


C++ compilation error: Multiple types cannot be cast, how to deal with it?
C Compilation error: Multiple types cannot be cast, how to deal with it?
In C program development, forced type conversion is a common operation. However, when using forced type conversion, we often encounter errors in which forced type conversion cannot be performed between multiple types. So, how to deal with this type conversion error?
1. Understand forced type conversion
When performing forced type conversion, we need to use the four forced type conversion methods provided by the C language: static_cast, reinterpret_cast, const_cast and dynamic_cast.
Among them, static_cast is the most commonly used type conversion method. It can convert basic types into other types without changing the type. It can also convert pointers or reference types with inheritance relationships into their parent classes or Subclass type. reinterpret_cast can convert a pointer or reference of any type to a pointer or reference of another type, but may result in undefined behavior. const_cast is used to add or remove const qualifier from a pointer or reference type. dynamic_cast can perform safe conversions in pointer or reference types with inherited relationships, but can only convert pointer or reference types to their parent or subclass types. By correctly choosing the coercion method, you can avoid the problem of inability to coerce type conversion between multiple types.
2. Reasons why multiple types cannot be forced to convert
1. Type incompatibility
In C, different combinations of the same data type may produce different Data types such as char, int, long and float can be combined, but the data types produced by different combination methods are different. If you convert an incompatible data type to another data type, a compilation error will occur.
2. Type implicit conversion
In some cases, C will automatically perform type conversion. For example, when performing an arithmetic or relational operation, if the operands are of different types, C converts one of the operands to the other type. If you force a type that has been implicitly converted to another data type, multiple types cannot be cast errors will occur.
3. Data precision loss
When performing data type conversion, problems such as loss of decimal place precision may occur. For example, when casting a floating-point number to an integer, the decimal part is truncated, resulting in a loss of precision. If you convert one data type with loss of precision to another data type, various type cannot be cast errors will occur.
3. Methods for dealing with multiple types that cannot be forced to convert
1. Avoid confusion between different data types
In program development, different data types should be avoided of mixed use. For example, when performing arithmetic operations or relational operations, ensure that the operands are of the same data type. By avoiding confusion between different data types, you can avoid errors where multiple types cannot be cast.
2. Choose the correct type conversion method
When performing forced type conversion, you should choose the correct type conversion method according to the specific situation. For example, if you need to convert a floating point number to an integer, you should use static_cast or round function plus cast to avoid loss of precision. If you need to convert a pointer or reference type with an inheritance relationship, you should use dynamic_cast to perform type conversion to avoid errors in which multiple types cannot be cast.
3. Consider type compatibility
When performing forced type conversion, the compatibility between the two data types should be considered. For example, when converting between char type and int type, you can use static_cast to perform the conversion. However, due to the differences in storage methods and value ranges between the char type and the int type, the conversion results will be inaccurate. Therefore, type compatibility should be considered based on actual needs to avoid errors in which multiple types cannot be forced to convert.
In short, in program development, errors in which multiple types cannot be cast are a common problem. We can avoid compilation errors and ensure the correct operation of the program by correctly choosing the forced type conversion method. At the same time, it is necessary to avoid confusion between different data types, consider type compatibility, and prevent errors in which multiple types cannot be coerced from the source.
The above is the detailed content of C++ compilation error: Multiple types cannot be cast, how to deal with it?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


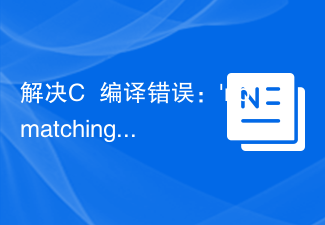
Solve C++ compilation error: 'nomatchingfunctionforcallto'function'', how to solve it? When writing programs in C++, we often encounter various compilation errors. One of the common errors is "nomatchingfunctionforcallto'function'". This error usually occurs when a function is called and the compiler cannot find a matching function declaration or definition. Book
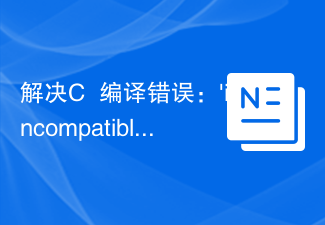
Solve C++ compilation error: 'incompatibletypes', how to solve it? During the development process of C++, we often encounter error messages given by the compiler. One common type of error is "incompatibletypes". This error message indicates that there is a type mismatch in the program, which may be inconsistent variable types, mismatched function parameter types, etc. This article will introduce several common type incompatibility errors and give corresponding solutions.
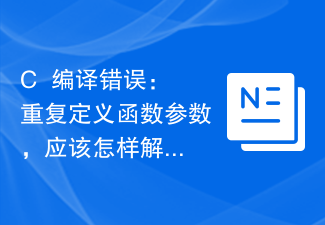
As an efficient programming language, C++ is widely used in various fields because of its reliability. However, in the process of writing code, we often encounter some compilation errors, and repeated definition of function parameters is one of them. This article will detail the reasons and solutions for repeatedly defining function parameters. What is repeatedly defining function parameters? In C++ programming, function parameters refer to variables or expressions that appear in function definitions and declarations and are used to accept actual parameters passed when a function is called. When defining a function's argument list, each argument must be
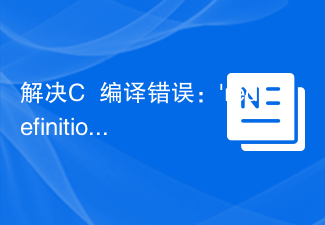
Solve C++ compilation error: 'redefinitionof'function'', how to solve it? As a powerful programming language, C++ is often widely used in software development. However, writing error-free C++ programs is not easy for beginners. One of the common errors is "redefinitionof'function'", which is a function redefinition error. In this article I will explain the causes of this error and how to fix it. wrong reason
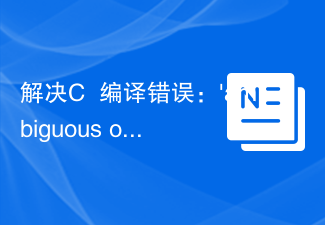
How to solve C++ compilation error: 'ambiguousoverloadfor'function''? When programming in C++, we often encounter compilation errors. Among them, a common error is 'ambiguousoverloadfor'function'. This error reminds us that there is ambiguity in overloading functions when calling functions. This article will explain the causes of this error and provide several solutions to resolve it. First, let
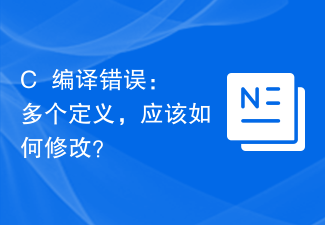
In C++ programming, "multiple definition" (multiple definitions) compilation errors often occur. This is because multiple variables, functions, or objects with the same name are defined in the program. These variables, functions, or objects are all considered to be the same by the compiler, so the compiler will generate a "multipledefinition" error. In actual programming, how should we avoid and solve such problems? Using header files in C++, we can convert some reused functions or variables into
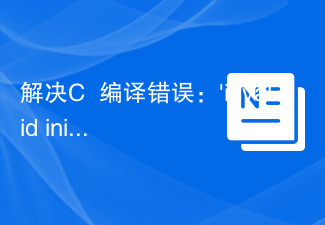
How to solve the C++ compilation error: 'invalidinitializationofreferenceoftype'type&'fromexpressionoftype'type''? Problem background: In C++ programming, we sometimes encounter compilation errors. One of them is the error message "invalidinitializationofreferenceof

C++ compilation error: Undefined variable used, how to solve it? When writing C++ programs, we often encounter compilation errors, one of the more common errors is the use of undefined variables. If you encounter this error, don't worry, next, this article will introduce you how to solve this error. The reason for this error is that an undefined or undeclared variable is used in the program. The C++ compiler does not find the definition of this variable, so it cannot allocate memory space, causing the compiler to generate an error. The solution to this problem is as follows
