed. This can be achieved through the close() function of the file stream object.
2. Reading data from the file
After opening the text file, we can use the file input stream object (ifstream) to read data from the text file. C provides a variety of methods to read data from files, including the following:
- getline() function: Read a line of text data from the file.
Operator: Read a word or a value from the file.
- read() function: Read data of specified length from the file.
- get() function: Read a character from the file.
- peek() function: Pre-read the next character but do not remove it from the file.
- ignore() function: Ignore the specified number of characters in the file.
The following is a simple example that demonstrates how to read data from a file:
#include <fstream> #include <iostream> using namespace std; int main() { // 创建文件流对象并关联文件 ifstream file("example.txt"); // 判断文件是否打开成功 if (!file) { cout << "无法打开文件" << endl; return -1; } // 读取文件内容 string str; while (getline(file, str)) { cout << str << endl; } // 关闭文件 file.close(); return 0; }
In the above code, we create an input file stream object named file, And associate it to the file example.txt. In the while loop, the file content is read line by line by calling the getline() function, and each line of data is stored in the string variable str. Finally, the read content is output through cout.
3. Write data to a file
In C, you can use the file output stream object (ofstream) to write data to a text file. Commonly used writing functions include the following:
- <
- put() function: Write a character.
- write() function: Write data of specified length.
The following is a simple example that demonstrates how to write data to a file:
#include <fstream> #include <iostream> using namespace std; int main() { // 创建文件流对象并关联文件 ofstream file("example.txt"); // 判断文件是否打开成功 if (!file) { cout << "无法打开文件" << endl; return -1; } // 向文件中写入数据 for (int i = 1; i <= 10; i++) { file << "This is line " << i << endl; } // 关闭文件 file.close(); return 0; }
In the above code, we create an output file stream object named file, And associate it to the file example.txt. In the for loop, data is written to the file through the << operator. Each loop writes one line of text data, and finally the file is closed through the close() function.
4. File pointer
When performing file operations, you need to use a pointer to indicate the current file read and write location. The file pointer, often called the file position indicator, is an internal variable maintained by each file that records the number of bytes from the start of the file to the current position.
C provides the following functions to operate the file pointer:
- tellg() function: Get the file reading position.
- tellp() function: Get the file writing location.
- seekg() function: Set the file reading position.
- seekp() function: Set the file writing location.
The following is a simple example that demonstrates how to operate the file pointer:
#include <fstream> #include <iostream> using namespace std; int main() { // 创建文件流对象并关联文件 fstream file("example.txt", ios::in | ios::out); // 判断文件是否打开成功 if (!file) { cout << "无法打开文件" << endl; return -1; } // 获取、设置文件指针位置 file.seekp(6); // 将文件写位置设置为6 file.put('X'); // 在文件位置6处写入字符'X' file.seekg(0); // 将文件读位置设置为0 char ch = file.get(); // 从文件位置0读取一个字符 cout << "第一个字符是:" << ch << endl; // 关闭文件 file.close(); return 0; }
In the above code, we create a file stream object named file and associate it with to the file example.txt. Before manipulating the file pointer, the file needs to be opened.
In the example, we use the seekp() function to set the file pointer position to 6, and then use the put() function to write the character 'X' at file position 6. Next, use the seekg() function to set the file pointer position to 0, use the get() function to read a character from file position 0, and output it.
5. Summary
The above are the basic operations for input and output of text files using C. When performing file operations, you need to pay attention to the order in which files are opened and closed, as well as the operation of the file pointer position. Text file reading and writing operations, pointer positioning and other operations are very simple, but you need to keep in mind the commonly used functions and operation methods.
The above is the detailed content of Using text files for input and output in C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


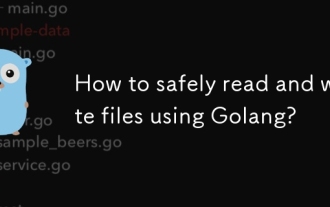
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
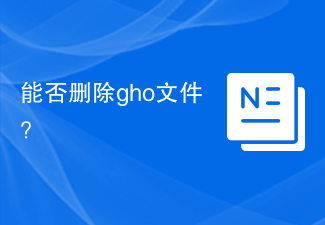
A gho file is an image file created by NortonGhost software and used to back up and restore the operating system and data. In some cases, you can delete gho files, but do so with caution. This article will introduce the role of gho files, precautions for deleting gho files, and how to delete gho files. First, let's understand the role of gho files. A gho file is a compressed system and data backup file that can save an image of an entire hard disk or a specific partition. This kind of backup file is usually used for emergency recovery
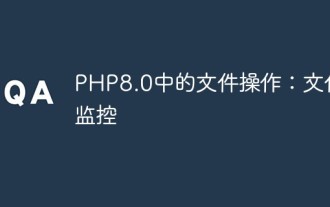
With the continuous development of web applications, PHP has become one of the most important programming languages in web development. As an extremely flexible programming language, each version of PHP brings new features and optimizations to meet different needs and application scenarios. In the PHP8.0 version, a very practical file operation function has been added, namely file monitoring. This function is very suitable for application scenarios that require monitoring and processing of file changes, such as file backup, file synchronization, log monitoring, etc. This article will take you
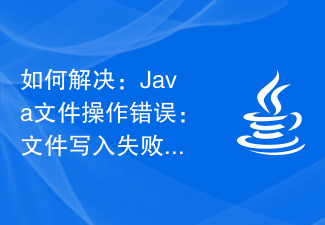
How to solve: Java file operation error: File writing failed. In Java programming, you often encounter the need for file operations, and file writing is one of the important functions. However, sometimes we encounter file writing failure errors, which may prevent the program from running properly. This article will describe some common causes and solutions to help you solve this type of problem. Wrong path: A common problem is wrong file path. When we try to write a file to the specified path, if the path does not exist or the permissions are insufficient, the file will be written.
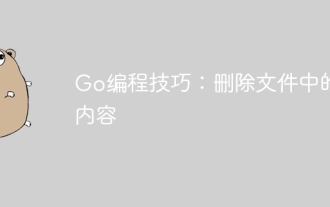
The Go language provides two methods to clear file contents: using io.Seek and io.Truncate, or using ioutil.WriteFile. Method 1 involves moving the cursor to the end of the file and then truncating the file, method 2 involves writing an empty byte array to the file. The practical case demonstrates how to use these two methods to clear content in Markdown files.
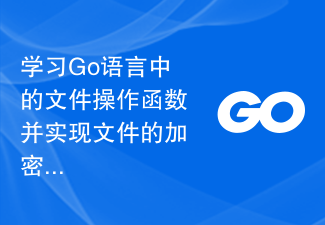
Learn the file operation functions in Go language and implement the encryption, compression, upload and download functions of files. Go language is an open source statically typed programming language. It is widely popular in the development field for its efficient performance and concise syntax. The standard library of the Go language provides a wealth of file operation functions, making it very simple to read and write files, encrypt and compress them, upload and download them. This article will introduce how to use the file operation functions in the Go language to implement the functions of encrypting, compressing, uploading and downloading files. First, we need to import the relevant three
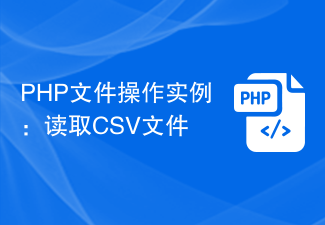
PHP is a popular programming language widely used in web development. In web applications, file operations are a basic and common function. This article will explain how to use PHP to read a CSV file and display it in an HTML table. CSV is a common file format used to import tabular data into spreadsheet software such as Excel. CSV files usually consist of many lines, each line consisting of comma separated values. The first line usually contains column headers, which describe the meaning of each column value. Here we will use PHP
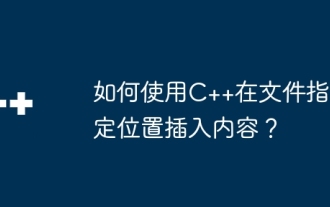
In C++, use the ofstream class to insert content at a specified location in a file: open the file and locate the insertion point. use
