JavaScript program forms coils in matrix form
We will use JavaScript to form the coils in the matrix. The process involves manipulating elements of a matrix to create spiral patterns. This can be accomplished by changing the traversal direction, keeping track of the elements visited, and adjusting the index accordingly. We will continually work on the logic to ensure that the program runs smoothly and efficiently to produce the required output.
method
One way to form coils in a matrix using JavaScript is as follows -
Define the size of the matrix.
Initialize the matrix with zeros.
Use nested loops to iterate through the matrix and change the value of a specific cell based on the pattern of the coil.
Track the traversal direction (right, down, left, up) and change the direction as needed.
Use another loop to print the matrix.
Repeat the process to form multiple coils if needed.
Example
Here is an example of how to implement a function in JavaScript to form a coil in a matrix -
function formCoils(matrix) { let row = 0, col = 0, direction = 'down'; for (let i = 0; i < matrix.length * matrix[0].length; i++) { matrix[row][col] = i + 1; if (direction === 'down') { if (row === matrix.length - 1 || matrix[row + 1][col] !== 0) { direction = 'right'; col++; } else { row++; } } else if (direction === 'right') { if (col === matrix[0].length - 1 || matrix[row][col + 1] !== 0) { direction = 'up'; row--; } else { col++; } } else if (direction === 'up') { if (row === 0 || matrix[row - 1][col] !== 0) { direction = 'left'; col--; } else { row--; } } else if (direction === 'left') { if (col === 0 || matrix[row][col - 1] !== 0) { direction = 'down'; row++; } else { col--; } } } return matrix; } const matrix = [[0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0], [0, 0, 0, 0]]; console.log(formCoils(matrix));
formCoilsThe function accepts a matrix and returns the same matrix with the numbers forming the coil shape starting from the upper left corner.
This function uses the variable direction to keep track of the direction in which numbers should be filled into the matrix. It starts with direction set to "Down" and updates the direction based on the current position of the matrix and whether the next position is filled or not . That number is then placed at the current location and the row and column variables are updated accordingly.
Repeat this process until every position in the matrix is filled with numbers.
Usage example -
The above is the detailed content of JavaScript program forms coils in matrix form. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
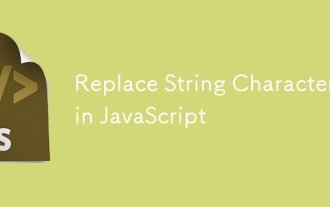
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
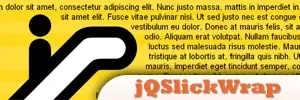
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
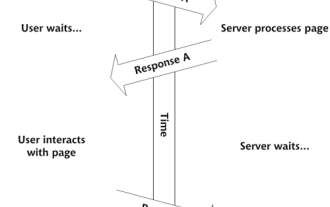
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
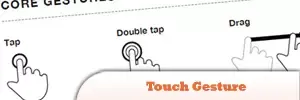
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
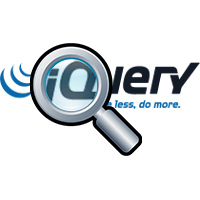
jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
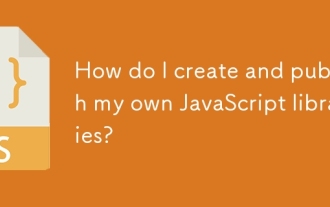
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
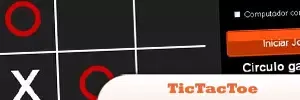
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
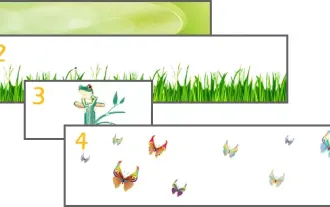
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
