How to flatten a JavaScript object into a single-depth object?
In JavaScript, objects can be created using curly braces {}. This is called an object literal. Object literals can contain properties and methods.
What is flattening?
Flattening is the process of reducing an object to a single depth object. In other words, all properties and methods of the object are contained in a single depth object.
How to flatten an object?
There are multiple ways to flatten an object.
Use for...in loop
The for...in loop can be used to iterate over the properties of an object. For each property we can add it to a new object.
The following is a code snippet that uses a for...in loop to flatten an object.
<!doctype html> <html> <head> <title>Example - flatten an object</title> </head> <body> <div id="result1">Original Object: </div> <div id="result2">Flatten Object: </div> <script> var obj = { "a": 1, "b": 2, "c": { "d": 3, "e": 4 } }; var newObj = {}; for (var key in obj) { if (typeof obj[key] === "object") { for (var subKey in obj[key]) { newObj[subKey] = obj[key][subKey]; } } else { newObj[key] = obj[key]; } } document.getElementById("result1").innerHTML += JSON.stringify(obj) document.getElementById("result2").innerHTML += JSON.stringify(newObj) console.log(newObj); </script> </body> </html>
In the above code, we declare an object literal named obj. Then we declare an empty object literal named newObj. We use a for...in loop to iterate over the properties of obj. For each attribute, we check whether the attribute value is an object. If the property value is an object, then we again use a for...in loop to iterate over the child properties of the property value.
For each subproperty, we add it to the newObj object with the subproperty name as the key. If the property value is not an object, then we add the property to the newObj object using the property name as the key. Finally, we log newObj to the console.
Benefits of flattening
Flattening objects has multiple benefits. Some of them are-
Ease of Access Properties
When an object is flattened, all properties and methods are included in an object. This makes accessing properties and methods easy.
Easy to manipulate data
When an object is flattened, the data is contained within a single object. This makes it easy to manipulate the data.
Easy to serialize
When an object is flattened, it can be easily serialized. Serialization is the process of converting objects into a format that can be stored or transmitted.
Disadvantages of flattening
Flattening objects has some disadvantages. Some of them are-
Data redundancy
When an object is flattened, data redundancy may occur. Data redundancy means the same data is stored in multiple places.
Maintenance difficulties
When an object is flattened, it can be difficult to maintain. This is because all properties and methods are contained within an object.
in conclusion
In short, flattening is the process of reducing an object to a single-depth object. There are several ways to flatten an object. Some of the benefits of flattening objects are ease of accessing properties, ease of manipulating data, and ease of serialization. Flat objects have shortcomings such as data redundancy and difficulty in maintenance.
The above is the detailed content of How to flatten a JavaScript object into a single-depth object?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


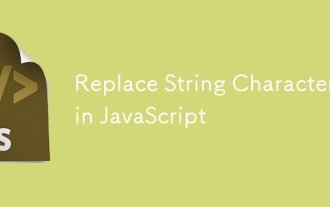
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
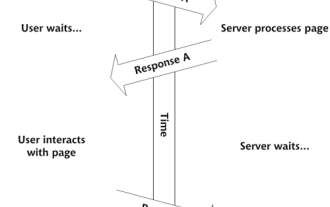
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
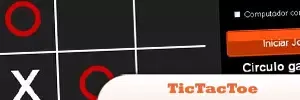
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
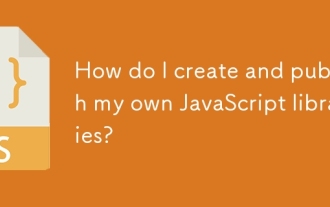
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
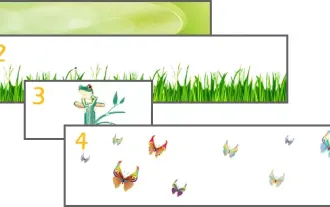
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
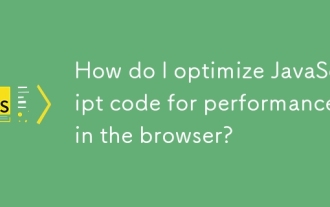
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
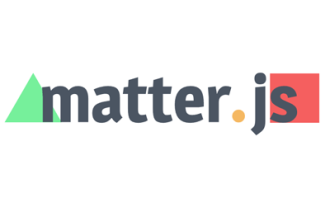
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
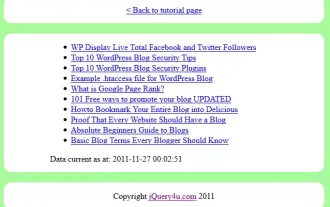
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
